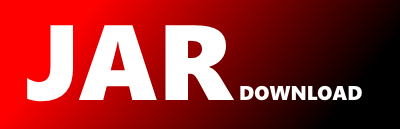
net.dona.doip.client.SearchResults Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of doip-sdk Show documentation
Show all versions of doip-sdk Show documentation
DOIP Software Development Kit that implements DOIP v2 Specification.
package net.dona.doip.client;
import java.util.Iterator;
import java.util.Spliterator;
import java.util.Spliterators;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
/**
* A representation of search results from {@link DoipClient} search operations.
* Provides either ids (an {code Iterable} or {@code Stream} of String objects) or
* Digital Objects (an {@code Iterable} or {@code Stream} of DigitalObject objects).
*
* @param either String for searchIds or DigitalObject for full search
*/
public interface SearchResults extends Iterable, AutoCloseable {
/**
* Returns the full number of results across all pages, or -1 if the number is not available
*/
int size();
/**
* Returns an {@code Iterator} of the search results (either String or DigitalObject).
*/
@Override
Iterator iterator();
/**
* Closes the search results, releasing the connection to the server.
*/
@Override
void close();
@Override
default Spliterator spliterator() {
int characteristics = Spliterator.IMMUTABLE | Spliterator.NONNULL | Spliterator.ORDERED;
if (size() >= 0) {
return Spliterators.spliterator(iterator(), size(), characteristics);
} else {
return Spliterators.spliteratorUnknownSize(iterator(), characteristics);
}
}
/**
* Returns an {@code Stream} of the search results (either String or DigitalObject).
*/
default Stream stream() {
Stream stream = StreamSupport.stream(spliterator(), false);
return stream.onClose(this::close);
}
/**
* Returns a potentially parallel {@code Stream} of the search results (either String or DigitalObject).
*/
default Stream parallelStream() {
Stream stream = StreamSupport.stream(spliterator(), true);
return stream.onClose(this::close);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy