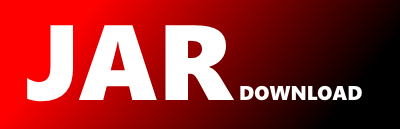
net.finmath.smartcontract.api.PlainSwapEditorApi Maven / Gradle / Ivy
/**
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech) (6.4.0).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package net.finmath.smartcontract.api;
import net.finmath.smartcontract.model.CashflowPeriod;
import net.finmath.smartcontract.model.Error;
import net.finmath.smartcontract.model.MarketDataSet;
import net.finmath.smartcontract.model.PlainSwapOperationRequest;
import net.finmath.smartcontract.model.SaveContractRequest;
import net.finmath.smartcontract.model.ValueResult;
import io.swagger.v3.oas.annotations.ExternalDocumentation;
import io.swagger.v3.oas.annotations.Operation;
import io.swagger.v3.oas.annotations.Parameter;
import io.swagger.v3.oas.annotations.Parameters;
import io.swagger.v3.oas.annotations.media.ArraySchema;
import io.swagger.v3.oas.annotations.media.Content;
import io.swagger.v3.oas.annotations.media.Schema;
import io.swagger.v3.oas.annotations.responses.ApiResponse;
import io.swagger.v3.oas.annotations.security.SecurityRequirement;
import io.swagger.v3.oas.annotations.tags.Tag;
import io.swagger.v3.oas.annotations.enums.ParameterIn;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.validation.annotation.Validated;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.context.request.NativeWebRequest;
import org.springframework.web.multipart.MultipartFile;
import jakarta.validation.Valid;
import jakarta.validation.constraints.*;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import jakarta.annotation.Generated;
@Generated(value = "org.openapitools.codegen.languages.SpringCodegen", date = "2024-12-05T10:54:28.421239+01:00[Europe/Berlin]")
@Validated
@Tag(name = "plainSwapEditor", description = "the plainSwapEditor API")
public interface PlainSwapEditorApi {
default Optional getRequest() {
return Optional.empty();
}
/**
* POST /plain-swap-editor/change-dataset : Request a saved contract.
*
* @param body (optional)
* @return Fetch successful. (status code 200)
* or unexpected error (status code 200)
*/
@Operation(
operationId = "changeDataset",
summary = "Request a saved contract.",
tags = { "plainSwapEditor" },
responses = {
@ApiResponse(responseCode = "200", description = "Fetch successful.", content = {
@Content(mediaType = "text/plain", schema = @Schema(implementation = String.class)),
@Content(mediaType = "application/json", schema = @Schema(implementation = String.class))
}),
@ApiResponse(responseCode = "default", description = "unexpected error", content = {
@Content(mediaType = "text/plain", schema = @Schema(implementation = Error.class)),
@Content(mediaType = "application/json", schema = @Schema(implementation = Error.class))
})
}
)
@RequestMapping(
method = RequestMethod.POST,
value = "/plain-swap-editor/change-dataset",
produces = { "text/plain", "application/json" },
consumes = { "application/json" }
)
default ResponseEntity _changeDataset(
@Parameter(name = "body", description = "") @Valid @RequestBody(required = false) String body
) {
return changeDataset(body);
}
// Override this method
default ResponseEntity changeDataset(String body) {
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
/**
* POST /plain-swap-editor/evaluate-from-editor : Request mapping for valuation of the trade data coming from the editor.
*
* @param plainSwapOperationRequest (optional)
* @return Valuation successful. (status code 200)
* or unexpected error (status code 200)
*/
@Operation(
operationId = "evaluateFromPlainSwapEditor",
summary = "Request mapping for valuation of the trade data coming from the editor.",
tags = { "plainSwapEditor" },
responses = {
@ApiResponse(responseCode = "200", description = "Valuation successful.", content = {
@Content(mediaType = "application/json", schema = @Schema(implementation = ValueResult.class))
}),
@ApiResponse(responseCode = "default", description = "unexpected error", content = {
@Content(mediaType = "application/json", schema = @Schema(implementation = Error.class))
})
}
)
@RequestMapping(
method = RequestMethod.POST,
value = "/plain-swap-editor/evaluate-from-editor",
produces = { "application/json" },
consumes = { "application/json" }
)
default ResponseEntity _evaluateFromPlainSwapEditor(
@Parameter(name = "PlainSwapOperationRequest", description = "") @Valid @RequestBody(required = false) PlainSwapOperationRequest plainSwapOperationRequest
) {
return evaluateFromPlainSwapEditor(plainSwapOperationRequest);
}
// Override this method
default ResponseEntity evaluateFromPlainSwapEditor(PlainSwapOperationRequest plainSwapOperationRequest) {
getRequest().ifPresent(request -> {
for (MediaType mediaType: MediaType.parseMediaTypes(request.getHeader("Accept"))) {
if (mediaType.isCompatibleWith(MediaType.valueOf("application/json"))) {
String exampleString = "{ \"valuationDate\" : \"valuationDate\", \"currency\" : \"currency\", \"value\" : 0.8008281904610115 }";
ApiUtil.setExampleResponse(request, "application/json", exampleString);
break;
}
}
});
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
/**
* POST /plain-swap-editor/generate-xml : Request mapping for generation of the trade data SDCmL
*
* @param plainSwapOperationRequest (optional)
* @return SDCmL was generated (status code 200)
* or unexpected error (status code 200)
*/
@Operation(
operationId = "generatePlainSwapSdcml",
summary = "Request mapping for generation of the trade data SDCmL",
tags = { "plainSwapEditor" },
responses = {
@ApiResponse(responseCode = "200", description = "SDCmL was generated", content = {
@Content(mediaType = "text/plain", schema = @Schema(implementation = String.class)),
@Content(mediaType = "application/json", schema = @Schema(implementation = String.class))
}),
@ApiResponse(responseCode = "default", description = "unexpected error", content = {
@Content(mediaType = "text/plain", schema = @Schema(implementation = Error.class)),
@Content(mediaType = "application/json", schema = @Schema(implementation = Error.class))
})
}
)
@RequestMapping(
method = RequestMethod.POST,
value = "/plain-swap-editor/generate-xml",
produces = { "text/plain", "application/json" },
consumes = { "application/json" }
)
default ResponseEntity _generatePlainSwapSdcml(
@Parameter(name = "PlainSwapOperationRequest", description = "") @Valid @RequestBody(required = false) PlainSwapOperationRequest plainSwapOperationRequest
) {
return generatePlainSwapSdcml(plainSwapOperationRequest);
}
// Override this method
default ResponseEntity generatePlainSwapSdcml(PlainSwapOperationRequest plainSwapOperationRequest) {
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
/**
* POST /plain-swap-editor/get-fixed-schedule : Request payment schedule for the fixed leg defined in the editor.
*
* @param plainSwapOperationRequest (optional)
* @return Schedule generation successful. (status code 200)
* or unexpected error (status code 200)
*/
@Operation(
operationId = "getFixedSchedule",
summary = "Request payment schedule for the fixed leg defined in the editor.",
tags = { "plainSwapEditor" },
responses = {
@ApiResponse(responseCode = "200", description = "Schedule generation successful.", content = {
@Content(mediaType = "application/json", array = @ArraySchema(schema = @Schema(implementation = CashflowPeriod.class)))
}),
@ApiResponse(responseCode = "default", description = "unexpected error", content = {
@Content(mediaType = "application/json", schema = @Schema(implementation = Error.class))
})
}
)
@RequestMapping(
method = RequestMethod.POST,
value = "/plain-swap-editor/get-fixed-schedule",
produces = { "application/json" },
consumes = { "application/json" }
)
default ResponseEntity> _getFixedSchedule(
@Parameter(name = "PlainSwapOperationRequest", description = "") @Valid @RequestBody(required = false) PlainSwapOperationRequest plainSwapOperationRequest
) {
return getFixedSchedule(plainSwapOperationRequest);
}
// Override this method
default ResponseEntity> getFixedSchedule(PlainSwapOperationRequest plainSwapOperationRequest) {
getRequest().ifPresent(request -> {
for (MediaType mediaType: MediaType.parseMediaTypes(request.getHeader("Accept"))) {
if (mediaType.isCompatibleWith(MediaType.valueOf("application/json"))) {
String exampleString = "[ { \"rate\" : 0.8008281904610115, \"cashflow\" : { \"valuationDate\" : \"valuationDate\", \"currency\" : \"currency\", \"value\" : 0.8008281904610115 }, \"fixingDate\" : \"2000-01-23T04:56:07.000+00:00\", \"paymentDate\" : \"2000-01-23T04:56:07.000+00:00\", \"periodStart\" : \"2000-01-23T04:56:07.000+00:00\", \"periodEnd\" : \"2000-01-23T04:56:07.000+00:00\" }, { \"rate\" : 0.8008281904610115, \"cashflow\" : { \"valuationDate\" : \"valuationDate\", \"currency\" : \"currency\", \"value\" : 0.8008281904610115 }, \"fixingDate\" : \"2000-01-23T04:56:07.000+00:00\", \"paymentDate\" : \"2000-01-23T04:56:07.000+00:00\", \"periodStart\" : \"2000-01-23T04:56:07.000+00:00\", \"periodEnd\" : \"2000-01-23T04:56:07.000+00:00\" } ]";
ApiUtil.setExampleResponse(request, "application/json", exampleString);
break;
}
}
});
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
/**
* POST /plain-swap-editor/get-floating-schedule : Request payment schedule for the floating leg defined in the editor.
*
* @param plainSwapOperationRequest (optional)
* @return Schedule generation successful. (status code 200)
* or unexpected error (status code 200)
*/
@Operation(
operationId = "getFloatingSchedule",
summary = "Request payment schedule for the floating leg defined in the editor.",
tags = { "plainSwapEditor" },
responses = {
@ApiResponse(responseCode = "200", description = "Schedule generation successful.", content = {
@Content(mediaType = "application/json", array = @ArraySchema(schema = @Schema(implementation = CashflowPeriod.class)))
}),
@ApiResponse(responseCode = "default", description = "unexpected error", content = {
@Content(mediaType = "application/json", schema = @Schema(implementation = Error.class))
})
}
)
@RequestMapping(
method = RequestMethod.POST,
value = "/plain-swap-editor/get-floating-schedule",
produces = { "application/json" },
consumes = { "application/json" }
)
default ResponseEntity> _getFloatingSchedule(
@Parameter(name = "PlainSwapOperationRequest", description = "") @Valid @RequestBody(required = false) PlainSwapOperationRequest plainSwapOperationRequest
) {
return getFloatingSchedule(plainSwapOperationRequest);
}
// Override this method
default ResponseEntity> getFloatingSchedule(PlainSwapOperationRequest plainSwapOperationRequest) {
getRequest().ifPresent(request -> {
for (MediaType mediaType: MediaType.parseMediaTypes(request.getHeader("Accept"))) {
if (mediaType.isCompatibleWith(MediaType.valueOf("application/json"))) {
String exampleString = "[ { \"rate\" : 0.8008281904610115, \"cashflow\" : { \"valuationDate\" : \"valuationDate\", \"currency\" : \"currency\", \"value\" : 0.8008281904610115 }, \"fixingDate\" : \"2000-01-23T04:56:07.000+00:00\", \"paymentDate\" : \"2000-01-23T04:56:07.000+00:00\", \"periodStart\" : \"2000-01-23T04:56:07.000+00:00\", \"periodEnd\" : \"2000-01-23T04:56:07.000+00:00\" }, { \"rate\" : 0.8008281904610115, \"cashflow\" : { \"valuationDate\" : \"valuationDate\", \"currency\" : \"currency\", \"value\" : 0.8008281904610115 }, \"fixingDate\" : \"2000-01-23T04:56:07.000+00:00\", \"paymentDate\" : \"2000-01-23T04:56:07.000+00:00\", \"periodStart\" : \"2000-01-23T04:56:07.000+00:00\", \"periodEnd\" : \"2000-01-23T04:56:07.000+00:00\" } ]";
ApiUtil.setExampleResponse(request, "application/json", exampleString);
break;
}
}
});
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
/**
* POST /plain-swap-editor/get-par-rate : Request the par rate for the swap defined in the editor.
*
* @param plainSwapOperationRequest (optional)
* @return Schedule generation successful. (status code 200)
* or unexpected error (status code 200)
*/
@Operation(
operationId = "getParRate",
summary = "Request the par rate for the swap defined in the editor.",
tags = { "plainSwapEditor" },
responses = {
@ApiResponse(responseCode = "200", description = "Schedule generation successful.", content = {
@Content(mediaType = "application/json", schema = @Schema(implementation = Double.class))
}),
@ApiResponse(responseCode = "default", description = "unexpected error", content = {
@Content(mediaType = "application/json", schema = @Schema(implementation = Error.class))
})
}
)
@RequestMapping(
method = RequestMethod.POST,
value = "/plain-swap-editor/get-par-rate",
produces = { "application/json" },
consumes = { "application/json" }
)
default ResponseEntity _getParRate(
@Parameter(name = "PlainSwapOperationRequest", description = "") @Valid @RequestBody(required = false) PlainSwapOperationRequest plainSwapOperationRequest
) {
return getParRate(plainSwapOperationRequest);
}
// Override this method
default ResponseEntity getParRate(PlainSwapOperationRequest plainSwapOperationRequest) {
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
/**
* GET /plain-swap-editor/get-saved-contracts : Request the list of saved contracts.
*
* @return Fetch successful. (status code 200)
* or unexpected error (status code 200)
*/
@Operation(
operationId = "getSavedContracts",
summary = "Request the list of saved contracts.",
tags = { "plainSwapEditor" },
responses = {
@ApiResponse(responseCode = "200", description = "Fetch successful.", content = {
@Content(mediaType = "application/json", array = @ArraySchema(schema = @Schema(implementation = String.class)))
}),
@ApiResponse(responseCode = "default", description = "unexpected error", content = {
@Content(mediaType = "application/json", array = @ArraySchema(schema = @Schema(implementation = String.class)))
})
}
)
@RequestMapping(
method = RequestMethod.GET,
value = "/plain-swap-editor/get-saved-contracts",
produces = { "application/json" }
)
default ResponseEntity> _getSavedContracts(
) {
return getSavedContracts();
}
// Override this method
default ResponseEntity> getSavedContracts() {
getRequest().ifPresent(request -> {
for (MediaType mediaType: MediaType.parseMediaTypes(request.getHeader("Accept"))) {
if (mediaType.isCompatibleWith(MediaType.valueOf("application/json"))) {
String exampleString = "[ \"\", \"\" ]";
ApiUtil.setExampleResponse(request, "application/json", exampleString);
break;
}
}
});
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
/**
* GET /plain-swap-editor/get-saved-market-data : Request the list of saved market data.
*
* @return Fetch successful. (status code 200)
* or unexpected error (status code 200)
*/
@Operation(
operationId = "getSavedMarketData",
summary = "Request the list of saved market data.",
tags = { "plainSwapEditor" },
responses = {
@ApiResponse(responseCode = "200", description = "Fetch successful.", content = {
@Content(mediaType = "application/json", array = @ArraySchema(schema = @Schema(implementation = String.class)))
}),
@ApiResponse(responseCode = "default", description = "unexpected error", content = {
@Content(mediaType = "application/json", array = @ArraySchema(schema = @Schema(implementation = String.class)))
})
}
)
@RequestMapping(
method = RequestMethod.GET,
value = "/plain-swap-editor/get-saved-market-data",
produces = { "application/json" }
)
default ResponseEntity> _getSavedMarketData(
) {
return getSavedMarketData();
}
// Override this method
default ResponseEntity> getSavedMarketData() {
getRequest().ifPresent(request -> {
for (MediaType mediaType: MediaType.parseMediaTypes(request.getHeader("Accept"))) {
if (mediaType.isCompatibleWith(MediaType.valueOf("application/json"))) {
String exampleString = "[ \"\", \"\" ]";
ApiUtil.setExampleResponse(request, "application/json", exampleString);
break;
}
}
});
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
/**
* GET /plain-swap-editor/grab-market-data : Request mapping for transferring the active dataset to the client
*
* @return Valuation successful. (status code 200)
* or unexpected error (status code 200)
*/
@Operation(
operationId = "grabMarketData",
summary = "Request mapping for transferring the active dataset to the client",
tags = { "plainSwapEditor" },
responses = {
@ApiResponse(responseCode = "200", description = "Valuation successful.", content = {
@Content(mediaType = "application/json", schema = @Schema(implementation = MarketDataSet.class))
}),
@ApiResponse(responseCode = "default", description = "unexpected error", content = {
@Content(mediaType = "application/json", schema = @Schema(implementation = Error.class))
})
}
)
@RequestMapping(
method = RequestMethod.GET,
value = "/plain-swap-editor/grab-market-data",
produces = { "application/json" }
)
default ResponseEntity _grabMarketData(
) {
return grabMarketData();
}
// Override this method
default ResponseEntity grabMarketData() {
getRequest().ifPresent(request -> {
for (MediaType mediaType: MediaType.parseMediaTypes(request.getHeader("Accept"))) {
if (mediaType.isCompatibleWith(MediaType.valueOf("application/json"))) {
String exampleString = "{ \"requestTimestamp\" : \"2000-01-23T04:56:07.000+00:00\", \"values\" : [ { \"symbol\" : \"symbol\", \"dataTimestamp\" : \"2000-01-23T04:56:07.000+00:00\", \"value\" : 0.8008281904610115 }, { \"symbol\" : \"symbol\", \"dataTimestamp\" : \"2000-01-23T04:56:07.000+00:00\", \"value\" : 0.8008281904610115 } ] }";
ApiUtil.setExampleResponse(request, "application/json", exampleString);
break;
}
}
});
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
/**
* POST /plain-swap-editor/load-contract : Request a saved contract.
*
* @param body (optional)
* @return Fetch successful. (status code 200)
* or unexpected error (status code 200)
*/
@Operation(
operationId = "loadContract",
summary = "Request a saved contract.",
tags = { "plainSwapEditor" },
responses = {
@ApiResponse(responseCode = "200", description = "Fetch successful.", content = {
@Content(mediaType = "application/json", schema = @Schema(implementation = PlainSwapOperationRequest.class))
}),
@ApiResponse(responseCode = "default", description = "unexpected error", content = {
@Content(mediaType = "application/json", schema = @Schema(implementation = Error.class))
})
}
)
@RequestMapping(
method = RequestMethod.POST,
value = "/plain-swap-editor/load-contract",
produces = { "application/json" },
consumes = { "application/json" }
)
default ResponseEntity _loadContract(
@Parameter(name = "body", description = "") @Valid @RequestBody(required = false) String body
) {
return loadContract(body);
}
// Override this method
default ResponseEntity loadContract(String body) {
getRequest().ifPresent(request -> {
for (MediaType mediaType: MediaType.parseMediaTypes(request.getHeader("Accept"))) {
if (mediaType.isCompatibleWith(MediaType.valueOf("application/json"))) {
String exampleString = "{ \"notionalAmount\" : 0.14658129805029452, \"floatingPaymentFrequency\" : { \"period\" : \"period\", \"fullName\" : \"fullName\", \"periodMultiplier\" : 1 }, \"currentGenerator\" : \"currentGenerator\", \"valuationSymbols\" : [ { \"symbol\" : \"symbol\", \"itemType\" : \"itemType\", \"tenor\" : \"tenor\", \"curve\" : \"curve\" }, { \"symbol\" : \"symbol\", \"itemType\" : \"itemType\", \"tenor\" : \"tenor\", \"curve\" : \"curve\" } ], \"terminationFeeAmount\" : 0.6027456183070403, \"fixedDayCountFraction\" : \"fixedDayCountFraction\", \"tradeDate\" : \"2000-01-23T04:56:07.000+00:00\", \"firstCounterparty\" : { \"baseUrl\" : \"http://example.com/aeiou\", \"fullName\" : \"fullName\", \"bicCode\" : \"bicCode\" }, \"secondCounterparty\" : { \"baseUrl\" : \"http://example.com/aeiou\", \"fullName\" : \"fullName\", \"bicCode\" : \"bicCode\" }, \"floatingPayingParty\" : { \"baseUrl\" : \"http://example.com/aeiou\", \"fullName\" : \"fullName\", \"bicCode\" : \"bicCode\" }, \"terminationDate\" : \"2000-01-23T04:56:07.000+00:00\", \"fixedPaymentFrequency\" : { \"period\" : \"period\", \"fullName\" : \"fullName\", \"periodMultiplier\" : 1 }, \"uniqueTradeIdentifier\" : \"uniqueTradeIdentifier\", \"marginBufferAmount\" : 0.08008281904610115, \"fixedPayingParty\" : { \"baseUrl\" : \"http://example.com/aeiou\", \"fullName\" : \"fullName\", \"bicCode\" : \"bicCode\" }, \"fixedRate\" : 5.962133916683182, \"currency\" : \"currency\", \"floatingDayCountFraction\" : \"floatingDayCountFraction\", \"floatingFixingDayOffset\" : -1, \"effectiveDate\" : \"2000-01-23T04:56:07.000+00:00\", \"floatingRateIndex\" : \"floatingRateIndex\" }";
ApiUtil.setExampleResponse(request, "application/json", exampleString);
break;
}
}
});
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
/**
* POST /plain-swap-editor/refresh-market-data : Request mapping for valuation of the trade data coming from the editor.
*
* @param plainSwapOperationRequest (optional)
* @return Valuation successful. (status code 200)
* or unexpected error (status code 200)
*/
@Operation(
operationId = "refreshMarketData",
summary = "Request mapping for valuation of the trade data coming from the editor.",
tags = { "plainSwapEditor" },
responses = {
@ApiResponse(responseCode = "200", description = "Valuation successful.", content = {
@Content(mediaType = "application/json", schema = @Schema(implementation = ValueResult.class))
}),
@ApiResponse(responseCode = "default", description = "unexpected error", content = {
@Content(mediaType = "application/json", schema = @Schema(implementation = Error.class))
})
}
)
@RequestMapping(
method = RequestMethod.POST,
value = "/plain-swap-editor/refresh-market-data",
produces = { "application/json" },
consumes = { "application/json" }
)
default ResponseEntity _refreshMarketData(
@Parameter(name = "PlainSwapOperationRequest", description = "") @Valid @RequestBody(required = false) PlainSwapOperationRequest plainSwapOperationRequest
) {
return refreshMarketData(plainSwapOperationRequest);
}
// Override this method
default ResponseEntity refreshMarketData(PlainSwapOperationRequest plainSwapOperationRequest) {
getRequest().ifPresent(request -> {
for (MediaType mediaType: MediaType.parseMediaTypes(request.getHeader("Accept"))) {
if (mediaType.isCompatibleWith(MediaType.valueOf("application/json"))) {
String exampleString = "{ \"valuationDate\" : \"valuationDate\", \"currency\" : \"currency\", \"value\" : 0.8008281904610115 }";
ApiUtil.setExampleResponse(request, "application/json", exampleString);
break;
}
}
});
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
/**
* POST /plain-swap-editor/save-contract : Request to save a contract.
*
* @param saveContractRequest (optional)
* @return Save successful. (status code 200)
* or unexpected error (status code 200)
*/
@Operation(
operationId = "saveContract",
summary = "Request to save a contract.",
tags = { "plainSwapEditor" },
responses = {
@ApiResponse(responseCode = "200", description = "Save successful.", content = {
@Content(mediaType = "text/plain", schema = @Schema(implementation = String.class)),
@Content(mediaType = "application/json", schema = @Schema(implementation = String.class))
}),
@ApiResponse(responseCode = "default", description = "unexpected error", content = {
@Content(mediaType = "text/plain", schema = @Schema(implementation = Error.class)),
@Content(mediaType = "application/json", schema = @Schema(implementation = Error.class))
})
}
)
@RequestMapping(
method = RequestMethod.POST,
value = "/plain-swap-editor/save-contract",
produces = { "text/plain", "application/json" },
consumes = { "application/json" }
)
default ResponseEntity _saveContract(
@Parameter(name = "SaveContractRequest", description = "") @Valid @RequestBody(required = false) SaveContractRequest saveContractRequest
) {
return saveContract(saveContractRequest);
}
// Override this method
default ResponseEntity saveContract(SaveContractRequest saveContractRequest) {
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
/**
* POST /plain-swap-editor/upload-market-data : Request to upload market data
*
* @param tradeData (required)
* @return Upload successful. (status code 200)
* or unexpected error (status code 200)
*/
@Operation(
operationId = "uploadMarketData",
summary = "Request to upload market data",
tags = { "plainSwapEditor" },
responses = {
@ApiResponse(responseCode = "200", description = "Upload successful.", content = {
@Content(mediaType = "text/plain", schema = @Schema(implementation = String.class)),
@Content(mediaType = "application/json", schema = @Schema(implementation = String.class))
}),
@ApiResponse(responseCode = "default", description = "unexpected error", content = {
@Content(mediaType = "text/plain", schema = @Schema(implementation = Error.class)),
@Content(mediaType = "application/json", schema = @Schema(implementation = Error.class))
})
}
)
@RequestMapping(
method = RequestMethod.POST,
value = "/plain-swap-editor/upload-market-data",
produces = { "text/plain", "application/json" },
consumes = { "multipart/form-data" }
)
default ResponseEntity _uploadMarketData(
@Parameter(name = "tradeData", description = "", required = true) @RequestPart(value = "tradeData", required = true) MultipartFile tradeData
) {
return uploadMarketData(tradeData);
}
// Override this method
default ResponseEntity uploadMarketData(MultipartFile tradeData) {
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy