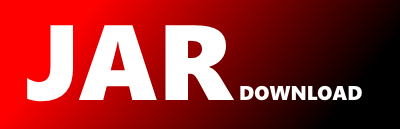
net.dreceiptx.receipt.allowanceCharge.ReceiptAllowanceCharge Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of drx-sdk-java Show documentation
Show all versions of drx-sdk-java Show documentation
Digital Receipt Exchange Java SDK
/*
* Copyright 2016 Digital Receipt Exchange Limited
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.dreceiptx.receipt.allowanceCharge;
import net.dreceiptx.receipt.tax.Tax;
import net.dreceiptx.receipt.tax.TaxCode;
import com.google.gson.annotations.SerializedName;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
public class ReceiptAllowanceCharge {
//TODO: ID is never used
private transient int Id;
@SerializedName("allowanceOrChargeType")
private AllowanceOrChargeType _allowanceOrChargeType;
@SerializedName("allowanceChargeType")
private AllowanceChargeType _allowanceChargeType;
@SerializedName("settlementType")
private SettlementType _settlementType;
@SerializedName("baseAmount")
private BigDecimal _amount;
@SerializedName("allowanceChargeDescription")
private String _description;
@SerializedName("leviedDutyFeeTax")
private List _taxes = new ArrayList();;
//TODO: If we keep it this should not be a double
public double getId() {
return Id;
}
//TODO: Consider changing to getSettlementType
public SettlementType getType() {
return _settlementType;
}
public BigDecimal getSubTotal() {
return _amount;
}
public String getDescription() {
return _description;
}
public BigDecimal getNetTotal() {
return _amount;
}
public BigDecimal getTotal() {
return _amount.add(this.getTaxesTotal());
}
public boolean hasTaxes() {
return !_taxes.isEmpty();
}
public BigDecimal getTaxesTotal() {
BigDecimal totalTaxes = new BigDecimal(0.0);
for (Tax tax : _taxes) {
totalTaxes = totalTaxes.add(tax.getTaxTotal());
}
return totalTaxes;
}
// TODO: Consider renaming to calculateTaxesTotal
/**
* Calculates the TaxesTotal for the given TaxCode
* @param taxCode to filter the taxes
* @return TotalTaxes for the given TaxCode if they exist otherwise 0
*/
public BigDecimal getTaxesTotal(TaxCode taxCode) {
BigDecimal totalTaxes = new BigDecimal(0.0);
for (Tax tax : _taxes) {
if(tax.getTaxCode().equals(taxCode)){
totalTaxes = totalTaxes.add(tax.getTaxTotal());
}
}
return totalTaxes;
}
public List getTaxes() {
return _taxes;
}
public boolean isCharge(){
return (_allowanceOrChargeType == AllowanceOrChargeType.CHARGE);
}
public boolean isAllowance(){
return (_allowanceOrChargeType == AllowanceOrChargeType.ALLOWANCE);
}
public static ReceiptAllowanceCharge Tip(double amount, String description){
ReceiptAllowanceCharge receiptAllowanceCharge = new ReceiptAllowanceCharge();
receiptAllowanceCharge._amount = BigDecimal.valueOf(amount);
receiptAllowanceCharge._description = description;
receiptAllowanceCharge._allowanceOrChargeType = AllowanceOrChargeType.CHARGE;
receiptAllowanceCharge._allowanceChargeType = AllowanceChargeType.CHARGE_TO_BE_PAID_BY_CUSTOMER;
receiptAllowanceCharge._settlementType = SettlementType.TIP;
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge Tip(double amount, String description, Tax tax){
ReceiptAllowanceCharge receiptAllowanceCharge = ReceiptAllowanceCharge.Tip(amount, description);
receiptAllowanceCharge._taxes.add(tax);
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge DeliveryFee(double amount, String description){
ReceiptAllowanceCharge receiptAllowanceCharge = new ReceiptAllowanceCharge();
receiptAllowanceCharge._amount = BigDecimal.valueOf(amount);
receiptAllowanceCharge._description = description;
receiptAllowanceCharge._allowanceOrChargeType = AllowanceOrChargeType.CHARGE;
receiptAllowanceCharge._allowanceChargeType = AllowanceChargeType.CHARGE_TO_BE_PAID_BY_CUSTOMER;
receiptAllowanceCharge._settlementType = SettlementType.DeliveryFee;
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge DeliveryFee(double amount, String description, Tax tax){
ReceiptAllowanceCharge receiptAllowanceCharge = ReceiptAllowanceCharge.DeliveryFee(amount, description);
receiptAllowanceCharge._taxes.add(tax);
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge FreightFee(double amount, String description){
ReceiptAllowanceCharge receiptAllowanceCharge = new ReceiptAllowanceCharge();
receiptAllowanceCharge._amount = BigDecimal.valueOf(amount);
receiptAllowanceCharge._description = description;
receiptAllowanceCharge._allowanceOrChargeType = AllowanceOrChargeType.CHARGE;
receiptAllowanceCharge._allowanceChargeType = AllowanceChargeType.CHARGE_TO_BE_PAID_BY_CUSTOMER;
receiptAllowanceCharge._settlementType = SettlementType.FreightFee;
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge FreightFee(double amount, String description, Tax tax){
ReceiptAllowanceCharge receiptAllowanceCharge = ReceiptAllowanceCharge.FreightFee(amount, description);
receiptAllowanceCharge._taxes.add(tax);
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge PackagingFee(double amount, String description){
ReceiptAllowanceCharge receiptAllowanceCharge = new ReceiptAllowanceCharge();
receiptAllowanceCharge._amount = BigDecimal.valueOf(amount);
receiptAllowanceCharge._description = description;
receiptAllowanceCharge._allowanceOrChargeType = AllowanceOrChargeType.CHARGE;
receiptAllowanceCharge._allowanceChargeType = AllowanceChargeType.CHARGE_TO_BE_PAID_BY_CUSTOMER;
receiptAllowanceCharge._settlementType = SettlementType.PackagingFee;
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge PackagingFee(double amount, String description, Tax tax){
ReceiptAllowanceCharge receiptAllowanceCharge = ReceiptAllowanceCharge.PackagingFee(amount, description);
receiptAllowanceCharge._taxes.add(tax);
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge ProcessingFee(double amount, String description){
ReceiptAllowanceCharge receiptAllowanceCharge = new ReceiptAllowanceCharge();
receiptAllowanceCharge._amount = BigDecimal.valueOf(amount);
receiptAllowanceCharge._description = description;
receiptAllowanceCharge._allowanceOrChargeType = AllowanceOrChargeType.CHARGE;
receiptAllowanceCharge._allowanceChargeType = AllowanceChargeType.CHARGE_TO_BE_PAID_BY_CUSTOMER;
receiptAllowanceCharge._settlementType = SettlementType.ProcessingFee;
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge ProcessingFee(double amount, String description, Tax tax){
ReceiptAllowanceCharge receiptAllowanceCharge = ReceiptAllowanceCharge.ProcessingFee(amount, description);
receiptAllowanceCharge._taxes.add(tax);
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge BookingFee(double amount, String description){
ReceiptAllowanceCharge receiptAllowanceCharge = new ReceiptAllowanceCharge();
receiptAllowanceCharge._amount = BigDecimal.valueOf(amount);
receiptAllowanceCharge._description = description;
receiptAllowanceCharge._allowanceOrChargeType = AllowanceOrChargeType.CHARGE;
receiptAllowanceCharge._allowanceChargeType = AllowanceChargeType.CHARGE_TO_BE_PAID_BY_CUSTOMER;
receiptAllowanceCharge._settlementType = SettlementType.BookingFee;
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge BookingFee(double amount, String description, Tax tax){
ReceiptAllowanceCharge receiptAllowanceCharge = ReceiptAllowanceCharge.BookingFee(amount, description);
receiptAllowanceCharge._taxes.add(tax);
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge GeneralDiscount(double amount, String description){
ReceiptAllowanceCharge receiptAllowanceCharge = new ReceiptAllowanceCharge();
receiptAllowanceCharge._amount = BigDecimal.valueOf(amount);
receiptAllowanceCharge._description = description;
receiptAllowanceCharge._allowanceOrChargeType = AllowanceOrChargeType.ALLOWANCE;
receiptAllowanceCharge._allowanceChargeType = AllowanceChargeType.CREDIT_CUSTOMER_ACCOUNT;
receiptAllowanceCharge._settlementType = SettlementType.GeneralDiscount;
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge GeneralDiscount(double amount, String description, Tax tax){
ReceiptAllowanceCharge receiptAllowanceCharge = ReceiptAllowanceCharge.GeneralDiscount(amount, description);
receiptAllowanceCharge._taxes.add(tax);
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge MultiBuyDiscount(double amount, String description){
ReceiptAllowanceCharge receiptAllowanceCharge = new ReceiptAllowanceCharge();
receiptAllowanceCharge._amount = BigDecimal.valueOf(amount);
receiptAllowanceCharge._description = description;
receiptAllowanceCharge._allowanceOrChargeType = AllowanceOrChargeType.ALLOWANCE;
receiptAllowanceCharge._allowanceChargeType = AllowanceChargeType.CREDIT_CUSTOMER_ACCOUNT;
receiptAllowanceCharge._settlementType = SettlementType.MultiBuyDiscount;
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge MultiBuyDiscount(double amount, String description, Tax tax){
ReceiptAllowanceCharge receiptAllowanceCharge = ReceiptAllowanceCharge.MultiBuyDiscount(amount, description);
receiptAllowanceCharge._taxes.add(tax);
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge ServiceFee(double amount, String description){
ReceiptAllowanceCharge receiptAllowanceCharge = new ReceiptAllowanceCharge();
receiptAllowanceCharge._amount = BigDecimal.valueOf(amount);
receiptAllowanceCharge._description = description;
receiptAllowanceCharge._allowanceOrChargeType = AllowanceOrChargeType.CHARGE;
receiptAllowanceCharge._allowanceChargeType = AllowanceChargeType.CHARGE_TO_BE_PAID_BY_CUSTOMER;
receiptAllowanceCharge._settlementType = SettlementType.ServiceFee;
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge ServiceFee(double amount, String description, Tax tax){
ReceiptAllowanceCharge receiptAllowanceCharge = ReceiptAllowanceCharge.ServiceFee(amount, description);
receiptAllowanceCharge._taxes.add(tax);
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge AdminFee(double amount, String description){
ReceiptAllowanceCharge receiptAllowanceCharge = new ReceiptAllowanceCharge();
receiptAllowanceCharge._amount = BigDecimal.valueOf(amount);
receiptAllowanceCharge._description = description;
receiptAllowanceCharge._allowanceOrChargeType = AllowanceOrChargeType.CHARGE;
receiptAllowanceCharge._allowanceChargeType = AllowanceChargeType.CHARGE_TO_BE_PAID_BY_CUSTOMER;
receiptAllowanceCharge._settlementType = SettlementType.AdminFee;
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge AdminFee(double amount, String description, Tax tax){
ReceiptAllowanceCharge receiptAllowanceCharge = ReceiptAllowanceCharge.AdminFee(amount, description);
receiptAllowanceCharge._taxes.add(tax);
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge AmendmentFee(double amount, String description){
ReceiptAllowanceCharge receiptAllowanceCharge = new ReceiptAllowanceCharge();
receiptAllowanceCharge._amount = BigDecimal.valueOf(amount);
receiptAllowanceCharge._description = description;
receiptAllowanceCharge._allowanceOrChargeType = AllowanceOrChargeType.CHARGE;
receiptAllowanceCharge._allowanceChargeType = AllowanceChargeType.CHARGE_TO_BE_PAID_BY_CUSTOMER;
receiptAllowanceCharge._settlementType = SettlementType.AmendmentFee;
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge AmendmentFee(double amount, String description, Tax tax){
ReceiptAllowanceCharge receiptAllowanceCharge = ReceiptAllowanceCharge.AmendmentFee(amount, description);
receiptAllowanceCharge._taxes.add(tax);
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge HandlingFee(double amount, String description){
ReceiptAllowanceCharge receiptAllowanceCharge = new ReceiptAllowanceCharge();
receiptAllowanceCharge._amount = BigDecimal.valueOf(amount);
receiptAllowanceCharge._description = description;
receiptAllowanceCharge._allowanceOrChargeType = AllowanceOrChargeType.CHARGE;
receiptAllowanceCharge._allowanceChargeType = AllowanceChargeType.CHARGE_TO_BE_PAID_BY_CUSTOMER;
receiptAllowanceCharge._settlementType = SettlementType.HandlingFee;
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge HandlingFee(double amount, String description, Tax tax){
ReceiptAllowanceCharge receiptAllowanceCharge = ReceiptAllowanceCharge.HandlingFee(amount, description);
receiptAllowanceCharge._taxes.add(tax);
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge ReturnOrCancellationFee(double amount, String description){
ReceiptAllowanceCharge receiptAllowanceCharge = new ReceiptAllowanceCharge();
receiptAllowanceCharge._amount = BigDecimal.valueOf(amount);
receiptAllowanceCharge._description = description;
receiptAllowanceCharge._allowanceOrChargeType = AllowanceOrChargeType.CHARGE;
receiptAllowanceCharge._allowanceChargeType = AllowanceChargeType.CHARGE_TO_BE_PAID_BY_CUSTOMER;
receiptAllowanceCharge._settlementType = SettlementType.ReturnOrCancellationFee;
return receiptAllowanceCharge;
}
public static ReceiptAllowanceCharge ReturnOrCancellationFee(double amount, String description, Tax tax){
ReceiptAllowanceCharge receiptAllowanceCharge = ReceiptAllowanceCharge.ReturnOrCancellationFee(amount, description);
receiptAllowanceCharge._taxes.add(tax);
return receiptAllowanceCharge;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy