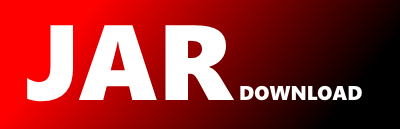
net.dreceiptx.receipt.invoice.Invoice Maven / Gradle / Ivy
/*
* Copyright 2016 Digital Receipt Exchange Limited
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.dreceiptx.receipt.invoice;
import net.dreceiptx.receipt.common.TransactionalParty;
import net.dreceiptx.receipt.lineitem.LineItem;
import net.dreceiptx.config.ConfigManager;
import net.dreceiptx.receipt.allowanceCharge.ReceiptAllowanceCharge;
import net.dreceiptx.receipt.common.LocationInformation;
import net.dreceiptx.receipt.common.DespatchInformation;
import net.dreceiptx.receipt.validation.ReceiptValidation;
import com.google.gson.annotations.SerializedName;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.concurrent.atomic.AtomicInteger;
import static net.dreceiptx.receipt.validation.ValidationErrors.ReceiptMustHaveALeastLineItem;
import net.dreceiptx.receipt.tax.TaxCode;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.HashMap;
import java.util.Map;
public class Invoice {
@SerializedName("documentStatusCode") private final String _documentStatusCode = "ORIGINAL";
@SerializedName("invoiceType") private final String _invoiceType = "TAX_INVOICE";
@SerializedName("invoiceCurrencyCode") private String _invoiceCurrencyCode;
@SerializedName("countryOfSupplyOfGoods") private String _countryOfSupplyOfGoods;
private transient LocationInformation _origin = new LocationInformation();
private transient LocationInformation _destination = new LocationInformation();
private transient DespatchInformation _despatchInformation = new DespatchInformation();
private transient AtomicInteger _lineItemId = null;
private transient AtomicInteger _allowanceOrChargeId = null;
private transient Date _creationDateTime = null;
private transient List _invoiceLineItems = new ArrayList();
private transient List _allowanceOrCharges = new ArrayList();
private transient String _invoiceIdentification;
private transient String _merchantName;
private transient Map _companyTaxNumbers = new HashMap<>();
private transient String _purchaseOrder;
private transient String _customerReference;
private transient String _salesOrderReference = null;
private transient String _defaultTimeZone;
private transient String _dateTimeFormat = "yyyy-MM-dd'T'HH:mm:ssZ";
@SerializedName("billTo") private TransactionalParty _billTo;
public Invoice() {
_lineItemId = new AtomicInteger(1);
_allowanceOrChargeId = new AtomicInteger(1);
}
public Invoice(ConfigManager configManager) {
_invoiceCurrencyCode = configManager.getConfigValue("default.currency");
_countryOfSupplyOfGoods = configManager.getConfigValue("default.country");
_defaultTimeZone = configManager.getConfigValue("default.timezone");
_creationDateTime = new Date();
_lineItemId = new AtomicInteger(1);
_allowanceOrChargeId = new AtomicInteger(1);
}
public String getMerchantName() {
return _merchantName;
}
public void setMerchantName(String merchantName) {
_merchantName = merchantName;
}
public String getCompanyTaxNumber(TaxCode taxCode) {
return _companyTaxNumbers.get(taxCode.getValue());
}
public void addCompanyTaxNumber(String taxCode, String taxNumber) {
_companyTaxNumbers.put(taxCode, taxNumber);
}
public void setPurchaseOrder(String purchaseOrder) {
_purchaseOrder = purchaseOrder;
}
public String getPurchaseOrder(){
return _purchaseOrder;
}
public void setCustomerReference(String customerReference) {
_customerReference = customerReference;
}
public String getCustomerReference(){
return _customerReference;
}
public void setSalesOrderReference(String salesOrderReference) {
_salesOrderReference = salesOrderReference;
}
public String getSalesOrderReference(){
return _salesOrderReference;
}
public void setCreationDateTime(Date date){
_creationDateTime = date;
}
public Date getCreationDateTime(){
return _creationDateTime;
}
public String getCreationDateTimeString(){
DateFormat dateFormat = new SimpleDateFormat(_dateTimeFormat);
return dateFormat.format(_creationDateTime);
}
public void setInvoiceIdentification(String invoiceIdentification) {
_invoiceIdentification = invoiceIdentification;
}
public String getInvoiceIdentification() {
return _invoiceIdentification;
}
public String getInvoiceCurrencyCode() {
return _invoiceCurrencyCode;
}
public void setInvoiceCurrencyCode(String invoiceCurrencyCode) {
_invoiceCurrencyCode = invoiceCurrencyCode;
}
public String getCountryOfSupplyOfGoods() {
return _countryOfSupplyOfGoods;
}
public void setCountryOfSupplyOfGoods(String countryOfSupplyOfGoods) {
_countryOfSupplyOfGoods = countryOfSupplyOfGoods;
}
public List getInvoiceLineItems() {
return _invoiceLineItems;
}
public void setInvoiceLineItems(List invoiceLineItems) {
_invoiceLineItems = invoiceLineItems;
}
public List getAllowanceOrCharges() {
return _allowanceOrCharges;
}
public void setOriginInformation(LocationInformation originInformation){
_origin = originInformation;
}
public LocationInformation getOriginInformation(){
return _origin;
}
public void setDestinationInformation(LocationInformation destinationInformation){
_destination = destinationInformation;
}
public LocationInformation getDestinationInformation(){
return _destination;
}
public DespatchInformation getDespatchInformation(){
return _despatchInformation;
}
public void setBillingInformation(String name) {
_billTo = new TransactionalParty(name);
}
public void setBillingInformation(TransactionalParty billToTransactionalParty) {
_billTo = billToTransactionalParty;
}
public boolean isBillingInformationSet() {
return (_billTo != null);
}
public String getBillingOrganisationName() {
if(_billTo != null){
return _billTo.getOrganisationName();
}else{
return null;
}
}
public void setDespatchInformation(DespatchInformation despatchInformation){
_despatchInformation = despatchInformation;
}
public BigDecimal getTotal() {
return this.getSubTotal().add(this.getTaxesTotal()).add(this.getSubTotalAllowances()).subtract(this.getSubTotalCharges());
}
public BigDecimal getTaxPercentage() {
BigDecimal subTotal = this.getSubTotal().add(this.getSubTotalAllowances()).add(this.getSubTotalCharges());
BigDecimal taxPercentage = BigDecimal.valueOf(0);
if (!subTotal.equals(BigDecimal.valueOf(0))) {
taxPercentage = this.getTaxesTotal().divide(subTotal, 2, BigDecimal.ROUND_HALF_UP).multiply(BigDecimal.valueOf(100));
}
return taxPercentage;
}
private boolean isNullOrWhiteSpace(String value) {
return value == null || value.isEmpty();
}
public BigDecimal getSubTotal() {
BigDecimal total = BigDecimal.valueOf(0);
for (LineItem lineItem : _invoiceLineItems) {
total = total.add(lineItem.getSubTotal());
}
return total;
}
public BigDecimal getTaxesTotal() {
BigDecimal total = BigDecimal.valueOf(0);
for (LineItem lineItem : _invoiceLineItems) {
total = total.add(lineItem.getTaxesTotal());
}
for (ReceiptAllowanceCharge allowanceCharge : _allowanceOrCharges) {
total = total.add(allowanceCharge.getTaxesTotal());
}
return total;
}
//TODO: Consider renaming TaxesTotalByTaxCode?
public BigDecimal getTaxesTotal(TaxCode taxCode) {
BigDecimal total = BigDecimal.valueOf(0);
for (LineItem lineItem : _invoiceLineItems) {
total = total.add(lineItem.getTaxesTotal(taxCode));
}
for (ReceiptAllowanceCharge allowanceCharge : _allowanceOrCharges) {
total = total.add(allowanceCharge.getTaxesTotal(taxCode));
}
return total;
}
public BigDecimal getSubTotalCharges() {
BigDecimal total = BigDecimal.valueOf(0);
for (ReceiptAllowanceCharge allowanceCharge : _allowanceOrCharges) {
if (allowanceCharge.isCharge()) {
total = total.add(allowanceCharge.getSubTotal());
}
}
return total;
}
public BigDecimal getSubTotalAllowances() {
BigDecimal total = BigDecimal.valueOf(0);
for (ReceiptAllowanceCharge allowanceCharge : _allowanceOrCharges) {
if (allowanceCharge.isAllowance()) {
total = total.add(allowanceCharge.getSubTotal());
}
}
return total;
}
public int addLineItem(LineItem lineItem) {
lineItem.setLineItemId(_lineItemId.getAndIncrement());
_invoiceLineItems.add(lineItem);
return lineItem.getLineItemId();
}
public void removeLineItem(int lineItemId) {
LineItem item = null;
for (LineItem lineItem : _invoiceLineItems) {
if (lineItem.getLineItemId() == lineItemId) {
item = lineItem;
break;
}
}
if (item != null) {
_invoiceLineItems.remove(item);
}
}
public boolean addAllowanceOrCharge(ReceiptAllowanceCharge receiptAllowanceCharge){
_allowanceOrCharges.add(receiptAllowanceCharge);
return true;
}
public void removeAllowanceOrChange(int id) {
ReceiptAllowanceCharge item = null;
for (ReceiptAllowanceCharge receiptAllowanceCharge : _allowanceOrCharges) {
if (receiptAllowanceCharge.getId() == id) {
item = receiptAllowanceCharge;
break;
}
}
if (item != null) {
_allowanceOrCharges.remove(item);
}
}
public ReceiptValidation validate(ReceiptValidation receiptValidation) {
if (_invoiceLineItems.size() < 1) {
receiptValidation.AddError(ReceiptMustHaveALeastLineItem);
}
return receiptValidation;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy