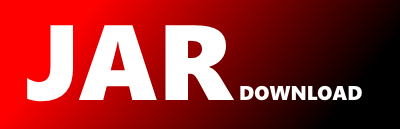
net.dongliu.commons.collection.Pair Maven / Gradle / Ivy
package net.dongliu.commons.collection;
import java.io.Serializable;
import java.util.Map;
/**
* Immutable Pair/Tuple2.
*
* @param the key type
* @param the value type
*/
public class Pair implements Map.Entry, Serializable {
private static final long serialVersionUID = -6468644647295390377L;
private final K key;
private final V value;
private Pair(K key, V value) {
this.key = key;
this.value = value;
}
/**
* Create a new pair
*/
public static Pair of(K key, V value) {
return new Pair<>(key, value);
}
@Override
public K getKey() {
return key;
}
@Override
public V getValue() {
return value;
}
/**
* Create one new pair, replace the key with newKey.
*/
public Pair withKey(K newKey) {
return new Pair<>(newKey, value);
}
/**
* Create one new pair, replace the value with newValue.
*/
public Pair withValue(V newValue) {
return new Pair<>(key, newValue);
}
/**
* The first value of this tuple
*/
public K first() {
return key;
}
/**
* The second value of this tuple
*/
public V second() {
return value;
}
/**
* Create one new pair, replace the first value with new value.
*/
public Pair withFirst(K first) {
return new Pair<>(first, second());
}
/**
* Create one new pair, replace the second value with new value.
*/
public Pair withSecond(V second) {
return new Pair<>(first(), second);
}
/**
* Append a new value.
*
* @param third the new value
* @return Triple hold the Pair's value and new value
*/
public Triple append(C third) {
return Triple.of(first(), second(), third);
}
/**
* Prepend a new value.
*
* @param value the new value
* @return Triple hold new value and the Pair's value
*/
public Triple prepend(A value) {
return Triple.of(value, first(), second());
}
@Override
public V setValue(V value) {
throw new UnsupportedOperationException();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Pair, ?> pair = (Pair, ?>) o;
if (key != null ? !key.equals(pair.key) : pair.key != null) return false;
return value != null ? value.equals(pair.value) : pair.value == null;
}
@Override
public int hashCode() {
int result = key != null ? key.hashCode() : 0;
result = 31 * result + (value != null ? value.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "(" + key + ", " + value + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy