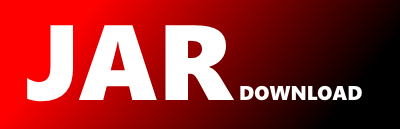
net.dongliu.prettypb.runtime.utils.BeanParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of prettypb-runtime Show documentation
Show all versions of prettypb-runtime Show documentation
Prettypb serialization runtime
package net.dongliu.prettypb.runtime.utils;
import net.dongliu.prettypb.runtime.include.ProtoBean;
import net.dongliu.prettypb.runtime.include.ProtoField;
import net.dongliu.prettypb.runtime.code.ProtoFieldInfo;
import net.dongliu.prettypb.runtime.code.ProtoInfo;
import net.dongliu.prettypb.runtime.exception.IllegalBeanException;
import java.lang.reflect.Field;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
/**
* parse proto types
*
* @author Dong Liu
*/
public class BeanParser {
private static volatile Map, ProtoInfo>> map = new HashMap<>();
private static final Lock lock = new ReentrantLock();
/**
* get proto type info. the result is cached
*
* @throws IllegalBeanException
*/
public static ProtoInfo getProtoInfo(Class clazz) throws IllegalBeanException {
ProtoInfo protoInfo = (ProtoInfo) map.get(clazz);
if (protoInfo != null) {
return protoInfo;
}
lock.lock();
try {
protoInfo = (ProtoInfo) map.get(clazz);
if (protoInfo != null) {
return protoInfo;
}
protoInfo = getProtoInfoInternal(clazz);
Map, ProtoInfo>> newMap = new HashMap<>();
newMap.putAll(map);
newMap.put(clazz, protoInfo);
map = newMap;
return protoInfo;
} finally {
lock.unlock();
}
}
private static ProtoInfo getProtoInfoInternal(Class clazz) throws IllegalBeanException {
ProtoBean protoBean = clazz.getAnnotation(ProtoBean.class);
if (protoBean == null) {
throw new IllegalBeanException("ProtoBean annotation not exists: " + clazz.getName());
}
Field[] fields = clazz.getDeclaredFields();
List protoFieldInfos = new ArrayList<>();
for (Field field : fields) {
ProtoField protoField = field.getAnnotation(ProtoField.class);
if (protoField == null) {
//ignore non-proto fields
continue;
}
protoField.getClass();
ProtoFieldInfo protoFieldInfo = ProtoFieldInfo.getProtoFieldInfo(clazz, field,
protoField);
protoFieldInfos.add(protoFieldInfo);
}
ProtoInfo protoInfo = new ProtoInfo();
protoInfo.setClazz(clazz);
protoInfo.addProtoFieldInfos(protoFieldInfos);
return protoInfo;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy