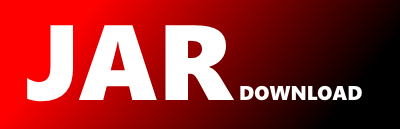
net.finmath.montecarlo.interestrate.LIBORModelMonteCarloSimulation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finmath-lib Show documentation
Show all versions of finmath-lib Show documentation
finmath lib is a Mathematical Finance Library in Java.
It provides algorithms and methodologies related to mathematical finance.
/*
* (c) Copyright Christian P. Fries, Germany. All rights reserved. Contact: [email protected].
*
* Created on 09.02.2004
*/
package net.finmath.montecarlo.interestrate;
import java.util.HashMap;
import java.util.Map;
import net.finmath.exception.CalculationException;
import net.finmath.montecarlo.BrownianMotionInterface;
import net.finmath.montecarlo.interestrate.modelplugins.AbstractLIBORCovarianceModel;
import net.finmath.montecarlo.process.AbstractProcess;
import net.finmath.montecarlo.process.AbstractProcessInterface;
import net.finmath.stochastic.RandomVariableInterface;
import net.finmath.time.TimeDiscretizationInterface;
/**
* Implements convenient methods for a libor market model,
* based on a given LIBORMarketModel
model
* and AbstractLogNormalProcess
process.
*
* @author Christian Fries
* @version 0.6
*/
public class LIBORModelMonteCarloSimulation implements LIBORModelMonteCarloSimulationInterface {
private final LIBORMarketModelInterface model;
/**
* Create a LIBOR Monte-Carlo Simulation from a given LIBORMarketModel and an AbstractProcess.
*
* @param model The LIBORMarketModel.
* @param process The process.
*/
public LIBORModelMonteCarloSimulation(LIBORMarketModelInterface model, AbstractProcess process) {
super();
this.model = model;
this.model.setProcess(process);
process.setModel(model);
}
/* (non-Javadoc)
* @see net.finmath.montecarlo.interestrate.LIBORModelMonteCarloSimulationInterface#getMonteCarloWeights(int)
*/
@Override
public RandomVariableInterface getMonteCarloWeights(int timeIndex) throws CalculationException {
return model.getProcess().getMonteCarloWeights(timeIndex);
}
/* (non-Javadoc)
* @see net.finmath.montecarlo.interestrate.LIBORModelMonteCarloSimulationInterface#getMonteCarloWeights(double)
*/
@Override
public RandomVariableInterface getMonteCarloWeights(double time) throws CalculationException {
return model.getProcess().getMonteCarloWeights(getTimeIndex(time));
}
/* (non-Javadoc)
* @see net.finmath.montecarlo.interestrate.LIBORModelMonteCarloSimulationInterface#getNumberOfFactors()
*/
@Override
public int getNumberOfFactors() {
return model.getProcess().getNumberOfFactors();
}
/* (non-Javadoc)
* @see net.finmath.montecarlo.MonteCarloSimulationInterface#getNumberOfPaths()
*/
@Override
public int getNumberOfPaths() {
return model.getProcess().getNumberOfPaths();
}
/* (non-Javadoc)
* @see net.finmath.montecarlo.MonteCarloSimulationInterface#getTime(int)
*/
@Override
public double getTime(int timeIndex) {
return model.getProcess().getTime(timeIndex);
}
/* (non-Javadoc)
* @see net.finmath.montecarlo.MonteCarloSimulationInterface#getTimeDiscretization()
*/
@Override
public TimeDiscretizationInterface getTimeDiscretization() {
return model.getProcess().getTimeDiscretization();
}
/* (non-Javadoc)
* @see net.finmath.montecarlo.MonteCarloSimulationInterface#getTimeIndex(double)
*/
@Override
public int getTimeIndex(double time) {
return model.getProcess().getTimeIndex(time);
}
@Override
public RandomVariableInterface getRandomVariableForConstant(double value) {
return model.getProcess().getBrownianMotion().getRandomVariableForConstant(value);
}
/* (non-Javadoc)
* @see net.finmath.montecarlo.interestrate.LIBORModelMonteCarloSimulationInterface#getBrownianMotion()
*/
@Override
public BrownianMotionInterface getBrownianMotion() {
return model.getProcess().getBrownianMotion();
}
/* (non-Javadoc)
* @see net.finmath.montecarlo.interestrate.LIBORModelMonteCarloSimulationInterface#getLIBOR(int, int)
*/
@Override
public RandomVariableInterface getLIBOR(int timeIndex, int liborIndex) throws CalculationException {
return model.getLIBOR(timeIndex, liborIndex);
}
/* (non-Javadoc)
* @see net.finmath.montecarlo.interestrate.LIBORModelMonteCarloSimulationInterface#getLIBORs(int)
*/
@Override
public RandomVariableInterface[] getLIBORs(int timeIndex) throws CalculationException
{
RandomVariableInterface[] randomVariableVector = new RandomVariableInterface[getNumberOfComponents()];
for(int componentIndex=0; componentIndex dataModified) throws CalculationException {
LIBORMarketModelInterface modelClone = model.getCloneWithModifiedData(dataModified);
return new LIBORModelMonteCarloSimulation(modelClone, (AbstractProcess) getProcess().clone());
}
/**
* Create a clone of this simulation modifying one of its properties (if any).
*
* @param entityKey The entity to modify.
* @param dataModified The data which should be changed in the new model
* @return Returns a clone of this model, where the specified part of the data is modified data (then it is no longer a clone :-)
* @throws net.finmath.exception.CalculationException Thrown if the valuation fails, specific cause may be available via the cause()
method.
*/
public LIBORModelMonteCarloSimulationInterface getCloneWithModifiedData(String entityKey, Object dataModified) throws CalculationException
{
Map dataModifiedMap = new HashMap();
dataModifiedMap.put(entityKey, dataModified);
return getCloneWithModifiedData(dataModifiedMap);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy