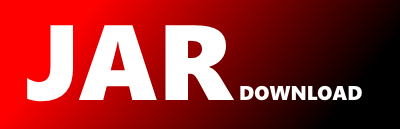
net.digitalid.utility.collections.list.FreezableArrayListSubclass Maven / Gradle / Ivy
The newest version!
package net.digitalid.utility.collections.list;
import java.util.Collection;
import java.util.Comparator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import javax.annotation.Generated;
import javax.annotation.Nonnull;
import net.digitalid.utility.annotations.method.Pure;
import net.digitalid.utility.circumfixes.Circumfix;
import net.digitalid.utility.collections.array.FreezableArray;
import net.digitalid.utility.collections.collection.FreezableCollection;
import net.digitalid.utility.collections.iterator.FreezableIterator;
import net.digitalid.utility.collections.map.FreezableMap;
import net.digitalid.utility.collections.set.FreezableSet;
import net.digitalid.utility.contracts.Ensure;
import net.digitalid.utility.contracts.Require;
import net.digitalid.utility.functional.failable.FailableBinaryOperator;
import net.digitalid.utility.functional.failable.FailableCollector;
import net.digitalid.utility.functional.failable.FailableConsumer;
import net.digitalid.utility.functional.failable.FailablePredicate;
import net.digitalid.utility.functional.failable.FailableUnaryFunction;
import net.digitalid.utility.functional.iterables.FiniteIterable;
import net.digitalid.utility.functional.iterables.InfiniteIterable;
import net.digitalid.utility.functional.iterators.ReadOnlyIterator;
import net.digitalid.utility.functional.iterators.ReadOnlyListIterator;
import net.digitalid.utility.tuples.Pair;
import net.digitalid.utility.validation.annotations.math.NonNegative;
@SuppressWarnings("null")
@Generated(value = "net.digitalid.utility.processor.generator.JavaFileGenerator", date = "2017-03-26T11:54:21.455+0200")
class FreezableArrayListSubclass extends FreezableArrayList {
/* -------------------------------------------------- Constructors -------------------------------------------------- */
FreezableArrayListSubclass() {
super();
validate();
}
FreezableArrayListSubclass(@NonNegative int initialCapacity) {
super(initialCapacity);
validate();
}
FreezableArrayListSubclass(@NonNegative int initialCapacity, @Nonnull Iterable extends E> iterable) {
super(initialCapacity, iterable);
validate();
}
/* -------------------------------------------------- Overridden Methods -------------------------------------------------- */
@Override
public T castTo(Class targetClass) {
T result = super.castTo(targetClass);
Ensure.that(result == this).orThrow("The result has to be but was $.", result);
return result;
}
@Override
public boolean hasSize(int arg0) {
Require.that(arg0 >= 0).orThrow("The arg0 has to be non-negative but was $.", arg0);
boolean result = super.hasSize(arg0);
return result;
}
@Override
public boolean sizeAtMost(int arg0) {
Require.that(arg0 >= 0).orThrow("The arg0 has to be non-negative but was $.", arg0);
boolean result = super.sizeAtMost(arg0);
return result;
}
@Override
public boolean sizeAtLeast(int arg0) {
Require.that(arg0 > 0).orThrow("The arg0 has to be positive but was $.", arg0);
boolean result = super.sizeAtLeast(arg0);
return result;
}
@Override
public FiniteIterable limit(int arg0) {
Require.that(arg0 > 0).orThrow("The arg0 has to be positive but was $.", arg0);
FiniteIterable result = super.limit(arg0);
return result;
}
@Override
public FiniteIterable extract(int arg0, int arg1) {
Require.that(arg0 > 0).orThrow("The arg0 has to be positive but was $.", arg0);
Require.that(arg1 > 0).orThrow("The arg1 has to be positive but was $.", arg1);
FiniteIterable result = super.extract(arg0, arg1);
return result;
}
@Override
public FiniteIterable> zipShortest(FiniteIterable extends TYPE> iterable) {
FiniteIterable> result = super.zipShortest(iterable);
return result;
}
@Override
public InfiniteIterable> zipLongest(InfiniteIterable extends TYPE> iterable) {
InfiniteIterable> result = super.zipLongest(iterable);
return result;
}
@Override
public boolean isSingle() {
boolean result = super.isSingle();
return result;
}
@Override
public boolean isEmptyOrSingle() {
boolean result = super.isEmptyOrSingle();
return result;
}
@Override
public FiniteIterable filter(FailablePredicate super E, ?> predicate) {
FiniteIterable result = super.filter(predicate);
return result;
}
@Override
public FiniteIterable filterNot(FailablePredicate super E, ?> predicate) {
FiniteIterable result = super.filterNot(predicate);
return result;
}
@Override
public FiniteIterable filterNulls() {
FiniteIterable result = super.filterNulls();
return result;
}
@Override
public FiniteIterable map(FailableUnaryFunction super E, ? extends TYPE, ?> function) {
FiniteIterable result = super.map(function);
return result;
}
@Override
public FiniteIterable instanceOf(Class type) {
FiniteIterable result = super.instanceOf(type);
return result;
}
@Override
public FiniteIterable skip(int arg0) {
Require.that(arg0 > 0).orThrow("The arg0 has to be positive but was $.", arg0);
FiniteIterable result = super.skip(arg0);
return result;
}
@Override
public FiniteIterable> zipShortest(InfiniteIterable extends TYPE> iterable) {
FiniteIterable> result = super.zipShortest(iterable);
return result;
}
@Override
public FiniteIterable> zipLongest(FiniteIterable extends TYPE> iterable) {
FiniteIterable> result = super.zipLongest(iterable);
return result;
}
@Override
public FiniteIterable flatten(int arg0) {
Require.that(arg0 > 0).orThrow("The arg0 has to be positive but was $.", arg0);
FiniteIterable result = super.flatten(arg0);
return result;
}
@Override
public FiniteIterable flattenOne() {
FiniteIterable result = super.flattenOne();
return result;
}
@Override
public FiniteIterable flattenAll() {
FiniteIterable result = super.flattenAll();
return result;
}
@Override
public boolean equals(FiniteIterable> iterable) {
boolean result = super.equals(iterable);
return result;
}
@Override
public E getFirst(E arg0) {
E result = super.getFirst(arg0);
return result;
}
@Override
public E getFirstOrNull() {
E result = super.getFirstOrNull();
return result;
}
@Override
public E getFirst() {
E result = super.getFirst();
return result;
}
@Override
public E getLast(E arg0) {
E result = super.getLast(arg0);
return result;
}
@Override
public E getLastOrNull() {
E result = super.getLastOrNull();
return result;
}
@Override
public E getLast() {
E result = super.getLast();
return result;
}
@Override
public int count(Object arg0) {
int result = super.count(arg0);
Ensure.that(result >= 0).orThrow("The result has to be non-negative but was $.", result);
return result;
}
@Override
public boolean containsAll(FiniteIterable> iterable) {
boolean result = super.containsAll(iterable);
return result;
}
@Override
public boolean containsNull() {
boolean result = super.containsNull();
return result;
}
@Override
public boolean containsDuplicates() {
boolean result = super.containsDuplicates();
return result;
}
@Override
public FiniteIterable distinct() {
FiniteIterable result = super.distinct();
return result;
}
@Override
public FiniteIterable doForEach(FailableConsumer super E, ? extends EXCEPTION> arg0) throws EXCEPTION {
FiniteIterable result = super.doForEach(arg0);
Ensure.that(result == this).orThrow("The result has to be but was $.", result);
return result;
}
@Override
public FiniteIterable intersect(FiniteIterable super E> iterable) {
FiniteIterable result = super.intersect(iterable);
return result;
}
@Override
public FiniteIterable exclude(FiniteIterable super E> iterable) {
FiniteIterable result = super.exclude(iterable);
return result;
}
@Override
public FiniteIterable combine(FiniteIterable extends E> iterable) {
FiniteIterable result = super.combine(iterable);
return result;
}
@Override
public InfiniteIterable combine(InfiniteIterable extends E> iterable) {
InfiniteIterable result = super.combine(iterable);
return result;
}
@Override
public InfiniteIterable repeated() {
InfiniteIterable result = super.repeated();
return result;
}
@Override
public E findFirst(FailablePredicate super E, ? extends EXCEPTION> arg0, E arg1) throws EXCEPTION {
E result = super.findFirst(arg0, arg1);
return result;
}
@Override
public E findFirst(FailablePredicate super E, ? extends EXCEPTION> predicate) throws EXCEPTION {
E result = super.findFirst(predicate);
return result;
}
@Override
public E findLast(FailablePredicate super E, ? extends EXCEPTION> arg0, E arg1) throws EXCEPTION {
E result = super.findLast(arg0, arg1);
return result;
}
@Override
public E findLast(FailablePredicate super E, ? extends EXCEPTION> predicate) throws EXCEPTION {
E result = super.findLast(predicate);
return result;
}
@Override
public E findUnique(FailablePredicate super E, ? extends EXCEPTION> predicate) throws EXCEPTION {
E result = super.findUnique(predicate);
return result;
}
@Override
public boolean matchAny(FailablePredicate super E, ? extends EXCEPTION> predicate) throws EXCEPTION {
boolean result = super.matchAny(predicate);
return result;
}
@Override
public boolean matchAll(FailablePredicate super E, ? extends EXCEPTION> predicate) throws EXCEPTION {
boolean result = super.matchAll(predicate);
return result;
}
@Override
public boolean matchNone(FailablePredicate super E, ? extends EXCEPTION> predicate) throws EXCEPTION {
boolean result = super.matchNone(predicate);
return result;
}
@Override
public E reduce(FailableBinaryOperator arg0, E arg1) throws EXCEPTION {
E result = super.reduce(arg0, arg1);
return result;
}
@Override
public E reduce(FailableBinaryOperator operator) throws EXCEPTION {
E result = super.reduce(operator);
return result;
}
@Override
public RESULT collect(FailableCollector super E, ? extends RESULT, ? extends COLLECT_EXCEPTION, ? extends RESULT_EXCEPTION> arg0) throws COLLECT_EXCEPTION, RESULT_EXCEPTION {
RESULT result = super.collect(arg0);
return result;
}
@Override
public boolean isOrdered(boolean strictly, boolean ascending) {
boolean result = super.isOrdered(strictly, ascending);
return result;
}
@Override
public boolean isAscending() {
boolean result = super.isAscending();
return result;
}
@Override
public boolean isStrictlyAscending() {
boolean result = super.isStrictlyAscending();
return result;
}
@Override
public boolean isDescending() {
boolean result = super.isDescending();
return result;
}
@Override
public boolean isStrictlyDescending() {
boolean result = super.isStrictlyDescending();
return result;
}
@Override
public FiniteIterable sorted(Comparator super E> comparator) {
FiniteIterable result = super.sorted(comparator);
return result;
}
@Override
public FiniteIterable sorted() {
FiniteIterable result = super.sorted();
return result;
}
@Override
public FiniteIterable reversed() {
FiniteIterable result = super.reversed();
return result;
}
@Override
public E min(Comparator super E> arg0, E arg1) {
E result = super.min(arg0, arg1);
return result;
}
@Override
public E min(Comparator super E> comparator) {
E result = super.min(comparator);
return result;
}
@Override
public E min(E arg0) {
E result = super.min(arg0);
return result;
}
@Override
public E min() {
E result = super.min();
return result;
}
@Override
public E max(Comparator super E> arg0, E arg1) {
E result = super.max(arg0, arg1);
return result;
}
@Override
public E max(Comparator super E> comparator) {
E result = super.max(comparator);
return result;
}
@Override
public E max(E arg0) {
E result = super.max(arg0);
return result;
}
@Override
public E max() {
E result = super.max();
return result;
}
@Override
public long sumAsLong() {
long result = super.sumAsLong();
return result;
}
@Override
public double sumAsDouble() {
double result = super.sumAsDouble();
return result;
}
@Override
public double average() {
double result = super.average();
return result;
}
@Override
public String join(CharSequence prefix, CharSequence suffix, CharSequence empty, CharSequence delimiter) {
String result = super.join(prefix, suffix, empty, delimiter);
return result;
}
@Override
public String join(CharSequence prefix, CharSequence suffix, CharSequence empty) {
String result = super.join(prefix, suffix, empty);
return result;
}
@Override
public String join(CharSequence prefix, CharSequence suffix) {
String result = super.join(prefix, suffix);
return result;
}
@Override
public String join(Circumfix fixes, CharSequence empty, CharSequence delimiter) {
String result = super.join(fixes, empty, delimiter);
return result;
}
@Override
public String join(Circumfix fixes, CharSequence empty) {
String result = super.join(fixes, empty);
return result;
}
@Override
public String join(Circumfix fixes) {
String result = super.join(fixes);
return result;
}
@Override
public String join(CharSequence delimiter) {
String result = super.join(delimiter);
return result;
}
@Override
public String join() {
String result = super.join();
return result;
}
@Override
public E[] toGenericArray() {
E[] result = super.toGenericArray();
return result;
}
@Override
public List toList() {
List result = super.toList();
return result;
}
@Override
public Set toSet() {
Set result = super.toSet();
return result;
}
@Override
public Map toMap(FailableUnaryFunction super E, ? extends KEY, ? extends EXCEPTION> function) throws EXCEPTION {
Map result = super.toMap(function);
return result;
}
@Override
public Map> groupBy(FailableUnaryFunction super E, ? extends KEY, ? extends EXCEPTION> function) throws EXCEPTION {
Map> result = super.groupBy(function);
return result;
}
@Override
public FiniteIterable evaluate() throws EXCEPTION {
FiniteIterable result = super.evaluate();
return result;
}
@Override
public int size(int arg0) {
Require.that(arg0 > 0).orThrow("The arg0 has to be positive but was $.", arg0);
int result = super.size(arg0);
Ensure.that(result >= 0).orThrow("The result has to be non-negative but was $.", result);
return result;
}
@Override
public FreezableArray toFreezableArray() {
FreezableArray result = super.toFreezableArray();
Ensure.that(result != null).orThrow("The result may not be null.", result);
Ensure.that(result == null || !result.isFrozen()).orThrow("The result has to be null or may not be frozen.", result);
return result;
}
@Override
public FreezableList toFreezableList() {
FreezableList result = super.toFreezableList();
Ensure.that(result != null).orThrow("The result may not be null.", result);
Ensure.that(result == null || !result.isFrozen()).orThrow("The result has to be null or may not be frozen.", result);
return result;
}
@Override
public FreezableSet toFreezableSet() {
FreezableSet result = super.toFreezableSet();
Ensure.that(result != null).orThrow("The result may not be null.", result);
Ensure.that(result == null || !result.isFrozen()).orThrow("The result has to be null or may not be frozen.", result);
return result;
}
@Override
public FreezableMap toFreezableMap(FailableUnaryFunction super E, ? extends KEY, ? extends EXCEPTION> function) throws EXCEPTION {
Require.that(function != null).orThrow("The function may not be null.", function);
FreezableMap result = super.toFreezableMap(function);
Ensure.that(result != null).orThrow("The result may not be null.", result);
Ensure.that(result == null || !result.isFrozen()).orThrow("The result has to be null or may not be frozen.", result);
return result;
}
@Override
public boolean containsAll(FreezableCollection> collection) {
Require.that(collection != null).orThrow("The collection may not be null.", collection);
boolean result = super.containsAll(collection);
return result;
}
@Override
public boolean addAll(FiniteIterable extends E> iterable) {
Require.that(iterable != null).orThrow("The iterable may not be null.", iterable);
Require.that(!isFrozen()).orThrow("The method 'addAll' may only be called on non-frozen objects.");
boolean result = super.addAll(iterable);
return result;
}
@Override
public boolean addAll(FreezableCollection extends E> collection) {
Require.that(collection != null).orThrow("The collection may not be null.", collection);
Require.that(!isFrozen()).orThrow("The method 'addAll' may only be called on non-frozen objects.");
boolean result = super.addAll(collection);
return result;
}
@Override
public boolean removeAll(FiniteIterable> iterable) {
Require.that(iterable != null).orThrow("The iterable may not be null.", iterable);
Require.that(!isFrozen()).orThrow("The method 'removeAll' may only be called on non-frozen objects.");
boolean result = super.removeAll(iterable);
return result;
}
@Override
public boolean removeAll(FreezableCollection> collection) {
Require.that(collection != null).orThrow("The collection may not be null.", collection);
Require.that(!isFrozen()).orThrow("The method 'removeAll' may only be called on non-frozen objects.");
boolean result = super.removeAll(collection);
return result;
}
@Override
public boolean retainAll(FiniteIterable> iterable) {
Require.that(iterable != null).orThrow("The iterable may not be null.", iterable);
Require.that(!isFrozen()).orThrow("The method 'retainAll' may only be called on non-frozen objects.");
boolean result = super.retainAll(iterable);
return result;
}
@Override
public boolean retainAll(FreezableCollection> collection) {
Require.that(collection != null).orThrow("The collection may not be null.", collection);
Require.that(!isFrozen()).orThrow("The method 'retainAll' may only be called on non-frozen objects.");
boolean result = super.retainAll(collection);
return result;
}
@Override
public boolean isFrozen() {
boolean result = super.isFrozen();
return result;
}
@Override
public ReadOnlyList freeze() {
Require.that(!isFrozen()).orThrow("The method 'freeze' may only be called on non-frozen objects.");
ReadOnlyList result = super.freeze();
Ensure.that(result != null).orThrow("The result may not be null.", result);
Ensure.that(result == null || result.isFrozen()).orThrow("The result has to be null or frozen.", result);
return result;
}
@Override
public ReadOnlyIterator iterator() {
ReadOnlyIterator result = super.iterator();
Ensure.that(result != null).orThrow("The result may not be null.", result);
return result;
}
@Override
public FreezableIterator freezableIterator() {
FreezableIterator result = super.freezableIterator();
Ensure.that(result != null).orThrow("The result may not be null.", result);
return result;
}
@Override
public E get(int index) {
Require.that(index >= 0 && index < size()).orThrow("The index may not be negative or greater than or equal to the size of this collection but was $.", index);
E result = super.get(index);
return result;
}
@Override
public ReadOnlyListIterator listIterator() {
ReadOnlyListIterator result = super.listIterator();
Ensure.that(result != null).orThrow("The result may not be null.", result);
return result;
}
@Override
public ReadOnlyListIterator listIterator(int index) {
Require.that(index >= 0 && index <= size()).orThrow("The index may not be negative or greater than the size of this collection but was $.", index);
ReadOnlyListIterator result = super.listIterator(index);
Ensure.that(result != null).orThrow("The result may not be null.", result);
return result;
}
@Override
public FreezableList subList(int fromIndex, int toIndex) {
Require.that(fromIndex >= 0 && fromIndex < size()).orThrow("The from index may not be negative or greater than or equal to the size of this collection but was $.", fromIndex);
Require.that(toIndex >= 0 && toIndex <= size()).orThrow("The to index may not be negative or greater than the size of this collection but was $.", toIndex);
FreezableList result = super.subList(fromIndex, toIndex);
Ensure.that(result != null).orThrow("The result may not be null.", result);
return result;
}
@Override
public boolean add(E element) {
Require.that(!isFrozen()).orThrow("The method 'add' may only be called on non-frozen objects.");
boolean result = super.add(element);
return result;
}
@Override
public void add(int index, E element) {
Require.that(index >= 0 && index <= size()).orThrow("The index may not be negative or greater than the size of this collection but was $.", index);
Require.that(!isFrozen()).orThrow("The method 'add' may only be called on non-frozen objects.");
super.add(index, element);
}
@Override
public boolean addAll(Collection extends E> collection) {
Require.that(collection != null).orThrow("The collection may not be null.", collection);
Require.that(!isFrozen()).orThrow("The method 'addAll' may only be called on non-frozen objects.");
boolean result = super.addAll(collection);
return result;
}
@Override
public boolean addAll(int index, Collection extends E> collection) {
Require.that(index >= 0 && index <= size()).orThrow("The index may not be negative or greater than the size of this collection but was $.", index);
Require.that(collection != null).orThrow("The collection may not be null.", collection);
Require.that(!isFrozen()).orThrow("The method 'addAll' may only be called on non-frozen objects.");
boolean result = super.addAll(index, collection);
return result;
}
@Override
public E remove(int index) {
Require.that(index >= 0 && index < size()).orThrow("The index may not be negative or greater than or equal to the size of this collection but was $.", index);
Require.that(!isFrozen()).orThrow("The method 'remove' may only be called on non-frozen objects.");
E result = super.remove(index);
return result;
}
@Override
public boolean remove(Object object) {
Require.that(!isFrozen()).orThrow("The method 'remove' may only be called on non-frozen objects.");
boolean result = super.remove(object);
return result;
}
@Override
protected void removeRange(int fromIndex, int toIndex) {
Require.that(fromIndex >= 0 && fromIndex < size()).orThrow("The from index may not be negative or greater than or equal to the size of this collection but was $.", fromIndex);
Require.that(toIndex >= 0 && toIndex <= size()).orThrow("The to index may not be negative or greater than the size of this collection but was $.", toIndex);
Require.that(!isFrozen()).orThrow("The method 'removeRange' may only be called on non-frozen objects.");
super.removeRange(fromIndex, toIndex);
}
@Override
public boolean removeAll(Collection> collection) {
Require.that(collection != null).orThrow("The collection may not be null.", collection);
Require.that(!isFrozen()).orThrow("The method 'removeAll' may only be called on non-frozen objects.");
boolean result = super.removeAll(collection);
return result;
}
@Override
public boolean retainAll(Collection> collection) {
Require.that(collection != null).orThrow("The collection may not be null.", collection);
Require.that(!isFrozen()).orThrow("The method 'retainAll' may only be called on non-frozen objects.");
boolean result = super.retainAll(collection);
return result;
}
@Override
public void clear() {
Require.that(!isFrozen()).orThrow("The method 'clear' may only be called on non-frozen objects.");
super.clear();
}
@Override
public E set(int index, E element) {
Require.that(index >= 0 && index < size()).orThrow("The index may not be negative or greater than or equal to the size of this collection but was $.", index);
Require.that(!isFrozen()).orThrow("The method 'set' may only be called on non-frozen objects.");
E result = super.set(index, element);
return result;
}
@Override
public void ensureCapacity(int minCapacity) {
Require.that(minCapacity >= 0).orThrow("The min capacity has to be non-negative but was $.", minCapacity);
Require.that(!isFrozen()).orThrow("The method 'ensureCapacity' may only be called on non-frozen objects.");
super.ensureCapacity(minCapacity);
}
@Override
public void trimToSize() {
Require.that(!isFrozen()).orThrow("The method 'trimToSize' may only be called on non-frozen objects.");
super.trimToSize();
}
/* -------------------------------------------------- Implement Methods -------------------------------------------------- */
/* -------------------------------------------------- Generated Methods -------------------------------------------------- */
@Pure
@Override
public void validate() {
super.validate();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy