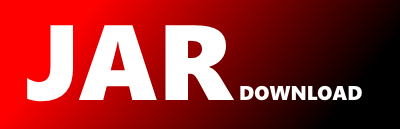
net.dreceiptx.client.DRXClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of drx-sdk-java Show documentation
Show all versions of drx-sdk-java Show documentation
Digital Receipt Exchange Java SDK
/*
* Copyright 2016 Digital Receipt Exchange Limited
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.dreceiptx.client;
import net.dreceiptx.SDK;
import net.dreceiptx.receipt.DigitalReceipt;
import net.dreceiptx.receipt.DigitalReceiptGenerator;
import net.dreceiptx.config.ConfigManager;
import net.dreceiptx.receipt.merchant.Merchant;
import net.dreceiptx.receipt.serialization.json.NewUsersSerializer;
import net.dreceiptx.users.NewUser;
import net.dreceiptx.users.NewUserRegistrationResult;
import net.dreceiptx.users.User;
import net.dreceiptx.users.UserIdentifierType;
import net.dreceiptx.users.Users;
import net.dreceiptx.client.exception.ExchangeClientException;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
import org.apache.commons.codec.binary.Base64;
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import java.io.*;
import java.net.*;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class DRXClient implements ExchangeClient {
private static final int BUFFER_SIZE = 4096;
private final ConfigManager _configManager;
private static final String CONTENT_TYPE_JSON = "application/json";
private static final String CONTENT_TYPE_XML = "application/xml";
private static final String CONTENT_TYPE_PDF = "application/pdf";
private String USER_AGENT;
private final EnvironmentType _environmentType;
private String _exchangeProtocol = "https";
private final String _exchangeHostname;
private final String _requesterId;
private final String _receiptVersion;
private String _directoryProtocol = "https";
private final String _directoryHostname;
private final String _userVersion;
private final String _downloadDirectory;
private int _responseErrorCode = 500;
private String _responseErrorMessage = "Unknown Error";
/**
* Creates instance of ExchangeClient using the given ConfigManager
*
* @param configManager the ConfigManager to be used by the ExchangeClient
* @throws ExchangeClientException
*/
public DRXClient(ConfigManager configManager) throws ExchangeClientException {
USER_AGENT = "dRx Java SDK/"+ SDK.VERSION +" Receipt/" + SDK.RECEIPT_VERSION_COMPATIBILITY;
_configManager = configManager;
_exchangeHostname = validateConfigOption("exchange.hostname");
_directoryHostname = validateConfigOption("directory.hostname");
_requesterId = validateConfigOption("api.requesterId");
_receiptVersion = validateConfigOption("receipt.version");
_userVersion = validateConfigOption("user.version");
_downloadDirectory = validateConfigOption("download.directory");
if(_configManager.exists("exchange.protocol")){
_environmentType = EnvironmentType.valueOf(_configManager.getConfigValue("environment.type"));
}else{
_environmentType = EnvironmentType.PROD;
}
if(_configManager.exists("exchange.protocol")){
_exchangeProtocol = _configManager.getConfigValue("exchange.protocol");
}
if(_configManager.exists("directory.protocol")){
_directoryProtocol = _configManager.getConfigValue("directory.protocol");
}
switch (_environmentType) {
case PROD:
_exchangeProtocol = "https";
_directoryProtocol = "https";
break;
}
}
@Override
public User searchUser(UserIdentifierType identifierType, String identifier) throws ExchangeClientException {
String encodedIdentifier;
try{
encodedIdentifier = URLEncoder.encode(identifier, "UTF-8");
}catch (UnsupportedEncodingException ex){
encodedIdentifier = identifier;
}
UriParameters uriParameters = new UriParameters();
uriParameters.add("idtype", identifierType.getValue());
return searchUser(encodedIdentifier, uriParameters);
}
private User searchUser(String encodedIdentifier, UriParameters uriParameters) throws ExchangeClientException {
try {
HttpURLConnection connection = createConnection(_directoryProtocol, _directoryHostname,
"/user/"+encodedIdentifier, CONTENT_TYPE_JSON, "GET", _userVersion, uriParameters);
this.openConnection(connection);
int responseCode = connection.getResponseCode();
if (responseCode == HttpCodes.HTTP_200_OK || responseCode == HttpCodes.HTTP_400_BAD_REQUEST) {
JsonObject exchangeResponse = getResponseJsonObject(connection);
if (exchangeResponse.get("success").getAsBoolean()) {
User user = new User();
JsonObject responseData = exchangeResponse.get("responseData").getAsJsonObject();
user.setGUID(responseData.get("guid").getAsString());
user.setRMS(responseData.get("rms").getAsString());
connection.disconnect();
return user;
} else {
connection.disconnect();
throw new ExchangeClientException(exchangeResponse.get("code").getAsInt(), exchangeResponse.get("exceptionMessage").getAsString());
}
} else if (responseCode == HttpCodes.HTTP_404_NOTFOUND) {
connection.disconnect();
throw new ExchangeClientException(404, "The exchange host could not be found or is currently unavailable, please check ConfigManager setting and ensure they are correct.");
}else if (responseCode == HttpCodes.HTTP_401_UNAUTHORIZED) {
loadErrorResponseJsonObject(connection);
connection.disconnect();
throw new ExchangeClientException(_responseErrorCode, _responseErrorMessage);
} else {
this.manageUnexpectedResponse(connection);
throw new ExchangeClientException(_responseErrorCode, _responseErrorMessage);
}
}catch (SocketTimeoutException te){
throw new ExchangeClientException(500, "The connection to the exchange timed out and did not receive a response", te);
}catch (ExchangeClientException dRxE) {
throw dRxE;
}catch (Exception e) {
throw new ExchangeClientException(500, e.toString(), e);
}
}
@Override
public Users searchUsers(UserIdentifierType identifierType, ArrayList userIdentifiers) throws ExchangeClientException {
UriParameters uriParameters = new UriParameters();
//TODO: Why lower case here?
uriParameters.add("idtype", identifierType.getValue().toLowerCase());
StringBuilder userIdentifiersParam = new StringBuilder();
boolean firstIteration = true;
for (String userIdentifier : userIdentifiers) {
if(!firstIteration){
userIdentifiersParam.append(";");
}
userIdentifiersParam.append(userIdentifier);
firstIteration = false;
}
uriParameters.add("identifiers", userIdentifiersParam.toString());
Users users = new Users();
try {
HttpURLConnection connection = createConnection(_directoryProtocol, _directoryHostname,
"/user", CONTENT_TYPE_JSON, "GET", _userVersion, uriParameters);
this.openConnection(connection);
int responseCode = connection.getResponseCode();
if (responseCode == HttpCodes.HTTP_200_OK || responseCode == HttpCodes.HTTP_400_BAD_REQUEST) {
JsonObject exchangeResponse = getResponseJsonObject(connection);
if (exchangeResponse.get("success").getAsBoolean()) {
JsonObject responseData = exchangeResponse.get("responseData").getAsJsonObject();
JsonObject userIdentifiersObject = responseData.get("userIdentifiers").getAsJsonObject();
for (Map.Entry entry : userIdentifiersObject.entrySet()) {
User user;
if (!entry.getValue().isJsonNull()) {
JsonObject userIdentifierObject = userIdentifiersObject.getAsJsonObject(entry.getKey());
user = new User();
user.setIdentifierType(identifierType);
user.setIdentifier(entry.getKey());
user.setGUID(userIdentifierObject.get("guid").getAsString());
user.setRMS(userIdentifierObject.get("rms").getAsString());
}else{
user = new User();
user.setIdentifierType(identifierType);
user.setIdentifier(entry.getKey());
}
users.add(entry.getKey(), user);
}
connection.disconnect();
return users;
} else {
throw new ExchangeClientException(exchangeResponse.get("code").getAsInt(), exchangeResponse.get("exceptionMessage").getAsString());
}
} else if (responseCode == HttpCodes.HTTP_404_NOTFOUND) {
connection.disconnect();
throw new ExchangeClientException(404, "The exchange host could not be found or is currently unavailable, please check ConfigManager setting and ensure they are correct.");
} else if (responseCode == HttpCodes.HTTP_401_UNAUTHORIZED) {
loadErrorResponseJsonObject(connection);
connection.disconnect();
throw new ExchangeClientException(_responseErrorCode, _responseErrorMessage);
} else {
this.manageUnexpectedResponse(connection);
connection.disconnect();
throw new ExchangeClientException(_responseErrorCode, _responseErrorMessage);
}
}catch(ConnectException ce){
throw new ExchangeClientException(500, "There was a connection exception, please ensure internet connectivity and exchange host settings", ce);
}catch (SocketTimeoutException te){
throw new ExchangeClientException(500, "The connection to the exchange timed out and did not receive a response", te);
}catch (ExchangeClientException dRxE) {
throw dRxE;
}catch (Exception e) {
throw new ExchangeClientException(500, e.toString(), e);
}
}
@Override
public String sendReceipt(DigitalReceiptGenerator receipt) throws ExchangeClientException {
try {
HttpURLConnection connection = createConnection(_exchangeProtocol, _exchangeHostname, "/receipt", CONTENT_TYPE_JSON,
"POST", _receiptVersion);
this.setConnectionContent(connection,receipt.encodeJson());
this.openConnection(connection);
int responseCode = connection.getResponseCode();
if (responseCode == HttpCodes.HTTP_201_CREATED || responseCode == HttpCodes.HTTP_400_BAD_REQUEST) {
JsonObject exchangeResponse = getResponseJsonObject(connection);
if (exchangeResponse.get("success").getAsBoolean()) {
JsonObject responseData = exchangeResponse.get("responseData").getAsJsonObject();
return responseData.get("receiptId").getAsString();
} else {
throw new ExchangeClientException(exchangeResponse.get("code").getAsInt(), exchangeResponse.get("exceptionMessage").getAsString());
}
} else if (responseCode == HttpCodes.HTTP_401_UNAUTHORIZED) {
loadErrorResponseJsonObject(connection);
connection.disconnect();
throw new ExchangeClientException(_responseErrorCode, _responseErrorMessage);
} else if (responseCode == HttpCodes.HTTP_404_NOTFOUND) {
connection.disconnect();
throw new ExchangeClientException(404, "The exchange host could not be found or is currently unavailable, please check ConfigManager setting and ensure they are correct.");
}else {
this.manageUnexpectedResponse(connection);
connection.disconnect();
throw new ExchangeClientException(_responseErrorCode, _responseErrorMessage);
}
}catch(ConnectException ce){
throw new ExchangeClientException(500, "There was a connection exception, please ensure internet connectivity and exchange host settings", ce);
}catch (SocketTimeoutException te){
throw new ExchangeClientException(500, "The connection to the exchange timed out and did not receive a response", te);
}catch (ExchangeClientException dRxE) {
throw dRxE;
}catch (Exception e) {
throw new ExchangeClientException(500, e.toString(), e);
}
}
@Override
public DigitalReceipt lookupReceipt(String receiptId) throws ExchangeClientException {
try {
HttpURLConnection connection = createConnection(_exchangeProtocol, _exchangeHostname, "/receipt/" + receiptId, CONTENT_TYPE_JSON, "GET", _receiptVersion);
this.openConnection(connection);
int responseCode = connection.getResponseCode();
if (responseCode == HttpCodes.HTTP_200_OK) {
String response = getResponseString(connection);
connection.disconnect();
return new DigitalReceipt(response);
} else if (responseCode == HttpCodes.HTTP_404_NOTFOUND) {
connection.disconnect();
throw new ExchangeClientException(404, "The exchange host could not be found or is currently unavailable, please check ConfigManager setting and ensure they are correct.");
} else if (responseCode == HttpCodes.HTTP_400_BAD_REQUEST || responseCode == HttpCodes.HTTP_401_UNAUTHORIZED) {
loadErrorResponseJsonObject(connection);
connection.disconnect();
throw new ExchangeClientException(_responseErrorCode, _responseErrorMessage);
} else {
this.manageUnexpectedResponse(connection);
connection.disconnect();
throw new ExchangeClientException(_responseErrorCode, _responseErrorMessage);
}
}catch(ConnectException ce){
throw new ExchangeClientException(500, "There was a connection exception, please ensure internet connectivity and exchange host settings", ce);
}catch (SocketTimeoutException te){
throw new ExchangeClientException(500, "The connection to the exchange timed out and did not receive a response", te);
}catch (Exception e) {
throw new ExchangeClientException(500, e.toString(), e);
}
}
//TODO: Add download to S3 Bucket function
//TODO: Allow to set download folder dynamically
public boolean downloadReceiptPDF(String receiptId) throws ExchangeClientException {
try {
HttpURLConnection connection = createConnection(_exchangeProtocol, _exchangeHostname, "/receipt/" + receiptId, CONTENT_TYPE_PDF, "GET", _receiptVersion);
this.openConnection(connection);
int responseCode = connection.getResponseCode();
if (responseCode == HttpCodes.HTTP_200_OK) {
InputStream pdfInputStream = connection.getInputStream();
String saveFilePath = _downloadDirectory + File.separator + receiptId + ".pdf";
// opens an output stream to save into file
FileOutputStream pdfOutputStream = new FileOutputStream(saveFilePath);
int bytesRead;
byte[] buffer = new byte[BUFFER_SIZE];
while ((bytesRead = pdfInputStream.read(buffer)) != -1) {
pdfOutputStream.write(buffer, 0, bytesRead);
}
pdfOutputStream.close();
pdfInputStream.close();
return true;
} else if (responseCode == HttpCodes.HTTP_404_NOTFOUND) {
connection.disconnect();
throw new ExchangeClientException(404, "The exchange host could not be found or is currently unavailable, please check ConfigManager setting and ensure they are correct.");
} else if (responseCode == HttpCodes.HTTP_400_BAD_REQUEST || responseCode == HttpCodes.HTTP_401_UNAUTHORIZED) {
loadErrorResponseJsonObject(connection);
connection.disconnect();
throw new ExchangeClientException(_responseErrorCode, _responseErrorMessage);
} else {
this.manageUnexpectedResponse(connection);
connection.disconnect();
throw new ExchangeClientException(_responseErrorCode, _responseErrorMessage);
}
}catch(ConnectException ce){
throw new ExchangeClientException(500, "There was a connection exception, please ensure internet connectivity and exchange host settings", ce);
}catch (SocketTimeoutException te){
throw new ExchangeClientException(500, "The connection to the exchange timed out and did not receive a response", te);
}catch (ExchangeClientException dRxE) {
throw dRxE;
}catch (Exception e) {
throw new ExchangeClientException(500, e.toString(), e);
}
}
public NewUserRegistrationResult registerNewUser(NewUser newUser) throws ExchangeClientException {
List _newUserCollection = new ArrayList<>();
_newUserCollection.add(newUser);
Map _newUserRegistrationResponse = this.registerNewUser(_newUserCollection);
return _newUserRegistrationResponse.get(newUser.getEmail());
}
public Map registerNewUser(List newUsers) throws ExchangeClientException {
Map _newUserRegistrationResponse = new HashMap<>();
try {
Gson gson = new GsonBuilder()
.setDateFormat("yyyy-MM-dd'T'HH:mm:ssZ")
.registerTypeAdapter(newUsers.getClass(), new NewUsersSerializer())
.create();
String newUserRegistrationJson = gson.toJson(newUsers);
HttpURLConnection connection = createConnection(_exchangeProtocol, _exchangeHostname, "/user", CONTENT_TYPE_JSON, "POST", _userVersion);
this.setConnectionContent(connection,newUserRegistrationJson);
this.openConnection(connection);
int responseCode = connection.getResponseCode();
if (responseCode == HttpCodes.HTTP_201_CREATED || responseCode == HttpCodes.HTTP_400_BAD_REQUEST) {
JsonObject exchangeResponse = getResponseJsonObject(connection);
if (exchangeResponse.get("success").getAsBoolean()) {
JsonObject responseData = exchangeResponse.get("responseData").getAsJsonObject();
JsonObject usersObject = responseData.get("users").getAsJsonObject();
for (Map.Entry entry : usersObject.entrySet()) {
NewUserRegistrationResult newUserRegistrationResult = new NewUserRegistrationResult();
if(!entry.getValue().isJsonNull()){
JsonObject userRegistrationObject = usersObject.getAsJsonObject(entry.getKey());
if(userRegistrationObject.get("success").getAsBoolean()){
newUserRegistrationResult.setUserGUID(userRegistrationObject.get("guid").getAsString());
}else{
newUserRegistrationResult.setException(userRegistrationObject.get("code").getAsInt(),userRegistrationObject.get("exception").getAsString());
}
}
_newUserRegistrationResponse.put(entry.getKey(), newUserRegistrationResult);
}
} else {
throw new ExchangeClientException(exchangeResponse.get("code").getAsInt(), exchangeResponse.get("exceptionMessage").getAsString());
}
} else if (responseCode == HttpCodes.HTTP_401_UNAUTHORIZED) {
loadErrorResponseJsonObject(connection);
connection.disconnect();
throw new ExchangeClientException(_responseErrorCode, _responseErrorMessage);
} else if (responseCode == HttpCodes.HTTP_404_NOTFOUND) {
connection.disconnect();
throw new ExchangeClientException(_responseErrorCode, "Exchange could not be found, ensure internet connection or valid URL");
}else {
this.manageUnexpectedResponse(connection);
connection.disconnect();
throw new ExchangeClientException(_responseErrorCode, _responseErrorMessage);
}
}catch(ConnectException ce){
throw new ExchangeClientException(500, "There was a connection exception, please ensure internet connectivity and exchange host settings", ce);
}catch (SocketTimeoutException te){
throw new ExchangeClientException(500, "The connection to the exchange timed out and did not receive a response", te);
}catch (ExchangeClientException dRxE) {
throw dRxE;
}catch (Exception e) {
throw new ExchangeClientException(500, e.toString(), e);
}
return _newUserRegistrationResponse;
}
public Merchant lookupMerchant(String Id) throws ExchangeClientException {
try {
String merchantLocationHostname = "https://cdn.dreceiptx.net/merchant/location/";
if(!_environmentType.equals(EnvironmentType.PROD)){
merchantLocationHostname = "https://cdn.dreceiptx.net/uat/merchant/location/";
}
URL merchantRequest = new URL(merchantLocationHostname + Id + "/info.json");
HttpURLConnection connection = (HttpURLConnection) merchantRequest.openConnection();
int responseCode = connection.getResponseCode();
if (responseCode == HttpCodes.HTTP_200_OK) {
BufferedReader merchantRequestConnectionReader = new BufferedReader(new InputStreamReader(
connection.getInputStream()));
StringBuilder response = new StringBuilder();
String line;
while ((line = merchantRequestConnectionReader.readLine()) != null) {
response.append(line);
}
merchantRequestConnectionReader.close();
Gson gson = new Gson();
Merchant merchant = gson.fromJson(response.toString(), Merchant.class);
merchant.setMerchantLocationHostname(merchantLocationHostname);
return merchant;
}
else{
throw new ExchangeClientException(404, "Unknown merchant Id, please supply a valid ");
}
}catch(ConnectException ce){
throw new ExchangeClientException(500, "There was a connection exception, please ensure internet connectivity and exchange host settings", ce);
}catch (SocketTimeoutException te){
throw new ExchangeClientException(500, "The connection to the exchange timed out and did not receive a response", te);
}catch (ExchangeClientException dRxE) {
throw dRxE;
}catch (Exception e) {
throw new ExchangeClientException(500, e.toString(), e);
}
}
private String createAuthKey(String timestamp) throws NoSuchAlgorithmException, InvalidKeyException, UnsupportedEncodingException {
String key = _configManager.getConfigValue("api.key") + ":" + String.valueOf(timestamp) + ":" + _configManager.getConfigValue("api.requesterId");
Mac sha256_HMAC = Mac.getInstance("HmacSHA256");
SecretKeySpec secret_key = new SecretKeySpec(_configManager.getConfigValue("api.secret").getBytes(), "HmacSHA256");
sha256_HMAC.init(secret_key);
String hash = Base64.encodeBase64String(sha256_HMAC.doFinal(key.getBytes()));
return "DRX " + hash;
}
private HttpURLConnection createConnection(String protocol, String hostname, String uri, String contentType,
String requestMethod, String requestVersion, UriParameters parameters)
throws IOException, InvalidKeyException, NoSuchAlgorithmException {
if(parameters != null) {
StringBuilder parameterString = new StringBuilder();
boolean firstIteration = true;
for (Map.Entry param : parameters.getEntrySet()) {
if(firstIteration){
parameterString.append("?");
firstIteration = false;
}else{
parameterString.append("&");
}
parameterString.append(param.getKey()).append("=").append(param.getValue());
}
uri = uri+parameterString.toString();
}
URL url = new URL(protocol + "://" + hostname + uri);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod(requestMethod);
connection.setDoOutput(true);
connection.setDoInput(true);
if(contentType != null) {
connection.setRequestProperty("Content-Type", contentType);
}
String timestamp = String.valueOf(System.currentTimeMillis());
connection.setRequestProperty("Authorization", createAuthKey(timestamp));
connection.setRequestProperty("x-drx-timestamp", timestamp);
connection.setRequestProperty("x-drx-requester", _requesterId);
connection.setRequestProperty("x-drx-version", requestVersion);
connection.setRequestProperty("User-Agent", USER_AGENT);
connection.setConnectTimeout(5000);
connection.setReadTimeout(300000);
return connection;
}
private HttpURLConnection createConnection(String protocol, String hostname, String uri, String contentType, String requestMethod,
String requestVersion) throws IOException, InvalidKeyException, NoSuchAlgorithmException {
return this.createConnection(protocol, hostname, uri, contentType, requestMethod, requestVersion, null);
}
private void setConnectionContent(HttpURLConnection connection, String content) throws IOException, ExchangeClientException {
if(SDK.DEBUG){
System.out.println("REQUEST CONTENT: "+content);
}
OutputStream os = connection.getOutputStream();
os.write(content.getBytes());
os.flush();
}
private HttpURLConnection openConnection(URL url) throws IOException, ExchangeClientException {
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
if(SDK.DEBUG){
System.out.println("URL: "+connection.getURL().toString());
System.out.println("METHOD: "+connection.getRequestMethod());
}
return connection;
}
private void openConnection(HttpURLConnection connection) throws IOException, ExchangeClientException {
connection.connect();
if(SDK.DEBUG){
System.out.println("URL: "+connection.getURL().toString());
System.out.println("METHOD: "+connection.getRequestMethod());
}
}
private void manageUnexpectedResponse(HttpURLConnection connection) throws IOException, ExchangeClientException {
_responseErrorCode = connection.getResponseCode();
_responseErrorMessage = connection.getResponseMessage();
if(SDK.DEBUG){
System.out.println("RESPONSE CODE: "+_responseErrorCode);
System.out.println("RESPONSE REASON: "+_responseErrorMessage);
System.out.println("RESPONSE CONTENT: "+this.getResponseString(connection));
}
}
private String getResponseString(HttpURLConnection connection) throws IOException, ExchangeClientException {
InputStream is;
if (connection.getResponseCode() >= 400) {
is = connection.getErrorStream();
} else {
is = connection.getInputStream();
}
if(is != null){
try (BufferedReader br = new BufferedReader(new InputStreamReader(is))) {
StringBuilder sb = new StringBuilder();
String line;
while ((line = br.readLine()) != null) {
sb.append(line).append("\n");
}
if(SDK.DEBUG){
System.out.println("RESPONSE CONTENT: " +sb.toString());
}
return sb.toString();
} catch (Exception e) {
throw new ExchangeClientException(500, e.toString(), e);
}
}else{
if(SDK.DEBUG){
System.out.println("RESPONSE CONTENT: N/A");
}
return "";
}
}
private JsonObject getResponseJsonObject(HttpURLConnection connection) throws IOException, ExchangeClientException {
try{
JsonParser parser = new JsonParser();
JsonObject jsonResponse = parser.parse(getResponseString(connection)).getAsJsonObject();
JsonElement element = jsonResponse.get("exchangeResponse");
if(SDK.DEBUG){
System.out.println("RESPONSE CONTENT: " +element.getAsJsonObject().toString());
}
return element.getAsJsonObject();
}catch (Exception e) {
throw new ExchangeClientException(500, "Failed to read Exchange response", e);
}
}
private void loadErrorResponseJsonObject(HttpURLConnection connection) throws ExchangeClientException {
try (BufferedReader br = new BufferedReader(new InputStreamReader(connection.getErrorStream()))) {
StringBuilder sb = new StringBuilder();
String line;
while ((line = br.readLine()) != null) {
sb.append(line).append("\n");
}
if(SDK.DEBUG){
System.out.println("RESPONSE CONTENT: " +sb.toString());
}
JsonParser parser = new JsonParser();
JsonObject jsonResponse = parser.parse(sb.toString()).getAsJsonObject();
JsonObject exchangeResponse = jsonResponse.get("exchangeResponse").getAsJsonObject();
_responseErrorCode = exchangeResponse.get("code").getAsInt();
_responseErrorMessage = exchangeResponse.get("exceptionMessage").getAsString();
} catch (Exception e) {
throw new ExchangeClientException(500, "Failed to load Exchange response", e);
}
}
private String validateConfigOption(String configParameter) throws ExchangeClientException {
if(_configManager.exists(configParameter)){
return _configManager.getConfigValue(configParameter);
}else{
throw new ExchangeClientException(101, "Required config parameter "+configParameter+" not supplied");
}
}
private static class HttpCodes {
private static int HTTP_200_OK = 200;
private static int HTTP_201_CREATED = 201;
private static int HTTP_400_BAD_REQUEST = 400;
private static int HTTP_401_UNAUTHORIZED = 401;
private static int HTTP_404_NOTFOUND = 404;
private static int HTTP_500_INTERNAL_SERVER_ERROR = 500;
private static int HTTP_501_INTERNAL_SERVER_ERROR = 501;
}
private enum EnvironmentType {
DEV("DEV", "Development or System Test"),
UAT("UAT", "User acceptance testing or model office"),
PROD("PROD", "Production/Live environment");
private String _value;
private String _displayName;
EnvironmentType(String value, String name) {
_value = value;
_displayName = name;
}
public String getValue() {
return _value;
}
public String getDisplayName() {
return _displayName;
}
@Override
public String toString() {
return _displayName;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy