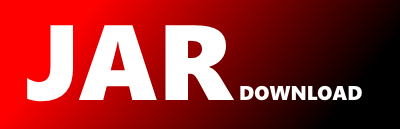
net.finmath.time.TimeDiscretization Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finmath-lib Show documentation
Show all versions of finmath-lib Show documentation
finmath lib is a Mathematical Finance Library in Java.
It provides algorithms and methodologies related to mathematical finance.
/* * (c) Copyright Christian P. Fries, Germany. Contact: [email protected]. * * Created on 20.04.2008 */ package net.finmath.time; import java.io.Serializable; import java.util.ArrayList; import java.util.Arrays; import java.util.Iterator; import java.util.Set; /** * This class represents a set of discrete points in time. *
* * @param initial First discretization point. * @param numberOfTimeSteps Number of time steps. * @param deltaT Time step size. */ public TimeDiscretization(double initial, int numberOfTimeSteps, double deltaT) { super(); timeDiscretization = new double[numberOfTimeSteps+1]; for(int timeIndex=0; timeIndex
* It handles the mapping from time indices to time points and back. * It uses a time tick size ("quantum"). This is to make comparison of times safe. * The default tick size is 1.0 / (365.0 * 24.0) (which corresponds to one hour if 1.0 is a non-leap-year): * Times are rounded to the nearest multiple of 1.0 / (365.0 * 24.0). * * This property can be configured via a System.setProperty("net.finmath.functions.TimeDiscretization.timeTickSize"). * * Objects of this class are immutable. * * @author Christian Fries * @version 1.6 */ public class TimeDiscretization implements Serializable, TimeDiscretizationInterface { private static final long serialVersionUID = 6880668325019167781L; private final static double timeTickSizeDefault = Double.parseDouble(System.getProperty("net.finmath.functions.TimeDiscretization.timeTickSize", new Double(1.0 / (365.0 * 24.0)).toString()));; private final double[] timeDiscretization; private final double timeTickSize = timeTickSizeDefault; public enum ShortPeriodLocation { SHORT_PERIOD_AT_START, SHORT_PERIOD_AT_END } /** * Constructs a time discretization from a given set of doubles. * The given array does not need to be sorted. * * @param times Given array or arguments list of discretization points. */ public TimeDiscretization(double... times) { super(); this.timeDiscretization = times.clone(); java.util.Arrays.sort(this.timeDiscretization); } /** * Constructs a time discretization from a given set of Doubles. * The given array does not need to be sorted. * * @param times Given array or arguments list of discretization points. */ public TimeDiscretization(Double[] times) { super(); this.timeDiscretization = new double[times.length]; for(int timeIndex=0; timeIndextimeDiscretization) { super(); this.timeDiscretization = new double[timeDiscretization.size()]; for(int timeIndex=0; timeIndex times) { super(); this.timeDiscretization = new double[times.size()]; Iterator time = times.iterator(); for(int timeIndex=0; timeIndex for(i=0; i ≤ timeSteps; i++) timeDiscretization[i] = initial + i * deltaT; getAsArrayList() { ArrayList times = new ArrayList (timeDiscretization.length); for (double aTimeDiscretization : timeDiscretization) times.add(aTimeDiscretization); return times; } @Override public TimeDiscretizationInterface getTimeShiftedTimeDiscretization(double timeShift) { double[] newTimeDiscretization = new double[timeDiscretization.length]; for(int timeIndex=0; timeIndex iterator() { return this.getAsArrayList().iterator(); } @Override public String toString() { return "TimeDiscretization [timeDiscretization=" + Arrays.toString(timeDiscretization) + ", timeTickSize=" + timeTickSize + "]"; } @Override public int hashCode() { final int prime = 31; int result = 1; result = prime * result + Arrays.hashCode(timeDiscretization); long temp; temp = Double.doubleToLongBits(timeTickSize); result = prime * result + (int) (temp ^ (temp >>> 32)); return result; } @Override public boolean equals(Object obj) { if (this == obj) return true; if (obj == null) return false; if (getClass() != obj.getClass()) return false; TimeDiscretization other = (TimeDiscretization) obj; if (!Arrays.equals(timeDiscretization, other.timeDiscretization)) return false; if (Double.doubleToLongBits(timeTickSize) != Double.doubleToLongBits(other.timeTickSize)) return false; return true; } private double roundToTimeTickSize(double time) { return Math.rint(time/timeTickSize)*timeTickSize; } }
© 2015 - 2025 Weber Informatics LLC | Privacy Policy