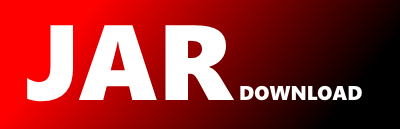
net.finmath.analytic.calibration.Solver Maven / Gradle / Ivy
/*
* (c) Copyright Christian P. Fries, Germany. Contact: [email protected].
*
* Created on 26.11.2012
*/
package net.finmath.analytic.calibration;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.Vector;
import net.finmath.analytic.model.AnalyticModelInterface;
import net.finmath.analytic.products.AnalyticProductInterface;
import net.finmath.montecarlo.RandomVariable;
import net.finmath.optimizer.SolverException;
import net.finmath.optimizer.StochasticOptimizerFactoryInterface;
import net.finmath.optimizer.StochasticOptimizerInterface;
import net.finmath.optimizer.StochasticPathwiseOptimizerFactoryLevenbergMarquardt;
import net.finmath.stochastic.RandomVariableInterface;
/**
* Generates a calibrated model for a given set
* of calibrationProducts
with respect to given Curve
s.
*
* The model and the curve are assumed to be immutable, i.e., the solver
* will return a calibrate clone, containing clones for every curve
* which is part of the set of curves to be calibrated.
*
* The calibration is performed as a multi-threaded global optimization.
* I will greatly profit from a multi-core architecture.
*
* @author Christian Fries
*/
public class Solver {
private final AnalyticModelInterface model;
private final List calibrationProducts;
private final List calibrationTargetValues;
private final double calibrationAccuracy;
private final ParameterTransformation parameterTransformation;
private StochasticOptimizerFactoryInterface optimizerFactory;
private final double evaluationTime;
private final int maxIterations = 1000;
private int iterations = 0;
private double accuracy = Double.POSITIVE_INFINITY;
/**
* Generate a solver for the given parameter objects (independents) and
* objective functions (dependents).
*
* @param model The model from which a calibrated clone should be created.
* @param calibrationProducts The objective functions.
* @param calibrationTargetValues Array of target values for the objective functions.
* @param parameterTransformation A parameter transformation, if any, otherwise null.
* @param evaluationTime Evaluation time applied to the calibration products.
* @param optimizerFactory A factory providing the optimizer (for the given objective function)
*/
public Solver(AnalyticModelInterface model, Vector calibrationProducts, List calibrationTargetValues, ParameterTransformation parameterTransformation, double evaluationTime, StochasticOptimizerFactoryInterface optimizerFactory) {
super();
this.model = model;
this.calibrationProducts = calibrationProducts;
this.calibrationTargetValues = calibrationTargetValues;
this.parameterTransformation = parameterTransformation;
this.evaluationTime = evaluationTime;
this.optimizerFactory = optimizerFactory;
this.calibrationAccuracy = 0.0;
}
/**
* Generate a solver for the given parameter objects (independents) and
* objective functions (dependents).
*
* @param model The model from which a calibrated clone should be created.
* @param calibrationProducts The objective functions.
* @param calibrationTargetValues Array of target values for the objective functions.
* @param parameterTransformation A parameter transformation, if any, otherwise null.
* @param evaluationTime Evaluation time applied to the calibration products.
* @param calibrationAccuracy The error tolerance of the solver.
*/
public Solver(AnalyticModelInterface model, Vector calibrationProducts, List calibrationTargetValues, ParameterTransformation parameterTransformation, double evaluationTime, double calibrationAccuracy) {
super();
this.model = model;
this.calibrationProducts = calibrationProducts;
this.calibrationTargetValues = calibrationTargetValues;
this.parameterTransformation = parameterTransformation;
this.evaluationTime = evaluationTime;
this.calibrationAccuracy = calibrationAccuracy;
this.optimizerFactory = null;
}
/**
* Generate a solver for the given parameter objects (independents) and
* objective functions (dependents).
*
* @param model The model from which a calibrated clone should be created.
* @param calibrationProducts The objective functions.
* @param calibrationTargetValues Array of target values for the objective functions.
* @param evaluationTime Evaluation time applied to the calibration products.
* @param calibrationAccuracy The error tolerance of the solver.
*/
public Solver(AnalyticModelInterface model, Vector calibrationProducts, List calibrationTargetValues, double evaluationTime, double calibrationAccuracy) {
this(model, calibrationProducts, calibrationTargetValues, null, evaluationTime, calibrationAccuracy);
}
/**
* Generate a solver for the given parameter objects (independents) and
* objective functions (dependents).
*
* @param model The model from which a calibrated clone should be created.
* @param calibrationProducts The objective functions.
* @param evaluationTime Evaluation time applied to the calibration products.
* @param calibrationAccuracy The error tolerance of the solver.
*/
public Solver(AnalyticModelInterface model, Vector calibrationProducts, double evaluationTime, double calibrationAccuracy) {
this(model, calibrationProducts, null, null, evaluationTime, calibrationAccuracy);
}
/**
* Generate a solver for the given parameter objects (independents) and
* objective functions (dependents).
*
* @param model The model from which a calibrated clone should be created.
* @param calibrationProducts The objective functions.
*/
public Solver(AnalyticModelInterface model, Vector calibrationProducts) {
this(model, calibrationProducts, 0.0, 0.0);
}
/**
* Find the model such that the equation
*
*
* objectiveFunctions.getValue(model) = 0
*
*
* holds.
*
* @param objectsToCalibrate The set of parameterized objects to calibrate.
* @return A reference to a calibrated clone of the given model.
* @throws net.finmath.optimizer.SolverException Thrown if the underlying optimizer does not find a solution.
*/
public AnalyticModelInterface getCalibratedModel(Set objectsToCalibrate) throws SolverException {
final ParameterAggregation parameterAggregate = new ParameterAggregation(objectsToCalibrate);
// Set solver parameters
final RandomVariableInterface[] initialParameters;
// Apply parameter transformation to solver parameter space
if(parameterTransformation != null) initialParameters = parameterTransformation.getSolverParameter(parameterAggregate.getParameter());
else initialParameters = parameterAggregate.getParameter();
final RandomVariableInterface[] zeros = new RandomVariableInterface[calibrationProducts.size()];
final RandomVariableInterface[] ones = new RandomVariableInterface[calibrationProducts.size()];
final RandomVariableInterface[] lowerBound = new RandomVariableInterface[initialParameters.length];
final RandomVariableInterface[] upperBound = new RandomVariableInterface[initialParameters.length];
java.util.Arrays.fill(zeros, new RandomVariable(0.0));
java.util.Arrays.fill(ones, new RandomVariable(1.0));
java.util.Arrays.fill(lowerBound, new RandomVariable(Double.NEGATIVE_INFINITY));
java.util.Arrays.fill(upperBound, new RandomVariable(Double.POSITIVE_INFINITY));
StochasticOptimizerInterface.ObjectiveFunction objectiveFunction = new StochasticOptimizerInterface.ObjectiveFunction() {
public void setValues(RandomVariableInterface[] parameters, RandomVariableInterface[] values) throws SolverException {
RandomVariableInterface[] modelParameters = parameters;
try {
if(parameterTransformation != null) {
modelParameters = parameterTransformation.getParameter(parameters);
// Copy back the parameter constrain to inform the optimizer
System.arraycopy(parameterTransformation.getSolverParameter(modelParameters), 0, parameters, 0, parameters.length);
}
Map curvesParameterPairs = parameterAggregate.getObjectsToModifyForParameter(modelParameters);
AnalyticModelInterface modelClone = model.getCloneForParameter(curvesParameterPairs);
for(int i=0; i curvesParameterPairs = parameterAggregate.getObjectsToModifyForParameter(bestParameters);
calibratedModel = model.getCloneForParameter(curvesParameterPairs);
} catch (CloneNotSupportedException e) {
throw new SolverException(e);
}
accuracy = 0.0;
for(int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy