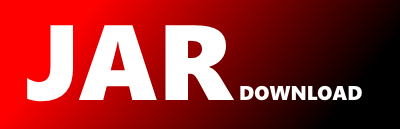
net.dongliu.commons.Marshaller Maven / Gradle / Ivy
package net.dongliu.commons;
import net.dongliu.commons.exception.UncheckedReflectionException;
import javax.annotation.Nullable;
import java.io.*;
/**
* Marshal and Unmarshal using jvm serialization
*
* @author Liu Dong
*/
public class Marshaller {
/**
* Marshal object to bytes
*/
public static byte[] marshal(@Nullable Object value) {
try (ByteArrayOutputStream bos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(bos)) {
oos.writeObject(value);
return bos.toByteArray();
} catch (IOException e) {
throw new UncheckedIOException(e);
}
}
/**
* Marshal object to bytes. output will be closed after write finished
*/
public static void marshal(OutputStream output, @Nullable Object value) {
try (ObjectOutputStream oos = new ObjectOutputStream(output)) {
oos.writeObject(value);
} catch (IOException e) {
throw new UncheckedIOException(e);
}
}
/**
* Unmarshal bytes to object
*/
public static Object unmarshal(byte[] bytes) {
try (ByteArrayInputStream bis = new ByteArrayInputStream(bytes);
ObjectInputStream ois = new ObjectInputStream(bis)) {
return ois.readObject();
} catch (IOException e) {
throw new UncheckedIOException(e);
} catch (ClassNotFoundException e) {
throw new UncheckedReflectionException(e);
}
}
/**
* Unmarshal to object from input stream. input will be closed after read finished
*/
public static Object unmarshal(InputStream input) {
try (ObjectInputStream ois = new ObjectInputStream(input)) {
return ois.readObject();
} catch (IOException e) {
throw new UncheckedIOException(e);
} catch (ClassNotFoundException e) {
throw new UncheckedReflectionException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy