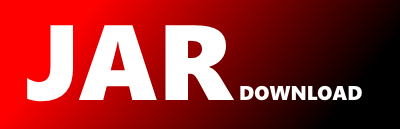
net.finmath.rootfinder.TestRootFinders Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finmath-lib Show documentation
Show all versions of finmath-lib Show documentation
finmath lib is a Mathematical Finance Library in Java.
It provides algorithms and methodologies related to mathematical finance.
/*
* Created on 02.12.2004
*
* (c) Copyright Christian P. Fries, Germany.
* Contact: [email protected].
*/
package net.finmath.rootfinder;
import java.text.DecimalFormat;
/**
* @author Christian Fries
* @version 1.1
* @date 2008-04-06
*/
public class TestRootFinders {
public static void main(String[] args) {
System.out.println("Applying root finders to x^3 + 2*y^2 + x + 1 = 0\n");
System.out.println("Root finders without derivative:");
System.out.println("--------------------------------");
RootFinder rootFinder;
rootFinder = new BisectionSearch(-10.0,10.0);
testRootFinder(rootFinder);
rootFinder = new RiddersMethod(-10.0,10.0);
testRootFinder(rootFinder);
rootFinder = new SecantMethod(2.0,10.0);
testRootFinder(rootFinder);
System.out.println("");
System.out.println("Root finders with derivative:");
System.out.println("--------------------------------");
RootFinderWithDerivative rootFinderWithDerivative;
rootFinderWithDerivative = new NewtonsMethod(2.0);
testRootFinderWithDerivative(rootFinderWithDerivative);
rootFinderWithDerivative = new SecantMethod(2.0,10.0);
testRootFinderWithDerivative(rootFinderWithDerivative);
}
public static void testRootFinder(RootFinder rootFinder) {
System.out.println("Testing " + rootFinder.getClass().getName() + ":");
// Find a solution to x^3 + x^2 + x + 1 = 0
while(rootFinder.getAccuracy() > 1E-11 && !rootFinder.isDone()) {
double x = rootFinder.getNextPoint();
// Our test function. Analytic solution is -1.0.
double y = x*x*x + x*x + x + 1;
rootFinder.setValue(y);
}
// Print result:
DecimalFormat formatter = new DecimalFormat("0.00E00");
System.out.print("Root......: "+formatter.format(rootFinder.getBestPoint())+"\t");
System.out.print("Accuracy..: "+formatter.format(rootFinder.getAccuracy() )+"\t");
System.out.print("Iterations: "+rootFinder.getNumberOfIterations() +"\n");
}
public static void testRootFinderWithDerivative(
RootFinderWithDerivative rootFinder) {
System.out.println("Testing " + rootFinder.getClass().getName() + ":");
// Find a solution to x^3 + x^2 + x + 1 = 0
while(rootFinder.getAccuracy() > 1E-11 && !rootFinder.isDone()) {
double x = rootFinder.getNextPoint();
double y = x*x*x + x*x + x + 1;
double p = 3*x*x + 2*x + 1;
rootFinder.setValueAndDerivative(y,p);
}
// Print result:
DecimalFormat formatter = new DecimalFormat("0.00E00");
System.out.print("Root......: "+formatter.format(rootFinder.getBestPoint())+"\t");
System.out.print("Accuracy..: "+formatter.format(rootFinder.getAccuracy() )+"\t");
System.out.print("Iterations: "+rootFinder.getNumberOfIterations() +"\n");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy