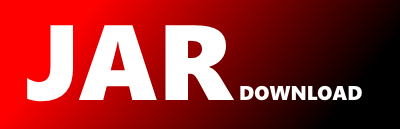
net.dongliu.commons.reflect.AbstractProperty Maven / Gradle / Ivy
package net.dongliu.commons.reflect;
import net.dongliu.commons.exception.Exceptions;
import javax.annotation.Nonnull;
import java.lang.invoke.MethodHandle;
/**
* Property for class, implemented by getterHandle/setter or field
*
* @author Liu Dong
*/
public abstract class AbstractProperty {
/**
* The property's name
*/
abstract String name();
abstract Class> type();
/**
* Only for internal use
*/
@Nonnull
protected abstract MethodHandle getterHandle();
/**
* Only for internal use
*/
@Nonnull
protected abstract MethodHandle setterHandle();
/**
* If property has read method
*/
abstract boolean canRead();
/**
* If property has write method
*/
abstract boolean canWrite();
/**
* Bind to a instance
*/
public StandaloneProperty bindTo(Object instance) {
return new BoundProperty(instance, this);
}
public Object get(Object bean) {
try {
return getterHandle().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void set(Object bean, Object value) {
try {
setterHandle().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public byte getByte(Object bean) {
try {
return (byte) getterHandle().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void setByte(Object bean, byte value) {
try {
setterHandle().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public short getShort(Object bean) {
try {
return (short) getterHandle().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void setShort(Object bean, short value) {
try {
setterHandle().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public int getInt(Object bean) {
try {
return (int) getterHandle().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void setInt(Object bean, int value) {
try {
setterHandle().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public long getLong(Object bean) {
try {
return (long) getterHandle().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void setLong(Object bean, long value) {
try {
setterHandle().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public double getDouble(Object bean) {
try {
return (double) getterHandle().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void setDouble(Object bean, double value) {
try {
setterHandle().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public float getFloat(Object bean) {
try {
return (float) getterHandle().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void setFloat(Object bean, float value) {
try {
setterHandle().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public boolean getBoolean(Object bean) {
try {
return (boolean) getterHandle().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void setBoolean(Object bean, boolean value) {
try {
setterHandle().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public char getChar(Object bean) {
try {
return (char) getterHandle().invoke(bean);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
public void setChar(Object bean, char value) {
try {
setterHandle().invoke(bean, value);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy