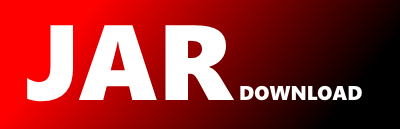
net.dongliu.commons.Predicates Maven / Gradle / Ivy
package net.dongliu.commons;
import javax.annotation.Nullable;
import java.util.Collection;
/**
* @author Liu Dong
*/
public class Predicates {
/**
* Require all value to be non-null
*
* @return the values
*/
@SafeVarargs
public static T[] requireValuesNonNull(@Nullable T... values) {
if (values == null) {
throw new NullPointerException();
}
for (T value : values) {
if (value == null) {
throw new NullPointerException();
}
}
return values;
}
/**
* Require all value to be non-null
*
* @return the values
*/
public static T[] requireValuesNonNull(@Nullable T[] values, String msg) {
if (values == null) {
throw new NullPointerException(msg);
}
for (T value : values) {
if (value == null) {
throw new NullPointerException(msg);
}
}
return values;
}
/**
* Require all value to be non-null
*
* @return the values
*/
public static , T> S requireValuesNonNull(@Nullable S values, String msg) {
if (values == null) {
throw new NullPointerException(msg);
}
for (T value : values) {
if (value == null) {
throw new NullPointerException(msg);
}
}
return values;
}
/**
* Require all value to be non-null
*
* @return the values
*/
public static , T> S requireValuesNonNull(@Nullable S values) {
if (values == null) {
throw new NullPointerException();
}
for (T value : values) {
if (value == null) {
throw new NullPointerException();
}
}
return values;
}
/**
* Require argument to satisfy condition, or throw IllegalArgument exception
*/
public static void requireArgument(boolean condition, String msg) {
if (!condition) {
throw new IllegalArgumentException(msg);
}
}
/**
* Require argument to satisfy condition, or throw IllegalArgument exception
*/
public static void requireArgument(boolean condition) {
if (!condition) {
throw new IllegalArgumentException();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy