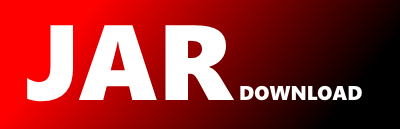
net.disy.commons.xml.bind.dwr.JAXBElementConverter Maven / Gradle / Ivy
/**
* Legato is a configurable, lightweight web mapping client that can be
* easily embedded into web pages and portals, CMS and individual web
* applications. Legato is implemented in JavaScript and based on the
* popular open source library OpenLayers.
*
* Copyright (C) 2010 disy Informationssysteme GmbH, http://www.disy.net
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package net.disy.commons.xml.bind.dwr;
import java.util.Collections;
import java.util.Map;
import java.util.TreeMap;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.JAXBException;
import javax.xml.namespace.QName;
import net.disy.commons.dwr.convert.AbstractConverter;
import org.apache.commons.lang.ObjectUtils;
import org.directwebremoting.dwrp.ObjectOutboundVariable;
import org.directwebremoting.extend.InboundContext;
import org.directwebremoting.extend.InboundVariable;
import org.directwebremoting.extend.MarshallException;
import org.directwebremoting.extend.OutboundContext;
import org.directwebremoting.extend.OutboundVariable;
import com.sun.xml.bind.api.JAXBRIContext;
import com.sun.xml.bind.v2.ContextFactory;
import com.sun.xml.bind.v2.model.runtime.RuntimeElementInfo;
public class JAXBElementConverter extends AbstractConverter {
private JAXBRIContext context;
public JAXBRIContext getJAXBRIContext() throws MarshallException {
if (context == null) {
try {
this.context = (JAXBRIContext) ContextFactory.createContext(getContextPath(), Thread
.currentThread()
.getContextClassLoader(), Collections. emptyMap());
}
catch (JAXBException jaxbex) {
throw new MarshallException(JAXBElement.class, jaxbex);
}
}
return context;
}
@Override
protected Object convertInbound(
@SuppressWarnings("unchecked") Class paramType,
InboundVariable data,
Map tokens,
InboundContext inctx,
String value) throws MarshallException {
QName name = getValue("name", QName.class, data, tokens, inctx); //$NON-NLS-1$
for (RuntimeElementInfo elementInfo : getJAXBRIContext()
.getRuntimeTypeInfoSet()
.getAllElements()) {
if (ObjectUtils.equals(name, elementInfo.getElementName())) {
@SuppressWarnings("unchecked")
final Class
© 2015 - 2024 Weber Informatics LLC | Privacy Policy