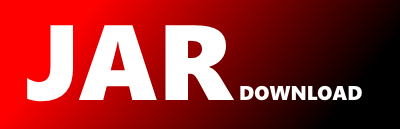
net.finmath.smartcontract.model.PlainSwapOperationRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finmath-smart-derivative-contract Show documentation
Show all versions of finmath-smart-derivative-contract Show documentation
Project to support the implementation a of smart derivative contract.
package net.finmath.smartcontract.model;
import java.net.URI;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
import net.finmath.smartcontract.model.Counterparty;
import net.finmath.smartcontract.model.FrontendItemSpec;
import net.finmath.smartcontract.model.PaymentFrequency;
import org.springframework.format.annotation.DateTimeFormat;
import org.openapitools.jackson.nullable.JsonNullable;
import java.time.OffsetDateTime;
import jakarta.validation.Valid;
import jakarta.validation.constraints.*;
import io.swagger.v3.oas.annotations.media.Schema;
import java.util.*;
import jakarta.annotation.Generated;
/**
* PlainSwapOperationRequest
*/
@Generated(value = "org.openapitools.codegen.languages.SpringCodegen", date = "2024-12-05T10:54:28.421239+01:00[Europe/Berlin]")
public class PlainSwapOperationRequest {
@JsonProperty("firstCounterparty")
private Counterparty firstCounterparty;
@JsonProperty("secondCounterparty")
private Counterparty secondCounterparty;
@JsonProperty("marginBufferAmount")
private Double marginBufferAmount;
@JsonProperty("terminationFeeAmount")
private Double terminationFeeAmount;
@JsonProperty("notionalAmount")
private Double notionalAmount;
@JsonProperty("currency")
private String currency;
@JsonProperty("uniqueTradeIdentifier")
private String uniqueTradeIdentifier;
@JsonProperty("tradeDate")
@DateTimeFormat(iso = DateTimeFormat.ISO.DATE_TIME)
private OffsetDateTime tradeDate;
@JsonProperty("effectiveDate")
@DateTimeFormat(iso = DateTimeFormat.ISO.DATE_TIME)
private OffsetDateTime effectiveDate;
@JsonProperty("terminationDate")
@DateTimeFormat(iso = DateTimeFormat.ISO.DATE_TIME)
private OffsetDateTime terminationDate;
@JsonProperty("fixedPayingParty")
private Counterparty fixedPayingParty;
@JsonProperty("fixedRate")
private Double fixedRate;
@JsonProperty("fixedDayCountFraction")
private String fixedDayCountFraction;
@JsonProperty("fixedPaymentFrequency")
private PaymentFrequency fixedPaymentFrequency;
@JsonProperty("floatingPayingParty")
private Counterparty floatingPayingParty;
@JsonProperty("floatingRateIndex")
private String floatingRateIndex;
@JsonProperty("floatingDayCountFraction")
private String floatingDayCountFraction;
@JsonProperty("floatingFixingDayOffset")
private Integer floatingFixingDayOffset;
@JsonProperty("floatingPaymentFrequency")
private PaymentFrequency floatingPaymentFrequency;
@JsonProperty("valuationSymbols")
@Valid
private List valuationSymbols = new ArrayList<>();
@JsonProperty("currentGenerator")
private String currentGenerator;
public PlainSwapOperationRequest firstCounterparty(Counterparty firstCounterparty) {
this.firstCounterparty = firstCounterparty;
return this;
}
/**
* Get firstCounterparty
* @return firstCounterparty
*/
@NotNull @Valid
@Schema(name = "firstCounterparty", requiredMode = Schema.RequiredMode.REQUIRED)
public Counterparty getFirstCounterparty() {
return firstCounterparty;
}
public void setFirstCounterparty(Counterparty firstCounterparty) {
this.firstCounterparty = firstCounterparty;
}
public PlainSwapOperationRequest secondCounterparty(Counterparty secondCounterparty) {
this.secondCounterparty = secondCounterparty;
return this;
}
/**
* Get secondCounterparty
* @return secondCounterparty
*/
@NotNull @Valid
@Schema(name = "secondCounterparty", requiredMode = Schema.RequiredMode.REQUIRED)
public Counterparty getSecondCounterparty() {
return secondCounterparty;
}
public void setSecondCounterparty(Counterparty secondCounterparty) {
this.secondCounterparty = secondCounterparty;
}
public PlainSwapOperationRequest marginBufferAmount(Double marginBufferAmount) {
this.marginBufferAmount = marginBufferAmount;
return this;
}
/**
* Get marginBufferAmount
* minimum: 0.0
* @return marginBufferAmount
*/
@NotNull @DecimalMin("0.0")
@Schema(name = "marginBufferAmount", requiredMode = Schema.RequiredMode.REQUIRED)
public Double getMarginBufferAmount() {
return marginBufferAmount;
}
public void setMarginBufferAmount(Double marginBufferAmount) {
this.marginBufferAmount = marginBufferAmount;
}
public PlainSwapOperationRequest terminationFeeAmount(Double terminationFeeAmount) {
this.terminationFeeAmount = terminationFeeAmount;
return this;
}
/**
* Get terminationFeeAmount
* minimum: 0.0
* @return terminationFeeAmount
*/
@NotNull @DecimalMin("0.0")
@Schema(name = "terminationFeeAmount", requiredMode = Schema.RequiredMode.REQUIRED)
public Double getTerminationFeeAmount() {
return terminationFeeAmount;
}
public void setTerminationFeeAmount(Double terminationFeeAmount) {
this.terminationFeeAmount = terminationFeeAmount;
}
public PlainSwapOperationRequest notionalAmount(Double notionalAmount) {
this.notionalAmount = notionalAmount;
return this;
}
/**
* Get notionalAmount
* minimum: 0.0
* @return notionalAmount
*/
@NotNull @DecimalMin("0.0")
@Schema(name = "notionalAmount", requiredMode = Schema.RequiredMode.REQUIRED)
public Double getNotionalAmount() {
return notionalAmount;
}
public void setNotionalAmount(Double notionalAmount) {
this.notionalAmount = notionalAmount;
}
public PlainSwapOperationRequest currency(String currency) {
this.currency = currency;
return this;
}
/**
* Get currency
* @return currency
*/
@NotNull
@Schema(name = "currency", requiredMode = Schema.RequiredMode.REQUIRED)
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public PlainSwapOperationRequest uniqueTradeIdentifier(String uniqueTradeIdentifier) {
this.uniqueTradeIdentifier = uniqueTradeIdentifier;
return this;
}
/**
* Get uniqueTradeIdentifier
* @return uniqueTradeIdentifier
*/
@Schema(name = "uniqueTradeIdentifier", requiredMode = Schema.RequiredMode.NOT_REQUIRED)
public String getUniqueTradeIdentifier() {
return uniqueTradeIdentifier;
}
public void setUniqueTradeIdentifier(String uniqueTradeIdentifier) {
this.uniqueTradeIdentifier = uniqueTradeIdentifier;
}
public PlainSwapOperationRequest tradeDate(OffsetDateTime tradeDate) {
this.tradeDate = tradeDate;
return this;
}
/**
* Get tradeDate
* @return tradeDate
*/
@NotNull @Valid
@Schema(name = "tradeDate", requiredMode = Schema.RequiredMode.REQUIRED)
public OffsetDateTime getTradeDate() {
return tradeDate;
}
public void setTradeDate(OffsetDateTime tradeDate) {
this.tradeDate = tradeDate;
}
public PlainSwapOperationRequest effectiveDate(OffsetDateTime effectiveDate) {
this.effectiveDate = effectiveDate;
return this;
}
/**
* Get effectiveDate
* @return effectiveDate
*/
@NotNull @Valid
@Schema(name = "effectiveDate", requiredMode = Schema.RequiredMode.REQUIRED)
public OffsetDateTime getEffectiveDate() {
return effectiveDate;
}
public void setEffectiveDate(OffsetDateTime effectiveDate) {
this.effectiveDate = effectiveDate;
}
public PlainSwapOperationRequest terminationDate(OffsetDateTime terminationDate) {
this.terminationDate = terminationDate;
return this;
}
/**
* Get terminationDate
* @return terminationDate
*/
@NotNull @Valid
@Schema(name = "terminationDate", requiredMode = Schema.RequiredMode.REQUIRED)
public OffsetDateTime getTerminationDate() {
return terminationDate;
}
public void setTerminationDate(OffsetDateTime terminationDate) {
this.terminationDate = terminationDate;
}
public PlainSwapOperationRequest fixedPayingParty(Counterparty fixedPayingParty) {
this.fixedPayingParty = fixedPayingParty;
return this;
}
/**
* Get fixedPayingParty
* @return fixedPayingParty
*/
@NotNull @Valid
@Schema(name = "fixedPayingParty", requiredMode = Schema.RequiredMode.REQUIRED)
public Counterparty getFixedPayingParty() {
return fixedPayingParty;
}
public void setFixedPayingParty(Counterparty fixedPayingParty) {
this.fixedPayingParty = fixedPayingParty;
}
public PlainSwapOperationRequest fixedRate(Double fixedRate) {
this.fixedRate = fixedRate;
return this;
}
/**
* Get fixedRate
* @return fixedRate
*/
@NotNull
@Schema(name = "fixedRate", requiredMode = Schema.RequiredMode.REQUIRED)
public Double getFixedRate() {
return fixedRate;
}
public void setFixedRate(Double fixedRate) {
this.fixedRate = fixedRate;
}
public PlainSwapOperationRequest fixedDayCountFraction(String fixedDayCountFraction) {
this.fixedDayCountFraction = fixedDayCountFraction;
return this;
}
/**
* Get fixedDayCountFraction
* @return fixedDayCountFraction
*/
@NotNull
@Schema(name = "fixedDayCountFraction", requiredMode = Schema.RequiredMode.REQUIRED)
public String getFixedDayCountFraction() {
return fixedDayCountFraction;
}
public void setFixedDayCountFraction(String fixedDayCountFraction) {
this.fixedDayCountFraction = fixedDayCountFraction;
}
public PlainSwapOperationRequest fixedPaymentFrequency(PaymentFrequency fixedPaymentFrequency) {
this.fixedPaymentFrequency = fixedPaymentFrequency;
return this;
}
/**
* Get fixedPaymentFrequency
* @return fixedPaymentFrequency
*/
@NotNull @Valid
@Schema(name = "fixedPaymentFrequency", requiredMode = Schema.RequiredMode.REQUIRED)
public PaymentFrequency getFixedPaymentFrequency() {
return fixedPaymentFrequency;
}
public void setFixedPaymentFrequency(PaymentFrequency fixedPaymentFrequency) {
this.fixedPaymentFrequency = fixedPaymentFrequency;
}
public PlainSwapOperationRequest floatingPayingParty(Counterparty floatingPayingParty) {
this.floatingPayingParty = floatingPayingParty;
return this;
}
/**
* Get floatingPayingParty
* @return floatingPayingParty
*/
@NotNull @Valid
@Schema(name = "floatingPayingParty", requiredMode = Schema.RequiredMode.REQUIRED)
public Counterparty getFloatingPayingParty() {
return floatingPayingParty;
}
public void setFloatingPayingParty(Counterparty floatingPayingParty) {
this.floatingPayingParty = floatingPayingParty;
}
public PlainSwapOperationRequest floatingRateIndex(String floatingRateIndex) {
this.floatingRateIndex = floatingRateIndex;
return this;
}
/**
* Get floatingRateIndex
* @return floatingRateIndex
*/
@NotNull
@Schema(name = "floatingRateIndex", requiredMode = Schema.RequiredMode.REQUIRED)
public String getFloatingRateIndex() {
return floatingRateIndex;
}
public void setFloatingRateIndex(String floatingRateIndex) {
this.floatingRateIndex = floatingRateIndex;
}
public PlainSwapOperationRequest floatingDayCountFraction(String floatingDayCountFraction) {
this.floatingDayCountFraction = floatingDayCountFraction;
return this;
}
/**
* Get floatingDayCountFraction
* @return floatingDayCountFraction
*/
@NotNull
@Schema(name = "floatingDayCountFraction", requiredMode = Schema.RequiredMode.REQUIRED)
public String getFloatingDayCountFraction() {
return floatingDayCountFraction;
}
public void setFloatingDayCountFraction(String floatingDayCountFraction) {
this.floatingDayCountFraction = floatingDayCountFraction;
}
public PlainSwapOperationRequest floatingFixingDayOffset(Integer floatingFixingDayOffset) {
this.floatingFixingDayOffset = floatingFixingDayOffset;
return this;
}
/**
* Get floatingFixingDayOffset
* minimum: -2
* maximum: 2
* @return floatingFixingDayOffset
*/
@NotNull @Min(-2) @Max(2)
@Schema(name = "floatingFixingDayOffset", requiredMode = Schema.RequiredMode.REQUIRED)
public Integer getFloatingFixingDayOffset() {
return floatingFixingDayOffset;
}
public void setFloatingFixingDayOffset(Integer floatingFixingDayOffset) {
this.floatingFixingDayOffset = floatingFixingDayOffset;
}
public PlainSwapOperationRequest floatingPaymentFrequency(PaymentFrequency floatingPaymentFrequency) {
this.floatingPaymentFrequency = floatingPaymentFrequency;
return this;
}
/**
* Get floatingPaymentFrequency
* @return floatingPaymentFrequency
*/
@NotNull @Valid
@Schema(name = "floatingPaymentFrequency", requiredMode = Schema.RequiredMode.REQUIRED)
public PaymentFrequency getFloatingPaymentFrequency() {
return floatingPaymentFrequency;
}
public void setFloatingPaymentFrequency(PaymentFrequency floatingPaymentFrequency) {
this.floatingPaymentFrequency = floatingPaymentFrequency;
}
public PlainSwapOperationRequest valuationSymbols(List valuationSymbols) {
this.valuationSymbols = valuationSymbols;
return this;
}
public PlainSwapOperationRequest addValuationSymbolsItem(FrontendItemSpec valuationSymbolsItem) {
this.valuationSymbols.add(valuationSymbolsItem);
return this;
}
/**
* Get valuationSymbols
* @return valuationSymbols
*/
@NotNull @Valid
@Schema(name = "valuationSymbols", requiredMode = Schema.RequiredMode.REQUIRED)
public List getValuationSymbols() {
return valuationSymbols;
}
public void setValuationSymbols(List valuationSymbols) {
this.valuationSymbols = valuationSymbols;
}
public PlainSwapOperationRequest currentGenerator(String currentGenerator) {
this.currentGenerator = currentGenerator;
return this;
}
/**
* Get currentGenerator
* @return currentGenerator
*/
@NotNull
@Schema(name = "currentGenerator", requiredMode = Schema.RequiredMode.REQUIRED)
public String getCurrentGenerator() {
return currentGenerator;
}
public void setCurrentGenerator(String currentGenerator) {
this.currentGenerator = currentGenerator;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PlainSwapOperationRequest plainSwapOperationRequest = (PlainSwapOperationRequest) o;
return Objects.equals(this.firstCounterparty, plainSwapOperationRequest.firstCounterparty) &&
Objects.equals(this.secondCounterparty, plainSwapOperationRequest.secondCounterparty) &&
Objects.equals(this.marginBufferAmount, plainSwapOperationRequest.marginBufferAmount) &&
Objects.equals(this.terminationFeeAmount, plainSwapOperationRequest.terminationFeeAmount) &&
Objects.equals(this.notionalAmount, plainSwapOperationRequest.notionalAmount) &&
Objects.equals(this.currency, plainSwapOperationRequest.currency) &&
Objects.equals(this.uniqueTradeIdentifier, plainSwapOperationRequest.uniqueTradeIdentifier) &&
Objects.equals(this.tradeDate, plainSwapOperationRequest.tradeDate) &&
Objects.equals(this.effectiveDate, plainSwapOperationRequest.effectiveDate) &&
Objects.equals(this.terminationDate, plainSwapOperationRequest.terminationDate) &&
Objects.equals(this.fixedPayingParty, plainSwapOperationRequest.fixedPayingParty) &&
Objects.equals(this.fixedRate, plainSwapOperationRequest.fixedRate) &&
Objects.equals(this.fixedDayCountFraction, plainSwapOperationRequest.fixedDayCountFraction) &&
Objects.equals(this.fixedPaymentFrequency, plainSwapOperationRequest.fixedPaymentFrequency) &&
Objects.equals(this.floatingPayingParty, plainSwapOperationRequest.floatingPayingParty) &&
Objects.equals(this.floatingRateIndex, plainSwapOperationRequest.floatingRateIndex) &&
Objects.equals(this.floatingDayCountFraction, plainSwapOperationRequest.floatingDayCountFraction) &&
Objects.equals(this.floatingFixingDayOffset, plainSwapOperationRequest.floatingFixingDayOffset) &&
Objects.equals(this.floatingPaymentFrequency, plainSwapOperationRequest.floatingPaymentFrequency) &&
Objects.equals(this.valuationSymbols, plainSwapOperationRequest.valuationSymbols) &&
Objects.equals(this.currentGenerator, plainSwapOperationRequest.currentGenerator);
}
@Override
public int hashCode() {
return Objects.hash(firstCounterparty, secondCounterparty, marginBufferAmount, terminationFeeAmount, notionalAmount, currency, uniqueTradeIdentifier, tradeDate, effectiveDate, terminationDate, fixedPayingParty, fixedRate, fixedDayCountFraction, fixedPaymentFrequency, floatingPayingParty, floatingRateIndex, floatingDayCountFraction, floatingFixingDayOffset, floatingPaymentFrequency, valuationSymbols, currentGenerator);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PlainSwapOperationRequest {\n");
sb.append(" firstCounterparty: ").append(toIndentedString(firstCounterparty)).append("\n");
sb.append(" secondCounterparty: ").append(toIndentedString(secondCounterparty)).append("\n");
sb.append(" marginBufferAmount: ").append(toIndentedString(marginBufferAmount)).append("\n");
sb.append(" terminationFeeAmount: ").append(toIndentedString(terminationFeeAmount)).append("\n");
sb.append(" notionalAmount: ").append(toIndentedString(notionalAmount)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" uniqueTradeIdentifier: ").append(toIndentedString(uniqueTradeIdentifier)).append("\n");
sb.append(" tradeDate: ").append(toIndentedString(tradeDate)).append("\n");
sb.append(" effectiveDate: ").append(toIndentedString(effectiveDate)).append("\n");
sb.append(" terminationDate: ").append(toIndentedString(terminationDate)).append("\n");
sb.append(" fixedPayingParty: ").append(toIndentedString(fixedPayingParty)).append("\n");
sb.append(" fixedRate: ").append(toIndentedString(fixedRate)).append("\n");
sb.append(" fixedDayCountFraction: ").append(toIndentedString(fixedDayCountFraction)).append("\n");
sb.append(" fixedPaymentFrequency: ").append(toIndentedString(fixedPaymentFrequency)).append("\n");
sb.append(" floatingPayingParty: ").append(toIndentedString(floatingPayingParty)).append("\n");
sb.append(" floatingRateIndex: ").append(toIndentedString(floatingRateIndex)).append("\n");
sb.append(" floatingDayCountFraction: ").append(toIndentedString(floatingDayCountFraction)).append("\n");
sb.append(" floatingFixingDayOffset: ").append(toIndentedString(floatingFixingDayOffset)).append("\n");
sb.append(" floatingPaymentFrequency: ").append(toIndentedString(floatingPaymentFrequency)).append("\n");
sb.append(" valuationSymbols: ").append(toIndentedString(valuationSymbols)).append("\n");
sb.append(" currentGenerator: ").append(toIndentedString(currentGenerator)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy