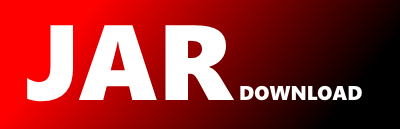
net.disy.commons.xml.namespace.dwr.QNameConverter Maven / Gradle / Ivy
/**
* Legato is a configurable, lightweight web mapping client that can be
* easily embedded into web pages and portals, CMS and individual web
* applications. Legato is implemented in JavaScript and based on the
* popular open source library OpenLayers.
*
* Copyright (C) 2010 disy Informationssysteme GmbH, http://www.disy.net
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package net.disy.commons.xml.namespace.dwr;
import java.util.Map;
import java.util.TreeMap;
import javax.xml.namespace.QName;
import net.disy.commons.dwr.convert.AbstractConverter;
import org.apache.commons.lang.StringUtils;
import org.directwebremoting.dwrp.ObjectOutboundVariable;
import org.directwebremoting.extend.InboundContext;
import org.directwebremoting.extend.InboundVariable;
import org.directwebremoting.extend.MarshallException;
import org.directwebremoting.extend.OutboundContext;
import org.directwebremoting.extend.OutboundVariable;
public class QNameConverter extends AbstractConverter {
@Override
protected Object convertInbound(
@SuppressWarnings("unchecked") Class paramType,
InboundVariable data,
Map tokens,
InboundContext inctx,
String value) throws MarshallException {
final String namespaceURI = getValue("namespaceURI", String.class, data, tokens, inctx); //$NON-NLS-1$
final String localPart = getValue("localPart", String.class, data, tokens, inctx); //$NON-NLS-1$
final String prefix = getValue("prefix", String.class, data, tokens, inctx); //$NON-NLS-1$
final QName result;
if (!StringUtils.isEmpty(namespaceURI) && !StringUtils.isEmpty(prefix)) {
result = new QName(namespaceURI, localPart, prefix);
}
else if (!StringUtils.isEmpty(namespaceURI)) {
result = new QName(namespaceURI, localPart);
}
else {
result = new QName(localPart);
}
return result;
}
@Override
public OutboundVariable convertOutbound(Object data, OutboundContext outctx)
throws MarshallException {
final QName name = (QName) data;
// // Where we collect out converted children
final Map ovs = new TreeMap();
// We need to do this before collecing the children to save recurrsion
final ObjectOutboundVariable ov = new ObjectOutboundVariable(outctx);
outctx.put(data, ov);
if (!StringUtils.isEmpty(name.getNamespaceURI())) {
ovs.put("namespaceURI", getConverterManager() //$NON-NLS-1$
.convertOutbound(name.getNamespaceURI(), outctx));
}
ovs.put("localPart", getConverterManager().convertOutbound(name.getLocalPart(), outctx)); //$NON-NLS-1$
if (!StringUtils.isEmpty(name.getPrefix())) {
ovs.put("prefix", getConverterManager().convertOutbound(name.getPrefix(), outctx)); //$NON-NLS-1$
}
ov.init(ovs, null);
return ov;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy