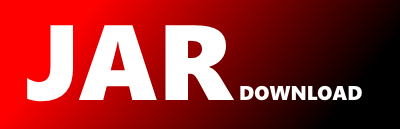
net.dongliu.commons.collection.Maps Maven / Gradle / Ivy
package net.dongliu.commons.collection;
import javax.annotation.Nullable;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
/**
* Utils method for map
*
* @author Liu Dong
*/
public class Maps {
/**
* Map is null or empty
*/
public static boolean isNullOrEmpty(@Nullable Map, ?> map) {
return map == null || map.isEmpty();
}
/**
* If map is null, return immutable empty map; else return self
*/
public static Map nullToEmpty(@Nullable Map map) {
return map == null ? Maps.of() : map;
}
/**
* Create immutable view of this Set.
*/
public static Map asImmutable(Map map) {
return Collections.unmodifiableMap(map);
}
/**
* Create a immutable map with defensive copy
*/
public static Map toImmutable(Map map) {
return Collections.unmodifiableMap(new HashMap<>(map));
}
/**
* Create immutable empty map
*/
public static Map of() {
return Collections.emptyMap();
}
/**
* Create immutable map
*/
public static Map of(Map.Entry extends K, ? extends V> value) {
Map map = create(1);
map.put(value.getKey(), value.getValue());
return asImmutable(map);
}
/**
* Create immutable map
*/
public static Map of(Map.Entry extends K, ? extends V> value1,
Map.Entry extends K, ? extends V> value2) {
Map map = create(2);
map.put(value1.getKey(), value1.getValue());
map.put(value2.getKey(), value2.getValue());
return asImmutable(map);
}
/**
* Create immutable map
*/
public static Map of(Map.Entry extends K, ? extends V> value1,
Map.Entry extends K, ? extends V> value2,
Map.Entry extends K, ? extends V> value3) {
Map map = create(3);
map.put(value1.getKey(), value1.getValue());
map.put(value2.getKey(), value2.getValue());
map.put(value3.getKey(), value3.getValue());
return asImmutable(map);
}
/**
* Create immutable map
*/
public static Map of(Map.Entry extends K, ? extends V> value1,
Map.Entry extends K, ? extends V> value2,
Map.Entry extends K, ? extends V> value3,
Map.Entry extends K, ? extends V> value4) {
Map map = create(4);
map.put(value1.getKey(), value1.getValue());
map.put(value2.getKey(), value2.getValue());
map.put(value3.getKey(), value3.getValue());
map.put(value4.getKey(), value4.getValue());
return asImmutable(map);
}
/**
* Create immutable map
*/
public static Map of(Map.Entry extends K, ? extends V> value1,
Map.Entry extends K, ? extends V> value2,
Map.Entry extends K, ? extends V> value3,
Map.Entry extends K, ? extends V> value4,
Map.Entry extends K, ? extends V> value5) {
Map map = create(5);
map.put(value1.getKey(), value1.getValue());
map.put(value2.getKey(), value2.getValue());
map.put(value3.getKey(), value3.getValue());
map.put(value4.getKey(), value4.getValue());
map.put(value5.getKey(), value5.getValue());
return asImmutable(map);
}
@SafeVarargs
public static Map of(Map.Entry extends K, ? extends V>... values) {
if (values.length == 0) {
return of();
}
return asImmutable(create(values));
}
public static Map of(Collection extends Map.Entry> values) {
return asImmutable(create(values));
}
/**
* Create mutable empty map, with expected size
*/
public static Map create(int expectedSize) {
return new HashMap<>((int) (expectedSize / 0.75f) + 1, 0.75f);
}
/**
* Create mutable empty map
*/
public static Map create() {
return new HashMap<>();
}
/**
* Create mutable map
*/
public static Map create(Map.Entry extends K, ? extends V> value) {
Map map = create();
map.put(value.getKey(), value.getValue());
return map;
}
/**
* Create mutable map
*/
public static Map create(Map.Entry extends K, ? extends V> value1,
Map.Entry extends K, ? extends V> value2) {
Map map = create();
map.put(value1.getKey(), value1.getValue());
map.put(value2.getKey(), value2.getValue());
return map;
}
/**
* Create mutable map
*/
public static Map create(Map.Entry extends K, ? extends V> value1,
Map.Entry extends K, ? extends V> value2,
Map.Entry extends K, ? extends V> value3) {
Map map = create();
map.put(value1.getKey(), value1.getValue());
map.put(value2.getKey(), value2.getValue());
map.put(value3.getKey(), value3.getValue());
return map;
}
/**
* Create mutable map
*/
public static Map create(Map.Entry extends K, ? extends V> value1,
Map.Entry extends K, ? extends V> value2,
Map.Entry extends K, ? extends V> value3,
Map.Entry extends K, ? extends V> value4) {
Map map = create();
map.put(value1.getKey(), value1.getValue());
map.put(value2.getKey(), value2.getValue());
map.put(value3.getKey(), value3.getValue());
map.put(value4.getKey(), value4.getValue());
return map;
}
/**
* Create mutable map
*/
public static Map create(Map.Entry extends K, ? extends V> value1,
Map.Entry extends K, ? extends V> value2,
Map.Entry extends K, ? extends V> value3,
Map.Entry extends K, ? extends V> value4,
Map.Entry extends K, ? extends V> value5) {
Map map = create();
map.put(value1.getKey(), value1.getValue());
map.put(value2.getKey(), value2.getValue());
map.put(value3.getKey(), value3.getValue());
map.put(value4.getKey(), value4.getValue());
map.put(value5.getKey(), value5.getValue());
return map;
}
@SafeVarargs
public static Map create(Map.Entry extends K, ? extends V>... values) {
Map map = create(values.length);
for (Map.Entry extends K, ? extends V> tuple : values) {
map.put(tuple.getKey(), tuple.getValue());
}
return map;
}
public static Map create(Collection extends Map.Entry> values) {
Map map = create(values.size());
for (Map.Entry tuple : values) {
map.put(tuple.getKey(), tuple.getValue());
}
return map;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy