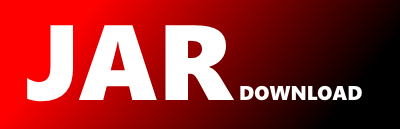
com.amazonaws.mws.MarketplaceWebServiceConfig Maven / Gradle / Ivy
The newest version!
/*******************************************************************************
* Copyright 2009 Amazon Services.
* Licensed under the Apache License, Version 2.0 (the "License");
*
* You may not use this file except in compliance with the License.
* You may obtain a copy of the License at: http://aws.amazon.com/apache2.0
* This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
* *****************************************************************************
*
* Marketplace Web Service Java Library
* API Version: 2009-01-01
* Generated: Tue Feb 03 15:59:58 PST 2009
*
*/
package com.amazonaws.mws;
/**
* Configuration for accessing Marketplace Web Service service
*/
public class MarketplaceWebServiceConfig {
public enum ProxyProtocol { HTTP, HTTPS };
private int connectionTimeout = 50000;
private int socketTimeout = 50000;
private String serviceVersion = "2009-01-01";
private String serviceURL = null;
private String userAgent = null;
private String signatureMethod = "HmacSHA256";
private String proxyHost = null;
private ProxyProtocol proxyProtocol = null;
private int proxyPort = -1;
private String proxyUsername = null;
private String proxyPassword = null;
private int maxErrorRetry = 3;
private int maxAsyncThreads = 30;
private int maxAsyncQueueSize = maxAsyncThreads * 10;
/**
* Gets Version of the API
*
* @return Version of the Service
*/
public String getServiceVersion() {
return serviceVersion;
}
/**
* Gets ConnectionTimeout property
*
* @return Connection Timeout for establishing connections
*/
public int getConnectionTimeout() {
return connectionTimeout;
}
/**
* Sets ConnectionTimeout property
*
* @param connectionTimeout Timeout until connection is established
*/
public void setConnectionTimeout(int connectionTimeout) {
this.connectionTimeout = connectionTimeout;
}
/**
* Sets ConnectionTimeout property and returns current MarketplaceWebServiceConfig
*
* @param connectionTimeout until connection is established
*
* @return MarketplaceWebServiceConfig
*/
public MarketplaceWebServiceConfig withConnectionTimeout(int connectionTimeout) {
setConnectionTimeout(connectionTimeout);
return this;
}
/**
* Checks if ConnectionTimeout property is set
*
* @return true if ConnectionTimeout property is set
*/
public boolean isSetConnectionTimeout() {
return true;
}
/**
* Gets SocketTimeout property
*
* @return Socket Timeout for waiting for data
*/
public int getSoTimeout() {
return socketTimeout;
}
/**
* Sets SocketTimeout property
*
* @param socketTimeout Timeout for waiting for data
*/
public void setSoTimeout(int socketTimeout) {
this.socketTimeout = socketTimeout;
}
/**
* Sets SocketTimeout property and returns current MarketplaceWebServiceConfig
*
* @param socketTimeout for waiting for data
*
* @return MarketplaceWebServiceConfig
*/
public MarketplaceWebServiceConfig withSoTimeout(int socketTimeout) {
setSoTimeout(socketTimeout);
return this;
}
/**
* Checks if SocketTimeout property is set
*
* @return true if SocketTimeout property is set
*/
public boolean isSetSoTimeout() {
return true;
}
/**
* Gets SignatureMethod property
*
* @return Signature Method for signing requests
*/
public String getSignatureMethod() {
return signatureMethod;
}
/**
* Sets SignatureMethod property
*
* @param signatureMethod Signature Method for signing requests
*/
public void setSignatureMethod(String signatureMethod) {
this.signatureMethod = signatureMethod;
}
/**
* Sets SignatureMethod property and returns current MarketplaceWebServiceConfig
*
* @param signatureMethod Signature Method for signing requests
*
* @return MarketplaceWebServiceConfig
*/
public MarketplaceWebServiceConfig withSignatureMethod(String signatureMethod) {
setSignatureMethod(signatureMethod);
return this;
}
/**
* Checks if SignatureMethod property is set
*
* @return true if SignatureMethod property is set
*/
public boolean isSetSignatureMethod() {
return true;
}
/**
* Gets UserAgent property
*
* @return User Agent String to use when sending request
*/
public String getUserAgent() {
return userAgent;
}
/**
* Sets UserAgent property
*
* @param applicationName User Agent String to use when sending request
* @param additionalNameValuePairs additionalNameValuePairs
* @param applicationVersion applicationVersion
* @param programmingLanguage programmingLanguage
*/
public void setUserAgent(
String applicationName,
String applicationVersion,
String programmingLanguage,
String... additionalNameValuePairs) {
if(applicationName==null) throw new IllegalArgumentException(
"applicationName cannot be NULL");
if(applicationVersion==null) throw new IllegalArgumentException(
"applicationVersion cannot be NULL");
if(programmingLanguage==null) throw new IllegalArgumentException(
"programmingLanguage cannot be NULL");
if(additionalNameValuePairs.length % 2 != 0) throw new IllegalArgumentException(
"there must be a matching value for every name you pass in");
StringBuilder b = new StringBuilder();
b.append(applicationName);
b.append("/");
b.append(applicationVersion);
b.append(" (Language=");
b.append(programmingLanguage);
int i=0;
while(i 0;
}
/**
* Gets MaxAsyncThreads property
*
* @return Max number of threads to be used for async operations
*/
public int getMaxAsyncThreads() {
return maxAsyncThreads;
}
/**
* Sets MaxAsyncThreads property
*
* @param maxAsyncThreads Max number of threads to spawn for async operation.
* Note, this parameter works in conjuction with MaxAsyncQueueSize. When
* Max number of threads is reached, requests will be stored in the queue.
* If queue becomes full, request will be executed on the calling thread.
*
* There is not a perfect combination of these two parameters, it will depend
* on your particular environment. Experiment.
*
*/
public void setMaxAsyncThreads(int maxAsyncThreads) {
this.maxAsyncThreads = maxAsyncThreads;
}
/**
* Sets MaxAsyncThreads property and returns current MarketplaceWebServiceConfig
*
* @param maxAsyncThreads Max number of threads to spawn for async operation.
* Note, this parameter works in conjuction with MaxAsyncQueueSize. When
* Max number of threads is reached, requests will be stored in the queue.
*
* If queue becomes full, request will be executed on the calling thread.
*
* There is not a perfect combination of these two parameters, it will depend
* on your particular environment. Experiment.
*
*
* @return MarketplaceWebServiceConfig
*/
public MarketplaceWebServiceConfig withMaxAsyncThreads(int maxAsyncThreads) {
setMaxAsyncThreads(maxAsyncThreads);
return this;
}
/**
* Checks if MaxAsyncThreads property is set
*
* @return true if MaxAsyncThreads property is set
*/
public boolean isSetMaxAsyncThreads() {
return this.maxAsyncThreads > 0;
}
/**
* Gets MaxAsyncQueueSize property
*
* @return Max number of requests to be queued when max number of threads is
* reached and all those threads are busy processing.
*/
public int getMaxAsyncQueueSize() {
return maxAsyncQueueSize;
}
/**
* Checks if MaxAsyncQueueSize property is set
*
* @return true if MaxAsyncQueueSize property is set
*/
public boolean isSetMaxAsyncQueueSize() {
return this.maxAsyncQueueSize > 0;
}
/**
* Sets MaxAsyncQueueSize property
*
* @param maxAsyncQueueSize Max number of requests to queue when max number of
* threads is reached, and all threads are busy processing.
*
* Note, this parameter works in conjuction with MaxAsyncThreads.
*
* When max number of threads is reached and queue is full, request will
* be executed on the calling thread.
*
* There is not a perfect combination of these two parameters, it will depend
* on your particular environment. Experiment.
*
*/
public void setMaxAsyncQueueSize(int maxAsyncQueueSize) {
this.maxAsyncQueueSize = maxAsyncQueueSize;
}
/**
* Sets MaxAsyncQueueSize property and returns current MarketplaceWebServiceConfig
*
* @param maxAsyncQueueSize Max number of requests to queue when max number of
* threads is reached, and all threads are busy processing.
*
* Note, this parameter works in conjuction with MaxAsyncThreads.
*
* When max number of threads is reached and queue is full, request will
* be executed on the calling thread.
*
* There is not a perfect combination of these two parameters, it will depend
* on your particular environment. Experiment.
*
*/
public MarketplaceWebServiceConfig withMaxAsyncQueueSize(int maxAsyncQueueSize) {
setMaxAsyncQueueSize(maxAsyncQueueSize);
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy