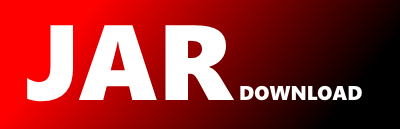
donky.microsoft.aspnet.signalr.client.http.HttpConnectionFuture Maven / Gradle / Ivy
/*
Copyright (c) Microsoft Open Technologies, Inc.
All Rights Reserved
See License.txt in the project root for license information.
*/
package donky.microsoft.aspnet.signalr.client.http;
import java.util.Queue;
import java.util.concurrent.ConcurrentLinkedQueue;
import donky.microsoft.aspnet.signalr.client.ErrorCallback;
import donky.microsoft.aspnet.signalr.client.SignalRFuture;
/**
* A SinglaRFuture for Http operations
*/
public class HttpConnectionFuture extends SignalRFuture {
private Queue mTimeoutQueue = new ConcurrentLinkedQueue();
private ErrorCallback mTimeoutCallback;
private Object mTimeoutLock = new Object();
/**
* Handles the timeout for an Http operation
*
* @param errorCallback
* The handler
*/
public void onTimeout(ErrorCallback errorCallback) {
synchronized (mTimeoutLock) {
mTimeoutCallback = errorCallback;
while (!mTimeoutQueue.isEmpty()) {
if (mTimeoutCallback != null) {
mTimeoutCallback.onError(mTimeoutQueue.poll());
}
}
}
}
/**
* Triggers the timeout error
*
* @param error
* The error
*/
public void triggerTimeout(Throwable error) {
synchronized (mTimeoutLock) {
if (mTimeoutCallback != null) {
mTimeoutCallback.onError(error);
} else {
mTimeoutQueue.add(error);
}
}
}
/**
* Represents the callback to invoke when a response is returned after a
* request
*/
public interface ResponseCallback {
/**
* Callback invoked when a response is returned by the request
*
* @param response
* The returned response
*/
public void onResponse(Response response) throws Exception;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy