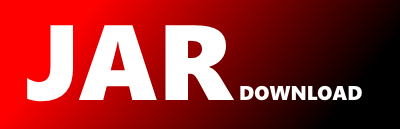
net.dongliu.commons.collection.Pair Maven / Gradle / Ivy
package net.dongliu.commons.collection;
import javax.annotation.Nullable;
import java.io.Serializable;
import java.util.Map;
/**
* Immutable pair
*
* @author Liu Dong
*/
public class Pair implements Map.Entry, Serializable {
private static final long serialVersionUID = -6525353427059094141L;
private final A first;
private final B second;
public Pair(A first, B second) {
this.first = first;
this.second = second;
}
public A first() {
return first;
}
public B second() {
return second;
}
public A getKey() {
return first;
}
/**
* Create a new pair with new key
*/
public Pair withKey(A newKey) {
return new Pair<>(newKey, second);
}
public A getName() {
return first;
}
/**
* Create a new pair with new key
*/
public Pair withName(A newKey) {
return new Pair<>(newKey, second);
}
public B getValue() {
return second;
}
@Override
public B setValue(B value) {
throw new UnsupportedOperationException("Pair is read only");
}
/**
* Create a new pair with new value
*/
public Pair withValue(B newValue) {
return new Pair<>(first, newValue);
}
@Override
public boolean equals(@Nullable Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Pair, ?> pair = (Pair, ?>) o;
if (first != null ? !first.equals(pair.first) : pair.first != null) return false;
return !(second != null ? !second.equals(pair.second) : pair.second != null);
}
@Override
public int hashCode() {
int result = first != null ? first.hashCode() : 0;
result = 31 * result + (second != null ? second.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "(" + first + "," + second + ")";
}
public static Pair of(K key, V value) {
return new Pair<>(key, value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy