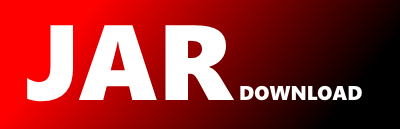
net.finmath.plots.Plot2D Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finmath-lib-plot-extensions Show documentation
Show all versions of finmath-lib-plot-extensions Show documentation
finmath lib plot extensions provide convenient
plotting methods by providing consistent wrappers
to plot libraries (like JFreeChart or JavaFX).
The newest version!
/*
* (c) Copyright Christian P. Fries, Germany. Contact: [email protected].
*
* Created on 21 May 2018
*/
package net.finmath.plots;
import java.awt.Color;
import java.io.File;
import java.io.IOException;
import java.text.NumberFormat;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.function.DoubleUnaryOperator;
import java.util.function.Function;
import java.util.stream.Collectors;
import javax.swing.JFrame;
import javax.swing.JPanel;
import org.jfree.chart.ChartPanel;
import org.jfree.chart.JFreeChart;
import org.jfree.chart.axis.NumberAxis;
import org.jfree.chart.renderer.xy.XYAreaRenderer;
import org.jfree.chart.renderer.xy.XYItemRenderer;
import org.jfree.chart.renderer.xy.XYLineAndShapeRenderer;
import org.jfree.data.Range;
import org.jfree.data.xy.XYSeries;
import org.jfree.data.xy.XYSeriesCollection;
import net.finmath.plots.jfreechart.JFreeChartUtilities;
import net.finmath.plots.jfreechart.StyleGuide;
/**
* Small convenient wrapper for JFreeChart line plot derived.
*
* @author Christian Fries
*/
public class Plot2D implements Plot {
private List plotables;
private String title = "";
private String xAxisLabel = "x";
private String yAxisLabel = "y";
private NumberFormat xAxisNumberFormat;
private NumberFormat yAxisNumberFormat;
private Boolean isLegendVisible = false;
private transient JFrame frame;
private transient JFreeChart chart;
private final Object updateLock = new Object();
private Double xmin;
private Double xmax;
private Double ymin;
private Double ymax;
public Plot2D(final List plotables) {
super();
this.plotables = plotables;
}
public Plot2D(final double xmin, final double xmax, final int numberOfPointsX, final List> doubleUnaryOperators) {
this(doubleUnaryOperators.stream().map(namedFunction -> { return new PlotableFunction2D(xmin, xmax, numberOfPointsX, namedFunction, null); }).collect(Collectors.toList()));
}
public Plot2D(final double xmin, final double xmax, final int numberOfPointsX, final DoubleUnaryOperator[] doubleUnaryOperators) {
this(xmin, xmax, numberOfPointsX,
Arrays.stream(doubleUnaryOperators).map(operator -> new Named("", operator)).collect(Collectors.toList())
);
}
public Plot2D(final double xmin, final double xmax, final int numberOfPointsX, final DoubleUnaryOperator function) {
this(xmin, xmax, numberOfPointsX, Collections.singletonList(new Named("",function)));
}
public Plot2D(final double xmin, final double xmax, final DoubleUnaryOperator function) {
this(xmin, xmax, 300, Collections.singletonList(new Named("",function)));
}
public Plot2D(final double xmin, final double xmax, final int numberOfPointsX, final Function function) {
this(xmin, xmax, numberOfPointsX, Collections.singletonList(new Named("", new DoubleUnaryOperator() {
@Override
public double applyAsDouble(double operand) {
return function.apply(operand);
}
})));
}
private void init() {
synchronized (updateLock) {
if(chart != null) {
return;
}
/*
* Creates an empty chart (the update method will set the data)
*/
final XYLineAndShapeRenderer renderer = new XYLineAndShapeRenderer();
final XYSeriesCollection data = new XYSeriesCollection();
chart = JFreeChartUtilities.getXYPlotChart(title, xAxisLabel, "#.##" /* xAxisNumberFormat */, yAxisLabel, "#.##" /* yAxisNumberFormat */, data, renderer, isLegendVisible);
}
}
private void update() {
synchronized (updateLock) {
final Map rangeAxisMap = new HashMap();
for(int functionIndex=0; functionIndex plotableSeries = plotable.getSeries();
final XYSeries series = new XYSeries(plotable.getName());
for(int i = 0; i plotables) {
this.plotables = plotables;
synchronized (updateLock) {
if(chart != null) {
update();
}
}
return this;
}
@Override
public Plot2D setTitle(final String title) {
this.title = title;
update();
return this;
}
@Override
public Plot2D setXAxisLabel(final String xAxisLabel) {
this.xAxisLabel = xAxisLabel;
synchronized (updateLock) {
if(chart != null) {
chart.getXYPlot().getDomainAxis().setLabel(xAxisLabel);
}
}
return this;
}
@Override
public Plot2D setYAxisLabel(final String yAxisLabel) {
this.yAxisLabel = yAxisLabel;
synchronized (updateLock) {
if(chart != null) {
chart.getXYPlot().getRangeAxis().setLabel(yAxisLabel);
}
}
return this;
}
@Override
public Plot setZAxisLabel(final String zAxisLabel) {
throw new UnsupportedOperationException("The 2D plot does not suport a z-axis. Try 3D plot instead.");
}
public Plot2D setXAxisNumberFormat(final NumberFormat xAxisNumberFormat) {
this.xAxisNumberFormat = xAxisNumberFormat;
return this;
}
public Plot2D setYAxisNumberFormat(final NumberFormat yAxisNumberFormat) {
this.yAxisNumberFormat = yAxisNumberFormat;
return this;
}
public Plot2D setXRange(final double xmin, final double xmax) {
this.xmin = xmin;
this.xmax = xmax;
update();
return this;
}
public Plot2D setYRange(final double ymin, final double ymax) {
this.ymin = ymin;
this.ymax = ymax;
update();
return this;
}
/**
* @param isLegendVisible the isLegendVisible to set
*/
@Override
public Plot setIsLegendVisible(final Boolean isLegendVisible) {
this.isLegendVisible = isLegendVisible;
update();
return this;
}
@Override
public String toString() {
return "Plot2D [plotables=" + plotables + ", title=" + title + ", xAxisLabel=" + xAxisLabel + ", yAxisLabel="
+ yAxisLabel + ", xAxisNumberFormat=" + xAxisNumberFormat + ", yAxisNumberFormat=" + yAxisNumberFormat
+ ", isLegendVisible=" + isLegendVisible + ", ymin=" + ymin + ", ymax=" + ymax + "]";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy