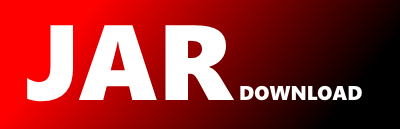
net.dongliu.commons.reflect.Property Maven / Gradle / Ivy
package net.dongliu.commons.reflect;
import net.dongliu.commons.Exceptions;
import java.lang.invoke.MethodHandle;
/**
* @author Liu Dong
*/
public abstract class Property {
public abstract String name();
protected abstract MethodHandle getter();
protected abstract MethodHandle setter();
public abstract boolean canRead();
public abstract boolean canWrite();
public Object get(Object bean) {
try {
return getter().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void set(Object bean, Object value) {
try {
setter().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public byte getByte(Object bean) {
try {
return (byte) getter().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void setByte(Object bean, byte value) {
try {
setter().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public short getShort(Object bean) {
try {
return (short) getter().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void setShort(Object bean, short value) {
try {
setter().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public int getInt(Object bean) {
try {
return (int) getter().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void setInt(Object bean, int value) {
try {
setter().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public long getLong(Object bean) {
try {
return (long) getter().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void setLong(Object bean, long value) {
try {
setter().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public double getDouble(Object bean) {
try {
return (double) getter().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void setDouble(Object bean, double value) {
try {
setter().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public float getFloat(Object bean) {
try {
return (float) getter().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void setFloat(Object bean, float value) {
try {
setter().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public boolean getBoolean(Object bean) {
try {
return (boolean) getter().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void setBoolean(Object bean, boolean value) {
try {
setter().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public char getChar(Object bean) {
try {
return (char) getter().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void setChar(Object bean, char value) {
try {
setter().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy