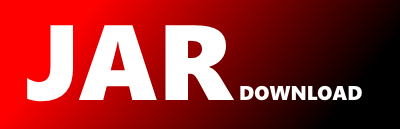
net.dongliu.prettypb.runtime.code.ProtoBufWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of prettypb-runtime Show documentation
Show all versions of prettypb-runtime Show documentation
Prettypb serialization runtime
package net.dongliu.prettypb.runtime.code;
import net.dongliu.prettypb.runtime.include.ProtoType;
import net.dongliu.prettypb.runtime.utils.Constant;
import java.io.Closeable;
import java.io.IOException;
import java.io.OutputStream;
/**
* write protobuf fields
*
* @author Dong Liu
*/
public class ProtoBufWriter implements Closeable {
private final OutputStream os;
public ProtoBufWriter(OutputStream os) {
this.os = os;
}
/**
* write Little-Endian base128 VarInt
*
* @param num
*/
public void writeVarInt(int num) throws IOException {
for (int i = 0; i < 5; i++) {
byte b = (byte) (num & 0x7f);
num = num >> 7;
if (num != 0) {
os.write((b | 0x80));
} else {
os.write(b);
break;
}
}
}
/**
* write Little-Endian base128 VarInt
*
* @param num
*/
public void writeVarLong(long num) throws IOException {
for (int i = 0; i < 10; i++) {
byte b = (byte) (num & 0x7f);
num = num >> 7;
if (num != 0) {
os.write((byte) (b | 0x80));
} else {
os.write(b);
break;
}
}
}
/**
* for protobuf sint32 type
*
* @param num
*/
public void writeVarSInt(int num) throws IOException {
writeVarInt(zigzag(num));
}
/**
* for protobuf sint64 type
*
* @param num
*/
public void writeVarSLong(long num) throws IOException {
writeVarLong(zigzag(num));
}
protected static int zigzag(int num) {
return (num << 1) ^ (num >> 31);
}
protected static long zigzag(long num) {
return (num << 1) ^ (num >> 63);
}
public void writeFixedInt(int num) throws IOException {
os.write((num & 0xff));
os.write(((num >> 8) & 0xff));
os.write(((num >> 16) & 0xff));
os.write(((num >> 24) & 0xff));
}
public void writeFloat(float num) throws IOException {
int i = Float.floatToRawIntBits(num);
writeFixedInt(i);
}
public void writeDouble(double num) throws IOException {
long l = Double.doubleToRawLongBits(num);
writeFixedLong(l);
}
public void writeFixedLong(long num) throws IOException {
os.write((int) (num & 0xff));
os.write((int) ((num >> 8) & 0xff));
os.write((int) ((num >> 16) & 0xff));
os.write((int) ((num >> 24) & 0xff));
os.write((int) ((num >> 32) & 0xff));
os.write((int) ((num >> 40) & 0xff));
os.write((int) ((num >> 48) & 0xff));
os.write((int) ((num >> 56) & 0xff));
}
public void writeString(String str) throws IOException {
byte[] bytes = str.getBytes(Constant.charset);
writeVarInt(bytes.length);
os.write(bytes);
}
public void writeBytes(byte[] bytes) throws IOException {
writeVarInt(bytes.length);
os.write(bytes);
}
public void writeByte(byte b) throws IOException {
os.write(b);
}
public void encodeTag(int wireType, int idx) throws IOException {
int i = idx << 3 | wireType;
writeVarInt(i);
}
public void encodeTag(ProtoType protoType, int idx) throws IOException {
encodeTag(protoType.getWireType(), idx);
}
@Override
public void close() throws IOException {
this.os.close();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy