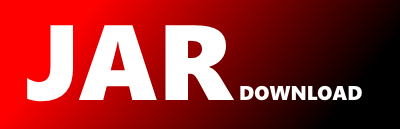
net.dona.doip.client.DoipSearchResults Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of doip-sdk Show documentation
Show all versions of doip-sdk Show documentation
DOIP Software Development Kit that implements DOIP v2 Specification.
package net.dona.doip.client;
import com.google.gson.stream.JsonReader;
import net.dona.doip.BadDoipException;
import net.dona.doip.InDoipMessage;
import net.dona.doip.InDoipSegment;
import net.dona.doip.client.transport.DoipClientResponse;
import net.dona.doip.util.InDoipMessageUtil;
import net.handle.hdllib.GsonUtility;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.Iterator;
import java.util.NoSuchElementException;
/**
* Used internally by {@link DoipClient} to produce search results
*
* @param either String for searchIds or DigitalObject for full search
*/
public class DoipSearchResults implements SearchResults {
private final DoipClientResponse resp;
private final InDoipMessage in;
private final JsonReader jsonReader;
private final Class klass;
private final int size;
private boolean closed = false;
public DoipSearchResults(DoipClientResponse resp, Class klass) throws IOException {
this.klass = klass;
this.resp = resp;
in = resp.getOutput();
InDoipSegment firstSegment = InDoipMessageUtil.getFirstSegment(in);
if (firstSegment == null) {
throw new BadDoipException("Missing input");
}
InputStream inputStream = firstSegment.getInputStream();
@SuppressWarnings("resource")
InputStreamReader isr = new InputStreamReader(inputStream, "UTF-8");
this.jsonReader = new JsonReader(isr);
jsonReader.beginObject();
@SuppressWarnings("hiding")
int size = -1;
while (jsonReader.hasNext()) {
String name = jsonReader.nextName();
if ("size".equals(name)) {
size = jsonReader.nextInt();
} else if ("results".equals(name)) {
jsonReader.beginArray();
break;
} else {
jsonReader.nextString();
}
}
this.size = size;
}
@Override
public int size() {
return size;
}
@Override
public Iterator iterator() {
return new DoipMessageIterator();
}
@Override
public synchronized void close() {
if (!closed) {
closed = true;
if (in != null) try { in.close(); } catch (Exception e) {}
if (resp != null) try { resp.close(); } catch (Exception e) {}
}
}
private class DoipMessageIterator implements Iterator {
private Boolean hasNextResult;
@Override
public boolean hasNext() {
if (hasNextResult != null) return hasNextResult.booleanValue();
if (closed) throw new IllegalStateException("Already closed");
try {
boolean res = jsonReader.hasNext();
hasNextResult = res;
if (res == false) {
close();
}
return res;
} catch (IOException e) {
throw new RuntimeException(new DoipException(e));
}
}
@Override
@SuppressWarnings("unchecked")
public T next() {
if (hasNextResult == null) hasNext();
if (!hasNextResult) throw new NoSuchElementException();
hasNextResult = null;
try {
if (klass == DigitalObject.class) {
DigitalObject dobj = GsonUtility.getGson().fromJson(jsonReader, DigitalObject.class);
return (T) dobj;
} else if (klass == String.class) {
String handle = jsonReader.nextString();
return (T) handle;
} else {
throw new AssertionError("Unexpected class " + klass);
}
} catch (Exception e) {
throw new RuntimeException(new DoipException(e));
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy