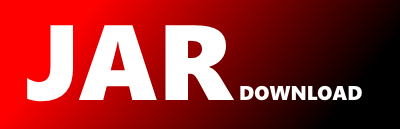
net.disy.ogc.wps.v_1_0_0.DefaultGetCapabilitiesService Maven / Gradle / Ivy
/**
* Legato is a configurable, lightweight web mapping client that can be
* easily embedded into web pages and portals, CMS and individual web
* applications. Legato is implemented in JavaScript and based on the
* popular open source library OpenLayers.
*
* Copyright (C) 2010 disy Informationssysteme GmbH, http://www.disy.net
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package net.disy.ogc.wps.v_1_0_0;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Locale;
import net.opengis.ows.v_1_1_0.MetadataType;
import net.opengis.ows.v_1_1_0.OperationsMetadata;
import net.opengis.ows.v_1_1_0.ServiceIdentification;
import net.opengis.ows.v_1_1_0.ServiceProvider;
import net.opengis.wps.v_1_0_0.GetCapabilities;
import net.opengis.wps.v_1_0_0.Languages;
import net.opengis.wps.v_1_0_0.LanguagesType;
import net.opengis.wps.v_1_0_0.ProcessBriefType;
import net.opengis.wps.v_1_0_0.ProcessDescriptionType;
import net.opengis.wps.v_1_0_0.ProcessOfferings;
import net.opengis.wps.v_1_0_0.WPSCapabilitiesType;
public class DefaultGetCapabilitiesService implements GetCapabilitiesService {
private WpsProcessRegistry wpsProcessRegistry;
public WpsProcessRegistry getWpsProcessRegistry() {
return wpsProcessRegistry;
}
public void setWpsProcessRegistry(WpsProcessRegistry wpsProcessRegistry) {
this.wpsProcessRegistry = wpsProcessRegistry;
}
private ServiceIdentification serviceIdentification;
public ServiceIdentification getServiceIdentification() {
return serviceIdentification;
}
public void setServiceIdentification(ServiceIdentification serviceIdentification) {
this.serviceIdentification = serviceIdentification;
}
private ServiceProvider serviceProvider;
public ServiceProvider getServiceProvider() {
return serviceProvider;
}
public void setServiceProvider(ServiceProvider serviceProvider) {
this.serviceProvider = serviceProvider;
}
private Locale defaultLanguage = Locale.ENGLISH;
public Locale getDefaultLanguage() {
return defaultLanguage;
}
public void setDefaultLanguage(Locale defaultLanguage) {
this.defaultLanguage = defaultLanguage;
}
private Collection supportedLanguages = Collections.singletonList(Locale.ENGLISH);
public Collection getSupportedLanguages() {
return supportedLanguages;
}
public void setSupportedLanguages(Collection supportedLanguages) {
this.supportedLanguages = supportedLanguages;
}
@Override
public WPSCapabilitiesType execute(GetCapabilities input) {
final WPSCapabilitiesType wpsCapabilities = new WPSCapabilitiesType();
wpsCapabilities.setVersion("1.0.0"); //$NON-NLS-1$
wpsCapabilities.setService("WPS"); //$NON-NLS-1$
final ServiceIdentification currentServiceIdentification = getServiceIdentification();
if (currentServiceIdentification != null) {
wpsCapabilities.setServiceIdentification(currentServiceIdentification);
}
final ServiceProvider currentServiceProvider = getServiceProvider();
if (currentServiceProvider != null) {
wpsCapabilities.setServiceProvider(currentServiceProvider);
}
wpsCapabilities.setOperationsMetadata(getOperationsMetadata());
wpsCapabilities.setProcessOfferings(getProcessOfferings());
wpsCapabilities.setLanguages(getLanguages());
if (getDefaultLanguage() != null) {
wpsCapabilities.setLang(getDefaultLanguage().toString());
}
return wpsCapabilities;
}
private OperationsMetadata operationsMetadata;
public OperationsMetadata getOperationsMetadata() {
return operationsMetadata;
}
public void setOperationsMetadata(OperationsMetadata operationsMetadata) {
this.operationsMetadata = operationsMetadata;
}
private ProcessOfferings getProcessOfferings() {
final ProcessOfferings processOfferings = new ProcessOfferings();
for (WpsProcess wpsProcess : getWpsProcessRegistry().getProcesses()) {
final ProcessDescriptionType processDescription = wpsProcess.getProcessDescription();
final ProcessBriefType briefProcessDescription = new ProcessBriefType();
if (processDescription.getIdentifier() != null) {
briefProcessDescription.setIdentifier(processDescription.getIdentifier());
}
if (processDescription.getTitle() != null) {
briefProcessDescription.setTitle(processDescription.getTitle());
}
if (processDescription.getAbstract() != null) {
briefProcessDescription.setAbstract(processDescription.getAbstract());
}
final List metadata = processDescription.getMetadata();
if (!metadata.isEmpty()) {
briefProcessDescription.getMetadata().addAll(metadata);
}
if (!processDescription.getProfile().isEmpty()) {
briefProcessDescription.getProfile().addAll(processDescription.getProfile());
}
if (processDescription.getWSDL() != null) {
briefProcessDescription.setWSDL(processDescription.getWSDL());
}
if (processDescription.getProcessVersion() != null) {
briefProcessDescription.setProcessVersion(processDescription.getProcessVersion());
}
processOfferings.getProcess().add(briefProcessDescription);
}
return processOfferings;
}
private Languages getLanguages() {
final Languages languages = new Languages();
if (getDefaultLanguage() != null) {
Languages.Default newDefaultLanguage = new Languages.Default();
newDefaultLanguage.setLanguage(getDefaultLanguage().toString());
languages.setDefault(newDefaultLanguage);
}
if (getSupportedLanguages() != null) {
final LanguagesType newSupportedLanguages = new LanguagesType();
for (Locale locale : getSupportedLanguages()) {
newSupportedLanguages.getLanguage().add(locale.toString());
}
languages.setSupported(newSupportedLanguages);
}
return languages;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy