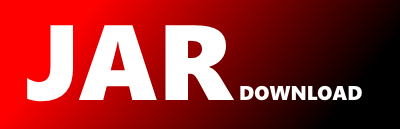
net.dongliu.commons.reflect.StaticMethod Maven / Gradle / Ivy
package net.dongliu.commons.reflect;
import net.dongliu.commons.exception.Exceptions;
import java.lang.invoke.MethodHandle;
import java.lang.invoke.MethodHandles;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.util.Objects;
/**
* Static class method
*
* @author Liu Dong
*/
public class StaticMethod extends AbstractMethod {
private final Method method;
private final MethodHandle methodHandle;
public StaticMethod(Method method) {
Objects.requireNonNull(method);
if (!Modifier.isStatic(method.getModifiers())) {
throw new IllegalArgumentException("Method should be static");
}
this.method = method;
this.method.setAccessible(true);
MethodHandles.Lookup lookup = MethodHandles.lookup();
try {
methodHandle = lookup.unreflect(method);
} catch (IllegalAccessException e) {
throw Exceptions.sneakyThrow(e);
}
}
/**
* Return method name
*/
public String name() {
return method.getName();
}
public Method getMethod() {
return method;
}
/**
* Invoke method with return values
*/
public Object call(Object... args) {
if (method.getReturnType() == void.class) {
// no return value
run(args);
return null;
}
try {
return methodHandle.invokeWithArguments(args);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
/**
* Invoke method with no value
*/
public void run(Object... args) {
try {
methodHandle.invokeWithArguments(args);
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
/**
* Invoke method with return values
*/
public Object call() {
if (method.getReturnType() == void.class) {
// no return value
run();
return null;
}
try {
return methodHandle.invoke();
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
/**
* Invoke method with no value
*/
public void run() {
try {
methodHandle.invoke();
} catch (Throwable throwable) {
throw Exceptions.sneakyThrow(throwable);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy