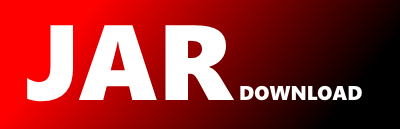
net.dubboclub.restful.export.mapping.ServiceHandler Maven / Gradle / Ivy
package net.dubboclub.restful.export.mapping;
import com.alibaba.dubbo.common.Constants;
import net.dubboclub.restful.exception.IllegalDefinitionException;
import net.dubboclub.restful.modal.RestfulPackage;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.util.ArrayList;
import java.util.List;
/**
* @date: 2016/2/22.
* @author:bieber.
* @project:dubbo-plus.
* @package:net.dubboclub.restful.export.container.
* @version:1.0.0
* @fix:
* @description: 描述功能
*/
public class ServiceHandler {
private String group;
private String version;
private Class serviceType;
private T impl;
private List methodHandlerList;
private String path;
public ServiceHandler(String group, String version, Class serviceType,String path, T impl) {
if(serviceType==null){
throw new IllegalArgumentException("[serviceType] must not be null");
}
this.group = Constants.ANY_VALUE.equals(group)?null:group;
this.version = Constants.ANY_VALUE.equals(version)?null:version;
this.serviceType = serviceType;
this.impl = impl;
methodHandlerList =new ArrayList();
this.path=path;
initHandler();
}
public String getPath() {
return path;
}
private void initHandler(){
Class type = this.serviceType;
while(type!=null&&type!=Object.class){
Method[] methods = type.getDeclaredMethods();
for(Method method:methods){
if(Modifier.isPublic(method.getModifiers())&&method.getParameterTypes().length==1&&RestfulPackage.class.isAssignableFrom(method.getParameterTypes()[0])){
MethodHandler methodHandler = new MethodHandler(method.getName(),method,method.getParameterTypes()[0],this.impl);
methodHandlerList.add(methodHandler);
}else{
throw new IllegalDefinitionException(type,method);
}
}
type = type.getSuperclass();
}
}
public List getMethodHandlerList() {
return methodHandlerList;
}
public MethodHandler mapping(RequestEntity requestEntity){
for(MethodHandler methodHandler:methodHandlerList){
if(methodHandler.support(requestEntity)){
return methodHandler;
}
}
return null;
}
public String getGroup() {
return group;
}
public String getVersion() {
return version;
}
public Class getServiceType() {
return serviceType;
}
public T getImpl() {
return impl;
}
@Override
public String toString() {
return "ServiceHandler{" +
"serviceType=" + serviceType.getName() +
", version='" + version + '\'' +
", group='" + group + '\'' +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy