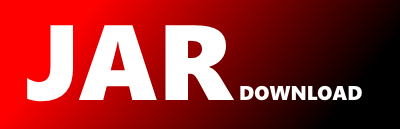
net.finmath.plots.Plot2DBarFX Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finmath-lib-plot-extensions Show documentation
Show all versions of finmath-lib-plot-extensions Show documentation
finmath lib plot extensions provide convenient
plotting methods by providing consistent wrappers
to plot libraries (like JFreeChart or JavaFX).
The newest version!
/*
* (c) Copyright Christian P. Fries, Germany. Contact: [email protected].
*
* Created on 21 May 2018
*/
package net.finmath.plots;
import java.awt.Graphics2D;
import java.awt.geom.Rectangle2D;
import java.awt.image.BufferedImage;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.text.NumberFormat;
import java.util.ArrayList;
import java.util.List;
import javax.imageio.ImageIO;
import javax.swing.JFrame;
import javax.swing.SwingUtilities;
import javafx.application.Platform;
import javafx.embed.swing.JFXPanel;
import javafx.scene.Scene;
import javafx.scene.chart.BarChart;
import javafx.scene.chart.CategoryAxis;
import javafx.scene.chart.Chart;
import javafx.scene.chart.NumberAxis;
import javafx.scene.chart.StackedBarChart;
import javafx.scene.chart.XYChart;
import javafx.scene.image.WritableImage;
import javafx.scene.paint.Color;
import javafx.scene.text.Font;
/**
* Small convenient wrapper for Java FX line plot.
*
* @author Christian Fries
*/
public class Plot2DBarFX implements Plot {
private List plotables;
private String title = "";
private String xAxisLabel = "x";
private String yAxisLabel = "y";
private NumberFormat xAxisNumberFormat;
private NumberFormat yAxisNumberFormat;
private Double yAxisLowerBound;
private Double yAxisUpperBound;
private Double yAxisTick;
private Boolean isLegendVisible = false;
private Boolean isSeriesStacked = false;
private transient JFrame frame;
private XYChart chart;
private final Object updateLock = new Object();
public Plot2DBarFX(final List plotables, final String title, final String xAxisLabel, final String yAxisLabel,
final NumberFormat yAxisNumberFormat, final Double yAxisLowerBound, final Double yAxisUpperBound, final Double yAxisTick,
final Boolean isLegendVisible) {
super();
this.plotables = plotables;
this.title = title;
this.xAxisLabel = xAxisLabel;
this.yAxisLabel = yAxisLabel;
this.yAxisNumberFormat = yAxisNumberFormat;
this.yAxisLowerBound = yAxisLowerBound;
this.yAxisUpperBound = yAxisUpperBound;
this.yAxisTick = yAxisTick;
this.isLegendVisible = isLegendVisible;
}
public Plot2DBarFX(final List plotables) {
super();
this.plotables = plotables;
}
/**
*
*/
public Plot2DBarFX() {
}
public static Plot2DBarFX of(final String name, final String[] labels, final double[] values, final String title, final String xAxisLabel, final String yAxisLabel, final NumberFormat yAxisNumberFormat, final boolean isLegendVisible) {
double min = Double.MAX_VALUE;
double max = -Double.MAX_VALUE;
final List plotables = new ArrayList();
final List histogramAsList = new ArrayList();
for(int i=0; i getSeries() {
return histogramAsList;
}
};
plotables.add(histo);
return new Plot2DBarFX(plotables, title, xAxisLabel, yAxisLabel, yAxisNumberFormat, min, max, (max-min)/10.0, isLegendVisible);
}
public static Plot2DBarFX of(final String[] labels, final double[] values, final String title, final String xAxisLabel, final String yAxisLabel, final NumberFormat yAxisNumberFormat, final boolean isLegendVisible) {
double min = Double.MAX_VALUE;
double max = -Double.MAX_VALUE;
final List plotables = new ArrayList();
final String name = "";
final List histogramAsList = new ArrayList();
for(int i=0; i getSeries() {
return histogramAsList;
}
};
plotables.add(histo);
return new Plot2DBarFX(plotables, title, xAxisLabel, yAxisLabel, yAxisNumberFormat, min, max, (max-min)/10.0, isLegendVisible);
}
private void init() {
//defining the axes
final CategoryAxis xAxis = new CategoryAxis();
xAxis.setAutoRanging(true);
xAxis.setAnimated(false);
final NumberAxis yAxis = new NumberAxis(yAxisLowerBound, yAxisUpperBound, yAxisTick);
xAxis.setLabel(xAxisLabel);
yAxis.setLabel(yAxisLabel);
xAxis.setTickLabelFont(new Font("Helvetica", 14));
yAxis.setTickLabelFont(new Font("Helvetica", 14));
// creating the chart
chart = isSeriesStacked ? new StackedBarChart(xAxis,yAxis) : new BarChart(xAxis,yAxis);
chart.setAnimated(true);
chart.setStyle("-fx-font-size: " + 16 + "px; -fx-font-family: Helvetica;");
update();
}
private void update() {
Platform.runLater(new Runnable() {
@Override public void run() {
if(plotables == null) {
return;
}
chart.setTitle(title);
for(int functionIndex=0; functionIndex plotableSeries = plotable.getSeries();
XYChart.Series series = null;
if(functionIndex < chart.getData().size()) {
series = chart.getData().get(functionIndex);
}
if(series == null) {
series = new XYChart.Series();
chart.getData().add(functionIndex, series);
}
series.setName(plotable.getName());
for(int i = 0; i data = null;
if(i < series.getData().size()) {
data = series.getData().get(i);
}
if(data == null) {
data = new XYChart.Data(plotableSeries.get(i).getName(), plotableSeries.get(i).getValue());
/*
if(style != null && style.getShape() != null) {
data.setNode(new javafx.scene.shape.Rectangle(30,30, color));
}
*/
series.getData().add(i, data);
}
data.setXValue(plotableSeries.get(i).getName());
data.setYValue(plotableSeries.get(i).getValue());
}
/*
* Apply style to line
*/
// if(style != null && style.getStoke() != null) series.getNode().setStyle("-fx-stroke: rgba(" + rgba + ");");
// else series.getNode().setStyle("-fx-stroke: none;");
/*
String rgb = String.format("%d, %d, %d", (int) (color.getRed() * 255), (int) (color.getGreen() * 255),(int) (color.getBlue() * 255));
series.getNode().setStyle("-fx-stroke: rgba(" + rgb + ",1.0); -fx-background-color: #FF0000, white;");
series.getNode().setStyle("-fx-stroke: rgba(" + rgb + ",1.0); -fx-background-color: #FF0000, white;");
*/
// lineChart.setStyle("-fx-create-symbols: false;");
// .default-color2.chart-line-symbol { -fx-background-color: #dda0dd, white; }
}
/*
Node[] legendItems = chart.lookupAll(".chart-legend-item-symbol").toArray(new Node[0]);
for(int i = 0; i plotables) {
if(chart != null) {
chart.setAnimated(true);
}
this.plotables = plotables;
synchronized (updateLock) {
if(chart != null) {
update();
}
}
if(chart != null) {
chart.setAnimated(false);
}
return this;
}
@Override
public Plot2DBarFX setTitle(final String title) {
this.title = title;
return this;
}
@Override
public Plot2DBarFX setXAxisLabel(final String xAxisLabel) {
this.xAxisLabel = xAxisLabel;
return this;
}
@Override
public Plot2DBarFX setYAxisLabel(final String yAxisLabel) {
this.yAxisLabel = yAxisLabel;
return this;
}
public Plot2DBarFX setYAxisRange(final Double min, final Double max) {
if(chart == null || chart.getYAxis() == null) {
return this;
}
if(min == null || max == null) {
chart.getYAxis().setAutoRanging(true);
}
else {
chart.getYAxis().setAutoRanging(false);
((NumberAxis)chart.getYAxis()).setLowerBound(min);
((NumberAxis)chart.getYAxis()).setUpperBound(max);
}
return this;
}
@Override
public Plot setZAxisLabel(final String zAxisLabel) {
throw new UnsupportedOperationException("The 2D plot does not suport a z-axis. Try 3D plot instead.");
}
/**
* @param isLegendVisible the isLegendVisible to set
*/
@Override
public Plot setIsLegendVisible(final Boolean isLegendVisible) {
this.isLegendVisible = isLegendVisible;
return this;
}
public void setIsSeriesStacked(final Boolean isSeriesStacked) {
this.isSeriesStacked = isSeriesStacked;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy