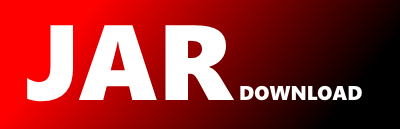
net.dongliu.commons.collection.RawValue Maven / Gradle / Ivy
package net.dongliu.commons.collection;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.lang.reflect.Array;
import java.util.List;
import java.util.Map;
import java.util.Objects;
/**
* Collection wrapper to handle raw list[String] / map[String, Object] collections
*
* @author Liu Dong
*/
public class RawValue {
private static final RawValue nil = new RawValue(null);
@Nullable
private final Object value;
private RawValue(@Nullable Object value) {
this.value = value;
}
/**
* Wrap collection value
*
* @param value a list, map, array or one plain value
*/
@Nonnull
public static RawValue of(Object value) {
return new RawValue(Objects.requireNonNull(value));
}
/**
* Get value at index, current value is array or list.
*
* @throws IndexOutOfBoundsException
*/
@Nonnull
public RawValue get(int index) {
if (value == null) {
return nil;
}
if (value instanceof List) {
return new RawValue(((List) value).get(index));
} else if (value.getClass().isArray()) {
return new RawValue(Array.get(value, index));
} else {
throw new UnsupportedOperationException("Type " + value.getClass() + " cannot get by index");
}
}
/**
* Get value with key, current value map.
*
* @throws IndexOutOfBoundsException
*/
@Nonnull
public RawValue get(String key) {
if (value == null) {
return nil;
}
if (value instanceof Map) {
return new RawValue(((Map) value).get(key));
} else {
throw new UnsupportedOperationException("Type " + value.getClass() + " cannot get by index");
}
}
/**
* Get current value as type T
*/
@Nullable
@SuppressWarnings("unchecked")
public T value() {
return (T) value;
}
/**
* Get current value as type T. if value is null, return default value
*/
@Nonnull
@SuppressWarnings("unchecked")
public T orElse(T defaultValue) {
Objects.requireNonNull(defaultValue);
if (value != null) {
return (T) value;
} else {
return defaultValue;
}
}
/**
* If has value
*/
public boolean present() {
return value != null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy