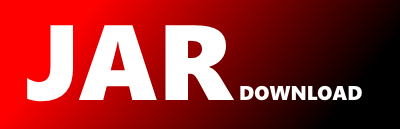
net.dreceiptx.receipt.lineitem.travel.GroundTransport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of drx-sdk-java Show documentation
Show all versions of drx-sdk-java Show documentation
Digital Receipt Exchange Java SDK
/*
* Copyright 2016 Digital Receipt Exchange Limited
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.dreceiptx.receipt.lineitem.travel;
import net.dreceiptx.receipt.common.GeographicalCoordinates;
import net.dreceiptx.receipt.ecom.AVPType;
import net.dreceiptx.receipt.lineitem.TradeItemDescriptionInformation;
import net.dreceiptx.receipt.lineitem.TransactionalTradeItemType;
import net.dreceiptx.receipt.lineitem.LineItem;
import java.util.Date;
public class GroundTransport extends LineItem {
public static final String LineItemTypeValue = "TRAVEL0003";
public GroundTransport(GroundTransportType groundTransportType, String provider, String shortDescription, String longDescription, double price) {
this(groundTransportType, provider, shortDescription, longDescription, 1, price);
}
public GroundTransport(GroundTransportType groundTransportType, String provider, String shortDescription, String longDescription, int quantity, double price) {
super(provider, shortDescription, longDescription , quantity, price);
setTradeItemGroupIdentificationCode(groundTransportType.code());
addTradeItemIdentification(LineItem.LineItemTypeIdentifier, GroundTransport.LineItemTypeValue);
}
public GroundTransport(TradeItemDescriptionInformation tradeItemDescriptionInformation, int quantity, double price) {
super(tradeItemDescriptionInformation, quantity, price);
_transactionalTradeItemType = TransactionalTradeItemType.MANUAL;
addTradeItemIdentification(LineItem.LineItemTypeIdentifier, GroundTransport.LineItemTypeValue);
}
public String getProvider(){
return getBrandName();
}
public GroundTransportType getGroundTransportType(){
return (GroundTransportType)getLineItemType(GroundTransportType.class, GroundTransportType.DEFAULT);
}
public String getTripDescription(){
return getDescription();
}
public void setPassengerName(String passengerName){
_AVPList.add(AVPType.PASSENGER_NAME.Code(), passengerName);
}
public String getPassengerName(){
return _AVPList.get(AVPType.PASSENGER_NAME.Code()).getValue();
}
public void setDriveName(String driverName){
_AVPList.add(AVPType.DRIVER_NAME.Code(), driverName);
}
public String getDriverName(){
return _AVPList.get(AVPType.DRIVER_NAME.Code()).getValue();
}
public void setVehicleIdentifier(String vehicleIdentifier){
_AVPList.add(AVPType.VEHICLE_IDENTIFIER.Code(), vehicleIdentifier);
}
public String getVehicleIdentifier(){
return _AVPList.get(AVPType.VEHICLE_IDENTIFIER.Code()).getValue();
}
public void setTripDistance(Double tripDistance){
_AVPList.add(AVPType.TRIP_DISTANCE.Code(), tripDistance.toString());
}
public Double getTripDistance(){
if(_AVPList.has(AVPType.TRIP_DISTANCE.Code())){
return Double.parseDouble(_AVPList.get(AVPType.TRIP_DISTANCE.Code()).getValue());
}
return null;
}
public Date getDepartureDate(){
return getDespatchDate();
}
public void setDepartureDate(Date departureDate){
setDespatchDate(departureDate);
}
public Date getArrivalDate(){
return getDeliveryDate();
}
public void setArrivalDate(Date arrivalDate){
setDeliveryDate(arrivalDate);
}
public void setBookingNumber(String bookingNumber){
_serialNumber = bookingNumber;
}
public String getBookingNumber(){
return _serialNumber;
}
public void setDepartureGeoLocation(GeographicalCoordinates geographicalCoordinates) {
_origin.setGeographicalCoordinates(geographicalCoordinates);
}
public GeographicalCoordinates getDepartureGeoLocation(){
return _origin.getGeographicalCoordinates();
}
public void setArrivalGeoLocation(GeographicalCoordinates geographicalCoordinates) {
_destination.setGeographicalCoordinates(geographicalCoordinates);
}
public GeographicalCoordinates getArrivalGeoLocation(){
return _destination.getGeographicalCoordinates();
}
public void setDepartureDetails(Date departureDate, GeographicalCoordinates geographicalCoordinates){
setDespatchDate(departureDate);
setDepartureGeoLocation(geographicalCoordinates);
}
public void setArrivalDetails(Date arrivalDate, GeographicalCoordinates geographicalCoordinates){
setArrivalDate(arrivalDate);
setArrivalGeoLocation(geographicalCoordinates);
}
public void setTripCode(String tripCode){
setBillingCostCentre(tripCode);
}
public String getTripCode(){
return getBillingCostCentre();
}
public void setTripReason(String reason){
setNote(reason);
}
public String getTripReason(){
return getNote();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy