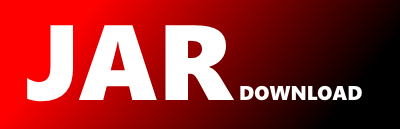
net.dongliu.apk.parser.utils.ResourceLoader Maven / Gradle / Ivy
package net.dongliu.apk.parser.utils;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.HashMap;
import java.util.Map;
/**
* methods for load resources.
*
* @author dongliu
*/
public class ResourceLoader {
/**
* load system attr ids for parse binary xml.
*/
public static Map loadSystemAttrIds() {
try (BufferedReader reader = toReader("/r_values.ini")) {
Map map = new HashMap<>();
String line;
while ((line = reader.readLine()) != null) {
String[] items = line.trim().split("=");
if (items.length != 2) {
continue;
}
String name = items[0].trim();
Integer id = Integer.valueOf(items[1].trim());
map.put(id, name);
}
return map;
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public static Map loadSystemStyles() {
Map map = new HashMap<>();
try (BufferedReader reader = toReader("/r_styles.ini")) {
String line;
while ((line = reader.readLine()) != null) {
line = line.trim();
String[] items = line.split("=");
if (items.length != 2) {
continue;
}
Integer id = Integer.valueOf(items[1].trim());
String name = items[0].trim();
map.put(id, name);
}
} catch (IOException e) {
throw new RuntimeException(e);
}
return map;
}
private static BufferedReader toReader(String path) {
return new BufferedReader(new InputStreamReader(
ResourceLoader.class.getResourceAsStream(path)));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy