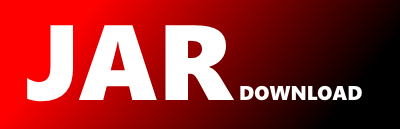
net.eusashead.parquet.util.Assert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of parquet-utils Show documentation
Show all versions of parquet-utils Show documentation
Parquet is a Java REST framework built on Yoke and Vert.x
The newest version!
package net.eusashead.parquet.util;
import java.util.Collection;
public class Assert {
public static void notNull(Object object) {
notNull(object, "Parameter cannot be null.");
}
public static void notNull(Object object, String message) {
if (object == null) {
throw new IllegalArgumentException(message);
}
}
public static void isNull(Object object) {
isNull(object, "Parameter must be null.");
}
public static void isNull(Object object, String message) {
if (object != null) {
throw new IllegalArgumentException(message);
}
}
public static void noNullElement(Object[] target) {
noNullElement(target, "Array must have no null elements.");
}
public static void noNullElement(Object[] target, String message) {
if (target != null) {
for (Object field : target) {
if (field == null) {
throw new IllegalArgumentException(message);
}
}
}
}
public static void notEmpty(Object[] target) {
notEmpty(target, "Parameter have length greater than zero.");
}
public static void notEmpty(Object[] target, String message) {
notNull(target);
if (target.length == 0) {
throw new IllegalArgumentException(message);
}
}
public static void greaterThan(int i, int obj) {
greaterThan(i, obj, String.format("Parameter must be greater than %s", i));
}
public static void greaterThan(int i, int obj, String message) {
if (obj <= i) {
throw new IllegalArgumentException(message);
}
}
public static void greaterThanEqual(int i, int obj) {
greaterThanEqual(i, obj, String.format("Parameter must be greater than or equal to %s", i));
}
public static void greaterThanEqual(int i, int obj, String message) {
if (obj < i) {
throw new IllegalArgumentException(message);
}
}
public static void lessThan(int i, int obj) {
lessThan(i, obj, String.format("Parameter must be less than %s", i));
}
public static void lessThan(int i, int obj, String message) {
if (obj >= i) {
throw new IllegalArgumentException(message);
}
}
public static void typeOf(Class clazz, Object object) {
typeOf(clazz, object, String.format("Object %s is not an instance of %s", object, clazz));
}
public static void typeOf(Class clazz, Object object, String message) {
if (!object.getClass().equals(clazz)) {
throw new IllegalArgumentException(message);
}
}
public static void notEmpty(String target) {
notEmpty(target, "String cannot be null or empty.");
}
public static void notEmpty(String target, String message) {
if (target == null || target.length() == 0) {
throw new IllegalArgumentException(message);
}
}
public static void equals(Number j, Number i) {
equals(j, i, String.format("Argument is not equal to ", i));
}
public static void equals(Number j, Number i, String message) {
if (!j.equals(i)) {
throw new IllegalArgumentException(message);
}
}
public static void isTrue(boolean value) {
isTrue(value, "Argument must be true");
}
public static void isTrue(boolean value, String message) {
if (!value) {
throw new IllegalArgumentException(message);
}
}
public static void notEmpty(Collection target, String message) {
if (target == null || target.size() == 0) {
throw new IllegalArgumentException(message);
}
}
public static void noNullElement(Collection target, String message) {
if (target != null) {
for (Object field : target) {
if (field == null) {
throw new IllegalArgumentException(message);
}
}
}
}
}