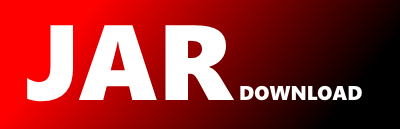
net.dongliu.commons.reflect.StaticField Maven / Gradle / Ivy
package net.dongliu.commons.reflect;
import net.dongliu.commons.Exceptions;
import net.dongliu.commons.exception.ReflectException;
import javax.annotation.Nullable;
import java.lang.invoke.MethodHandle;
import java.lang.invoke.MethodHandles;
import java.lang.reflect.Field;
import java.lang.reflect.Modifier;
import java.util.Objects;
/**
* @author Liu Dong
*/
public class StaticField {
private final Field field;
@Nullable
private final MethodHandle getter;
@Nullable
private final MethodHandle setter;
public StaticField(Field field) {
Objects.requireNonNull(field);
if (!Modifier.isStatic(field.getModifiers())) {
throw new IllegalArgumentException("Field should be static");
}
this.field = field;
field.setAccessible(true);
this.getter = getGetter(field);
this.setter = getSetter(field);
}
private MethodHandle getGetter(Field field) {
MethodHandles.Lookup lookup = MethodHandles.lookup();
try {
return lookup.unreflectGetter(field);
} catch (IllegalAccessException e) {
throw new IllegalArgumentException(e);
}
}
@Nullable
private MethodHandle getSetter(Field field) {
MethodHandles.Lookup lookup = MethodHandles.lookup();
if (!Modifier.isFinal(field.getModifiers())) {
try {
return lookup.unreflectSetter(field);
} catch (IllegalAccessException e) {
throw new ReflectException(e);
}
} else {
return null;
}
}
public String name() {
return field.getName();
}
public MethodHandle getter() {
return getter;
}
public MethodHandle setter() {
if (setter == null) {
throw new ReflectException(new IllegalAccessException("Filed can not write"));
}
return setter;
}
public boolean canRead() {
return true;
}
public boolean canWrite() {
return !Modifier.isFinal(field.getModifiers());
}
public Object get() {
try {
return getter().invoke();
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void set(Object value) {
try {
setter().invoke(value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public byte getByte() {
try {
return (byte) getter().invokeExact();
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void setByte(byte value) {
try {
setter().invokeExact(value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public short getShort() {
try {
return (short) getter().invokeExact();
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void setShort(short value) {
try {
setter().invokeExact(value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public int getInt() {
try {
return (int) getter().invokeExact();
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void setInt(int value) {
try {
setter().invokeExact(value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public long getLong() {
try {
return (long) getter().invokeExact();
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void setLong(long value) {
try {
setter().invokeExact(value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public double getDouble() {
try {
return (double) getter().invokeExact();
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void setDouble(double value) {
try {
setter().invokeExact(value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public float getFloat() {
try {
return (float) getter().invokeExact();
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void setFloat(float value) {
try {
setter().invokeExact(value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public boolean getBoolean() {
try {
return (boolean) getter().invokeExact();
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void setBoolean(boolean value) {
try {
setter().invokeExact(value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public char getChar() {
try {
return (char) getter().invokeExact();
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void setChar(char value) {
try {
setter().invokeExact(value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy