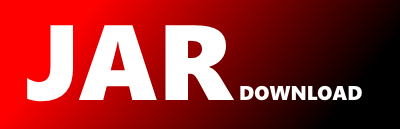
net.dongliu.commons.io.IO Maven / Gradle / Ivy
package net.dongliu.commons.io;
import java.io.*;
import java.nio.charset.Charset;
import java.nio.charset.StandardCharsets;
import java.util.function.Consumer;
import java.util.stream.Stream;
/**
* Utils method for io, only for internal use
*
* @author Liu Dong {@literal }
*/
public class IO {
private static final int BUFFER_SIZE = 8 * 1024;
/**
* Read input stream to string.
*
* @throws UncheckedIOException
*/
public static String toString(InputStream in, Charset charset) {
try (InputStreamReader isr = new InputStreamReader(in, charset);
BufferedReader br = new BufferedReader(isr)) {
char[] buf = new char[BUFFER_SIZE];
int count;
StringBuilder sb = new StringBuilder();
while ((count = br.read(buf)) != -1) {
sb.append(buf, 0, count);
}
return sb.toString();
} catch (IOException e) {
throw new UncheckedIOException(e);
}
}
/**
* Read input stream to byte array
*/
public static byte[] toBytes(InputStream in) {
try {
try (InputStream is = in;
ByteArrayOutputStream bos = new ByteArrayOutputStream()) {
byte[] buf = new byte[BUFFER_SIZE];
int count;
while ((count = is.read(buf)) != -1) {
bos.write(buf, 0, count);
}
return bos.toByteArray();
}
} catch (IOException e) {
throw new UncheckedIOException(e);
}
}
/**
* Copy data from inputStream to outputStream. InputStream will be closed after copy finished.
*/
public static void copy(InputStream in, OutputStream out) {
try {
byte[] buf = new byte[BUFFER_SIZE];
int count;
while ((count = in.read(buf)) != -1) {
out.write(buf, 0, count);
}
} catch (IOException e) {
throw new UncheckedIOException(e);
} finally {
closeQuietly(in);
}
}
/**
* Convert to stream of lines. reader will be closed after stream finished
*/
public static Stream toLineStream(BufferedReader reader) {
try {
return reader.lines().onClose(() -> {
try {
reader.close();
} catch (IOException e) {
throw new UncheckedIOException(e);
}
});
} catch (Error | RuntimeException e) {
closeQuietly(reader);
throw e;
}
}
/**
* Convert to stream of lines. reader will be closed after stream finished
*/
public static Stream toLineStream(InputStream in, Charset charset) {
try (InputStream is = in;
Reader reader = new InputStreamReader(is, charset)) {
return toLineStream(new BufferedReader(reader));
} catch (IOException e) {
throw new UncheckedIOException(e);
}
}
/**
* Convert to stream of lines. reader will be closed after stream finished
*/
public static Stream toLineStream(InputStream in) {
return toLineStream(in, StandardCharsets.UTF_8);
}
/**
* Close and ignore IOException
*/
public static void closeQuietly(AutoCloseable closeable) {
try {
closeable.close();
} catch (Exception ignore) {
}
}
/**
* Consume input stream data
*/
public static void forEachChunk(InputStream in, ChunkReceiver receiver) {
byte[] buffer = new byte[BUFFER_SIZE];
int len;
try {
while ((len = in.read(buffer)) != -1) {
receiver.onData(buffer, len);
}
} catch (IOException e) {
throw new UncheckedIOException(e);
} finally {
closeQuietly(in);
}
}
/**
* Consume Each line of reader
*/
public static void forEachLine(Reader reader, Consumer consumer) {
try (BufferedReader br = new BufferedReader(reader)) {
String line;
while ((line = br.readLine()) != null) {
consumer.accept(line);
}
} catch (IOException e) {
throw new UncheckedIOException(e);
} finally {
closeQuietly(reader);
}
}
/**
* Discard all data in stream and close
*/
public static void consume(InputStream in) {
try {
while (in.skip(BUFFER_SIZE) != 0) ;
byte[] buf = new byte[BUFFER_SIZE];
while (in.read(buf) != -1) ;
} catch (IOException e) {
throw new UncheckedIOException(e);
} finally {
closeQuietly(in);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy