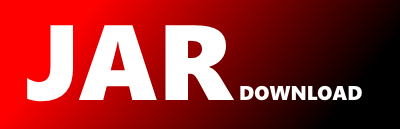
net.e6tech.elements.network.restful.WSResponseImpl Maven / Gradle / Ivy
/*
Copyright 2015-2019 Futeh Kao
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package net.e6tech.elements.network.restful;
import net.e6tech.elements.common.util.SystemException;
import javax.ws.rs.core.*;
import java.io.IOException;
import java.lang.annotation.Annotation;
import java.net.URI;
import java.util.Date;
import java.util.Locale;
import java.util.Map;
import java.util.Set;
/**
* Created by futeh.
*/
public class WSResponseImpl extends javax.ws.rs.core.Response {
private Response response;
WSResponseImpl(Response response) {
this.response = response;
}
@Override
public int getStatus() {
return response.getResponseCode();
}
@Override
public StatusType getStatusInfo() {
return null;
}
@Override
public Object getEntity() {
return null;
}
@Override
public T readEntity(Class entityType) {
try {
return response.read(entityType);
} catch (IOException e) {
throw new SystemException(e);
}
}
@Override
public T readEntity(GenericType entityType) {
try {
return response.read(entityType.getType());
} catch (IOException e) {
throw new SystemException(e);
}
}
@Override
public T readEntity(Class entityType, Annotation[] annotations) {
throw new UnsupportedOperationException();
}
@Override
public T readEntity(GenericType entityType, Annotation[] annotations) {
throw new UnsupportedOperationException();
}
@Override
public boolean hasEntity() {
return response != null && response.getResult() != null;
}
@Override
public boolean bufferEntity() {
return false;
}
@Override
public void close() {
// do nothing
}
@Override
public MediaType getMediaType() {
throw new UnsupportedOperationException();
}
@Override
public Locale getLanguage() {
throw new UnsupportedOperationException();
}
@Override
public int getLength() {
throw new UnsupportedOperationException();
}
@Override
public Set getAllowedMethods() {
throw new UnsupportedOperationException();
}
@Override
public Map getCookies() {
throw new UnsupportedOperationException();
}
@Override
public EntityTag getEntityTag() {
throw new UnsupportedOperationException();
}
@Override
public Date getDate() {
throw new UnsupportedOperationException();
}
@Override
public Date getLastModified() {
throw new UnsupportedOperationException();
}
@Override
public URI getLocation() {
throw new UnsupportedOperationException();
}
@Override
public Set getLinks() {
throw new UnsupportedOperationException();
}
@Override
public boolean hasLink(String relation) {
throw new UnsupportedOperationException();
}
@Override
public Link getLink(String relation) {
throw new UnsupportedOperationException();
}
@Override
public Link.Builder getLinkBuilder(String relation) {
throw new UnsupportedOperationException();
}
@Override
public MultivaluedMap getMetadata() {
throw new UnsupportedOperationException();
}
@Override
public MultivaluedMap getStringHeaders() {
throw new UnsupportedOperationException();
}
@Override
public String getHeaderString(String name) {
throw new UnsupportedOperationException();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy