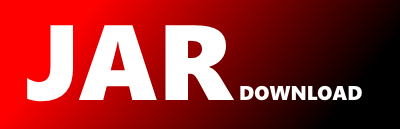
net.dubboclub.maven.DubbomRunMojo Maven / Gradle / Ivy
package net.dubboclub.maven;
import org.apache.commons.lang.StringUtils;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugins.annotations.LifecyclePhase;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import org.apache.maven.plugins.annotations.ResolutionScope;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import java.io.File;
import java.io.FileFilter;
import java.io.IOException;
import java.lang.reflect.Constructor;
import java.net.URL;
import java.net.URLClassLoader;
import java.util.jar.JarFile;
@Mojo(name = "run", defaultPhase = LifecyclePhase.TEST_COMPILE, threadSafe = true, requiresDependencyResolution = ResolutionScope.RUNTIME)
public class DubbomRunMojo extends AbstractDubbomMojo {
/**
* dubbom的配置文件地址,是相对classpath位置
* 默认是在classpath根目录的dubbom.properties文件
*/
@Parameter(property = "dubbomProperties", defaultValue = "dubbom.properties", required = true)
private String dubbomProperties;
/**
* spring 的xml配置文件地址,默认是classpath*:META-INF/spring/*.xml
*/
@Parameter(property = "springXMLPath", defaultValue = "classpath*:META-INF/spring/*.xml", required = true)
private String springXMLPath;
public void execute() throws MojoExecutionException, MojoFailureException {
pack();
File[] jars = targetDir.listFiles(new FileFilter() {
public boolean accept(File pathname) {
return pathname.getName().endsWith(".jar");
}
});
URL[] urls = new URL[jars.length];
int index = 0;
for (File jar : jars) {
try {
getLog().info(jar.getPath());
URL jarUrl = jar.toURI().toURL();
urls[index] = jarUrl;
index++;
} catch (IOException e) {
throw new MojoExecutionException("run project " + mavenProject.getArtifactId() + " occur an exception.", e);
}
}
URLClassLoader urlClassLoader = new URLClassLoader(urls, DubbomRunMojo.class.getClassLoader());
Thread.currentThread().setContextClassLoader(urlClassLoader);
System.setProperty("dubbo.properties.file", dubbomProperties);
String[] paths = StringUtils.split(springXMLPath, ",");
getLog().info("sprint xml path " + springXMLPath);
getLog().info("dubbom properties " + System.getProperty("dubbo.properties.file"));
ClassPathXmlApplicationContext applicationContext = new ClassPathXmlApplicationContext(paths);
applicationContext.setClassLoader(urlClassLoader);
applicationContext.start();
synchronized (DubbomRunMojo.class) {
while (true) {
try {
DubbomRunMojo.class.wait();
} catch (Throwable e) {
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy