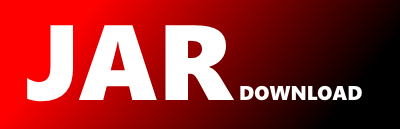
net.disy.commons.httpclient.methods.MarshallingRequestEntity Maven / Gradle / Ivy
The newest version!
/**
* Legato is a configurable, lightweight web mapping client that can be
* easily embedded into web pages and portals, CMS and individual web
* applications. Legato is implemented in JavaScript and based on the
* popular open source library OpenLayers.
*
* Copyright (C) 2010 disy Informationssysteme GmbH, http://www.disy.net
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package net.disy.commons.httpclient.methods;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
import java.io.UnsupportedEncodingException;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Marshaller;
import javax.xml.transform.stream.StreamResult;
import org.apache.commons.lang.Validate;
import org.apache.http.Header;
import org.apache.http.HttpEntity;
import org.apache.http.entity.AbstractHttpEntity;
import org.apache.http.message.BasicHeader;
/*
* Implementation of the {@link RequestEntity} which marshalls its content.
*/
public class MarshallingRequestEntity extends AbstractHttpEntity implements HttpEntity {
/**
* Marshaller.
*/
private final Marshaller marshaller;
/**
* Returns the marshaller to be used for marshalling.
*
* @return Marshaller.
*/
public Marshaller getMarshaller() {
return marshaller;
}
/**
* Object to be marshalled.
*/
private final Object object;
/**
* Returns object to be marshalled.
*
* @return Object to be marshalled.
*/
public Object getObject() {
return object;
}
/**
* Content type.
*/
private final String contentType;
/**
* Returns content type of the request entity.
*
* @return Content type.
*/
public Header getContentType() {
Header header = new BasicHeader("Content-type", contentType);
return header;
}
/**
* Charset used for data encoding.
*/
private final String charset;
/**
* Returns charset used for data encoding.
*
* @return Charset used for data encoding.
*/
public String getCharset() {
return charset;
}
/**
* Marshalled content.
*/
private final byte[] bytes;
/**
* Constructs a marshalling request entity. This constructor simply calls
* {@link #MarshallingRequestEntity(Marshaller, Object, String, String)}
* with application/xml
as content type and
* UTF-8
as charset.
*
* @param marshaller
* marshaller.
* @param object
* object to be marshalled.
* @throws Exception
* In case of marshalling or other problems.
*/
public MarshallingRequestEntity(Marshaller marshaller, Object object) throws JAXBException {
this(marshaller, object, "application/xml", "UTF-8"); //$NON-NLS-1$ //$NON-NLS-2$
}
/**
* Constructs a marshalling entity.
*
* @param marshaller
* marshaller.
* @param object
* object.
* @param contentType
* content type.
* @param charset
* charset.
* @throws Exception
* In case of marshalling or other problems.
*/
public MarshallingRequestEntity(
Marshaller marshaller,
Object object,
String contentType,
String charset) throws JAXBException {
Validate.notNull(marshaller);
Validate.notNull(object);
Validate.notNull(contentType);
Validate.notNull(charset);
this.object = object;
this.marshaller = marshaller;
this.contentType = contentType;
this.charset = charset;
this.bytes = createBytes();
}
public long getContentLength() {
return bytes.length;
}
public boolean isRepeatable() {
return false;
}
/**
* Marshalls the object into the byte array.
*
* @return Marshalled data.
* @throws Exception
* In case of marshalling problems.
*/
protected byte[] createBytes() throws JAXBException {
final ByteArrayOutputStream out = new ByteArrayOutputStream();
try {
final OutputStreamWriter writer = new OutputStreamWriter(out, getCharset());
final StreamResult result = new StreamResult(writer);
getMarshaller().marshal(getObject(), result);
// final int length = out.getCount();
// final byte[] bytes = new byte[length];
// System.arraycopy(out.getBytes(), 0, bytes, 0, length);
return out.toByteArray();
}
catch (UnsupportedEncodingException ueex) {
throw new RuntimeException(ueex);
}
}
@Override
@Deprecated
public void consumeContent() throws IOException {
// TODO Auto-generated method stub
}
@Override
public InputStream getContent() throws IOException, IllegalStateException {
// TODO Auto-generated method stub
return null;
}
@Override
public boolean isChunked() {
// TODO Auto-generated method stub
return false;
}
@Override
public boolean isStreaming() {
// TODO Auto-generated method stub
return false;
}
@Override
public void writeTo(OutputStream out) throws IOException {
out.write(bytes);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy