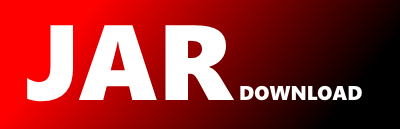
net.derquinse.common.metrics.cache.CacheMetrics Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of derquinse-common-metrics Show documentation
Show all versions of derquinse-common-metrics Show documentation
Coda Hale Metrics Package integration.
/*
* Copyright (C) the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.derquinse.common.metrics.cache;
import static com.google.common.base.Preconditions.checkNotNull;
import static com.yammer.metrics.MetricRegistry.name;
import com.google.common.cache.Cache;
import com.yammer.metrics.Gauge;
import com.yammer.metrics.MetricRegistry;
/**
* Metrics for a Guava {@link Cache}. The cache must record stats for the metrics to be useful.
* @author Andres Rodriguez
*/
public final class CacheMetrics {
/** Cache to instrument. */
private final Cache, ?> cache;
/** Average load penalty. */
private final AverageLoadPenalty averageLoadPenalty;
/** Eviction Count. */
private final EvictionCount evictionCount;
/** Hit Count. */
private final HitCount hitCount;
/** Hit rate. */
private final HitRate hitRate;
/** Load Count. */
private final LoadCount loadCount;
/** Load Exception Count. */
private final LoadExceptionCount loadExceptionCount;
/** Load Exception Rate. */
private final LoadExceptionRate loadExceptionRate;
/** Load Success Count. */
private final LoadSuccessCount loadSuccessCount;
/** Miss Count. */
private final MissCount missCount;
/** Miss rate. */
private final MissRate missRate;
/** Request Count. */
private final RequestCount requestCount;
/** Total load time. */
private final TotalLoadTime totalLoadTime;
/** Creates metrics for a {@link Cache} based on its statistics. */
public static CacheMetrics of(Cache, ?> cache) {
return new CacheMetrics(cache);
}
/** Constructor. */
private CacheMetrics(Cache, ?> cache) {
this.cache = checkNotNull(cache, "The cache to extract metrics from must be provided");
this.averageLoadPenalty = new AverageLoadPenalty();
this.evictionCount = new EvictionCount();
this.hitCount = new HitCount();
this.hitRate = new HitRate();
this.loadCount = new LoadCount();
this.loadExceptionCount = new LoadExceptionCount();
this.loadExceptionRate = new LoadExceptionRate();
this.loadSuccessCount = new LoadSuccessCount();
this.missCount = new MissCount();
this.missRate = new MissRate();
this.requestCount = new RequestCount();
this.totalLoadTime = new TotalLoadTime();
}
/** Registers the cache metrics in a registry. */
public CacheMetrics register(MetricRegistry registry, String baseName) {
registry.register(name(baseName, "averageLoadPenalty"), averageLoadPenalty);
registry.register(name(baseName, "evictionCount"), evictionCount);
registry.register(name(baseName, "hitCount"), hitCount);
registry.register(name(baseName, "hitRate"), hitRate);
registry.register(name(baseName, "loadCount"), loadCount);
registry.register(name(baseName, "loadExceptionCount"), loadExceptionCount);
registry.register(name(baseName, "loadExceptionRate"), loadExceptionRate);
registry.register(name(baseName, "loadSuccessCount"), loadSuccessCount);
registry.register(name(baseName, "missCount"), missCount);
registry.register(name(baseName, "missRate"), missRate);
registry.register(name(baseName, "requestCount"), requestCount);
registry.register(name(baseName, "totalLoadTime"), totalLoadTime);
return this;
}
/** Average load penalty. */
public Gauge getAverageLoadPenalty() {
return averageLoadPenalty;
}
/** Eviction Count. */
public Gauge getEvictionCount() {
return evictionCount;
}
/** Hit Count. */
public Gauge getHitCount() {
return hitCount;
}
/** Hit rate. */
public Gauge getHitRate() {
return hitRate;
}
/** Load Count. */
public Gauge getLoadCount() {
return loadCount;
}
/** Load Exception Count. */
public Gauge getLoadExceptionCount() {
return loadExceptionCount;
}
/** Load Exception Rate. */
public Gauge getLoadExceptionRate() {
return loadExceptionRate;
}
/** Load Success Count. */
public Gauge getLoadSuccessCount() {
return loadSuccessCount;
}
/** Miss Count. */
public Gauge getMissCount() {
return missCount;
}
/** Miss Rate. */
public Gauge getMissRate() {
return missRate;
}
/** Request Count. */
public Gauge getRequestCount() {
return requestCount;
}
/** Total load time. */
public Gauge getTotalLoadTime() {
return totalLoadTime;
}
/** Average load penalty. */
private class AverageLoadPenalty implements Gauge {
@Override
public Double getValue() {
return cache.stats().averageLoadPenalty();
}
}
/** Eviction Count. */
private class EvictionCount implements Gauge {
@Override
public Long getValue() {
return cache.stats().evictionCount();
}
}
/** Hit Count. */
private class HitCount implements Gauge {
@Override
public Long getValue() {
return cache.stats().hitCount();
}
}
/** Hit rate. */
private class HitRate implements Gauge {
@Override
public Double getValue() {
return cache.stats().hitRate();
}
}
/** Load Count. */
private class LoadCount implements Gauge {
@Override
public Long getValue() {
return cache.stats().loadCount();
}
}
/** Load Exception Count. */
private class LoadExceptionCount implements Gauge {
@Override
public Long getValue() {
return cache.stats().loadExceptionCount();
}
}
/** Load Exception Rate. */
private class LoadExceptionRate implements Gauge {
@Override
public Double getValue() {
return cache.stats().loadExceptionRate();
}
}
/** Load Success Count. */
private class LoadSuccessCount implements Gauge {
@Override
public Long getValue() {
return cache.stats().loadSuccessCount();
}
}
/** Miss Count. */
private class MissCount implements Gauge {
@Override
public Long getValue() {
return cache.stats().missCount();
}
}
/** Miss rate. */
private class MissRate implements Gauge {
@Override
public Double getValue() {
return cache.stats().missRate();
}
}
/** Request Count. */
private class RequestCount implements Gauge {
@Override
public Long getValue() {
return cache.stats().requestCount();
}
}
/** Total load time. */
private class TotalLoadTime implements Gauge {
@Override
public Long getValue() {
return cache.stats().totalLoadTime();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy