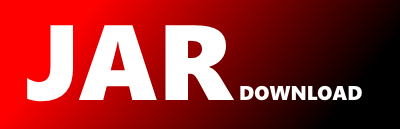
net.digitalid.utility.collections.map.FreezableLinkedHashMapSubclass Maven / Gradle / Ivy
The newest version!
package net.digitalid.utility.collections.map;
import java.util.Collection;
import java.util.Map;
import javax.annotation.Generated;
import javax.annotation.Nonnull;
import net.digitalid.utility.annotations.method.Pure;
import net.digitalid.utility.collections.collection.FreezableCollection;
import net.digitalid.utility.collections.set.FreezableSet;
import net.digitalid.utility.contracts.Ensure;
import net.digitalid.utility.contracts.Require;
import net.digitalid.utility.functional.iterables.FiniteIterable;
import net.digitalid.utility.immutable.entry.ReadOnlyEntrySet;
import net.digitalid.utility.validation.annotations.math.NonNegative;
import net.digitalid.utility.validation.annotations.math.Positive;
@SuppressWarnings("null")
@Generated(value = "net.digitalid.utility.processor.generator.JavaFileGenerator", date = "2017-03-26T11:54:21.271+0200")
class FreezableLinkedHashMapSubclass extends FreezableLinkedHashMap {
/* -------------------------------------------------- Constructors -------------------------------------------------- */
FreezableLinkedHashMapSubclass(@NonNegative int initialCapacity, @Positive float loadFactor, boolean accessOrder) {
super(initialCapacity, loadFactor, accessOrder);
validate();
}
FreezableLinkedHashMapSubclass(@Nonnull Map extends K, ? extends V> map) {
super(map);
validate();
}
/* -------------------------------------------------- Overridden Methods -------------------------------------------------- */
@Override
public T castTo(Class targetClass) {
T result = super.castTo(targetClass);
Ensure.that(result == this).orThrow("The result has to be but was $.", result);
return result;
}
@Override
public boolean isSingle() {
boolean result = super.isSingle();
return result;
}
@Override
public boolean isEmptyOrSingle() {
boolean result = super.isEmptyOrSingle();
return result;
}
@Override
public void putAll(ReadOnlyMap extends K, ? extends V> map) {
Require.that(map != null).orThrow("The map may not be null.", map);
Require.that(!isFrozen()).orThrow("The method 'putAll' may only be called on non-frozen objects.");
super.putAll(map);
}
@Override
public void removeAll(FiniteIterable> keys) {
Require.that(keys != null).orThrow("The keys may not be null.", keys);
Require.that(!isFrozen()).orThrow("The method 'removeAll' may only be called on non-frozen objects.");
super.removeAll(keys);
}
@Override
public void removeAll(Collection> keys) {
Require.that(keys != null).orThrow("The keys may not be null.", keys);
Require.that(!isFrozen()).orThrow("The method 'removeAll' may only be called on non-frozen objects.");
super.removeAll(keys);
}
@Override
public void removeAll(FreezableCollection> keys) {
Require.that(keys != null).orThrow("The keys may not be null.", keys);
Require.that(!isFrozen()).orThrow("The method 'removeAll' may only be called on non-frozen objects.");
super.removeAll(keys);
}
@Override
public void removeAll(ReadOnlyMap extends K, ? extends V> map) {
Require.that(map != null).orThrow("The map may not be null.", map);
Require.that(!isFrozen()).orThrow("The method 'removeAll' may only be called on non-frozen objects.");
super.removeAll(map);
}
@Override
public V putIfAbsentOrNullElseReturnPresent(K key, V value) {
Require.that(value != null).orThrow("The value may not be null.", value);
Require.that(!isFrozen()).orThrow("The method 'putIfAbsentOrNullElseReturnPresent' may only be called on non-frozen objects.");
V result = super.putIfAbsentOrNullElseReturnPresent(key, value);
Ensure.that(result != null).orThrow("The result may not be null.", result);
return result;
}
@Override
public boolean isEmpty() {
boolean result = super.isEmpty();
return result;
}
@Override
public boolean isFrozen() {
boolean result = super.isFrozen();
return result;
}
@Override
public ReadOnlyMap freeze() {
Require.that(!isFrozen()).orThrow("The method 'freeze' may only be called on non-frozen objects.");
ReadOnlyMap result = super.freeze();
Ensure.that(result == this).orThrow("The result has to be but was $.", result);
Ensure.that(result != null).orThrow("The result may not be null.", result);
Ensure.that(result == null || result.isFrozen()).orThrow("The result has to be null or frozen.", result);
return result;
}
@Override
public FreezableSet keySet() {
FreezableSet result = super.keySet();
Ensure.that(result != null).orThrow("The result may not be null.", result);
return result;
}
@Override
public FreezableCollection values() {
FreezableCollection result = super.values();
Ensure.that(result != null).orThrow("The result may not be null.", result);
return result;
}
@Override
public ReadOnlyEntrySet entrySet() {
ReadOnlyEntrySet result = super.entrySet();
Ensure.that(result != null).orThrow("The result may not be null.", result);
return result;
}
@Override
public V put(K key, V value) {
Require.that(!isFrozen()).orThrow("The method 'put' may only be called on non-frozen objects.");
V result = super.put(key, value);
return result;
}
@Override
public void putAll(Map extends K, ? extends V> map) {
Require.that(map != null).orThrow("The map may not be null.", map);
Require.that(!isFrozen()).orThrow("The method 'putAll' may only be called on non-frozen objects.");
super.putAll(map);
}
@Override
public V remove(Object object) {
Require.that(!isFrozen()).orThrow("The method 'remove' may only be called on non-frozen objects.");
V result = super.remove(object);
return result;
}
@Override
public void clear() {
Require.that(!isFrozen()).orThrow("The method 'clear' may only be called on non-frozen objects.");
super.clear();
}
/* -------------------------------------------------- Implement Methods -------------------------------------------------- */
/* -------------------------------------------------- Generated Methods -------------------------------------------------- */
@Pure
@Override
public void validate() {
super.validate();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy