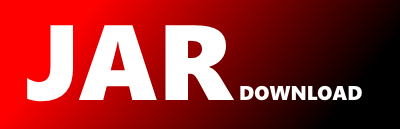
net.dongliu.commons.reflect.Property Maven / Gradle / Ivy
package net.dongliu.commons.reflect;
import net.dongliu.commons.Exceptions;
import java.lang.invoke.MethodHandle;
/**
* Property for class, implemented by getter/setter or field
*
* @author Liu Dong
*/
public interface Property {
/**
* The property's name
*/
String name();
Class> type();
MethodHandle getter();
MethodHandle setter();
/**
* If property has read method
*/
boolean canRead();
/**
* If property has write method
*/
boolean canWrite();
/**
* Bind to a instance
*/
default StandaloneProperty bindTo(Object instance) {
return new BindedProperty(instance, this);
}
default Object get(Object bean) {
try {
return getter().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
default void set(Object bean, Object value) {
try {
setter().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
default byte getByte(Object bean) {
try {
return (byte) getter().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
default void setByte(Object bean, byte value) {
try {
setter().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
default short getShort(Object bean) {
try {
return (short) getter().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
default void setShort(Object bean, short value) {
try {
setter().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
default int getInt(Object bean) {
try {
return (int) getter().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
default void setInt(Object bean, int value) {
try {
setter().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
default long getLong(Object bean) {
try {
return (long) getter().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
default void setLong(Object bean, long value) {
try {
setter().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
default double getDouble(Object bean) {
try {
return (double) getter().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
default void setDouble(Object bean, double value) {
try {
setter().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
default float getFloat(Object bean) {
try {
return (float) getter().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
default void setFloat(Object bean, float value) {
try {
setter().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
default boolean getBoolean(Object bean) {
try {
return (boolean) getter().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
default void setBoolean(Object bean, boolean value) {
try {
setter().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
default char getChar(Object bean) {
try {
return (char) getter().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
default void setChar(Object bean, char value) {
try {
setter().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy