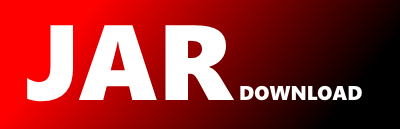
net.diversionmc.async.schedule.LoopState Maven / Gradle / Ivy
package net.diversionmc.async.schedule;
import net.diversionmc.async.Promise;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.atomic.AtomicInteger;
import java.util.function.Consumer;
/**
* State of a repeating schedule.
*
* @see Scheduler#scheduleRepeating(long, TimeUnit, Consumer) Main creator of objects of this class
* @see Promise#repeat(long, TimeUnit, Consumer) Main creator of repeating schedules
*/
public class LoopState {
private boolean stop = false;
private final AtomicInteger iteration;
/**
* Create a new loop state, backed by an integer pointer that may be changed by this loop holder, but not by the loop users.
*
* @param iteration Pointer to the iteration.
*/
public LoopState(AtomicInteger iteration) {
this.iteration = iteration;
}
/**
* Stop this loop.
*/
public void stop() {
stop = true;
}
/**
* Check if this loop has been stopped.
*
* @return Stop state.
*/
public boolean stopped() {
return stop;
}
/**
* Get the current iteration number of this loop, starting with 0.
*
* @return Iteration number.
*/
public int iteration() {
return iteration.get();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy