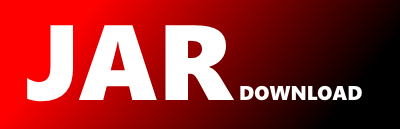
net.finmath.smartcontract.model.RefinitivMarketDataFields Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finmath-smart-derivative-contract Show documentation
Show all versions of finmath-smart-derivative-contract Show documentation
Project to support the implementation a of smart derivative contract.
package net.finmath.smartcontract.model;
import java.net.URI;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import java.time.LocalDate;
import org.springframework.format.annotation.DateTimeFormat;
import org.openapitools.jackson.nullable.JsonNullable;
import java.time.OffsetDateTime;
import jakarta.validation.Valid;
import jakarta.validation.constraints.*;
import io.swagger.v3.oas.annotations.media.Schema;
import java.util.*;
import jakarta.annotation.Generated;
/**
* RefinitivMarketDataFields
*/
@JsonTypeName("RefinitivMarketData_Fields")
@Generated(value = "org.openapitools.codegen.languages.SpringCodegen", date = "2024-12-05T10:54:28.421239+01:00[Europe/Berlin]")
public class RefinitivMarketDataFields {
@JsonProperty("BID")
private Double BID;
@JsonProperty("ASK")
private Double ASK;
@JsonProperty("VALUE_DT1")
@DateTimeFormat(iso = DateTimeFormat.ISO.DATE)
private LocalDate VALUE_DT1;
@JsonProperty("VALUE_TS1")
private String VALUE_TS1;
public RefinitivMarketDataFields BID(Double BID) {
this.BID = BID;
return this;
}
/**
* Get BID
* @return BID
*/
@Schema(name = "BID", requiredMode = Schema.RequiredMode.NOT_REQUIRED)
public Double getBID() {
return BID;
}
public void setBID(Double BID) {
this.BID = BID;
}
public RefinitivMarketDataFields ASK(Double ASK) {
this.ASK = ASK;
return this;
}
/**
* Get ASK
* @return ASK
*/
@Schema(name = "ASK", requiredMode = Schema.RequiredMode.NOT_REQUIRED)
public Double getASK() {
return ASK;
}
public void setASK(Double ASK) {
this.ASK = ASK;
}
public RefinitivMarketDataFields VALUE_DT1(LocalDate VALUE_DT1) {
this.VALUE_DT1 = VALUE_DT1;
return this;
}
/**
* Get VALUE_DT1
* @return VALUE_DT1
*/
@Valid
@Schema(name = "VALUE_DT1", requiredMode = Schema.RequiredMode.NOT_REQUIRED)
public LocalDate getVALUEDT1() {
return VALUE_DT1;
}
public void setVALUEDT1(LocalDate VALUE_DT1) {
this.VALUE_DT1 = VALUE_DT1;
}
public RefinitivMarketDataFields VALUE_TS1(String VALUE_TS1) {
this.VALUE_TS1 = VALUE_TS1;
return this;
}
/**
* Get VALUE_TS1
* @return VALUE_TS1
*/
@Schema(name = "VALUE_TS1", requiredMode = Schema.RequiredMode.NOT_REQUIRED)
public String getVALUETS1() {
return VALUE_TS1;
}
public void setVALUETS1(String VALUE_TS1) {
this.VALUE_TS1 = VALUE_TS1;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
RefinitivMarketDataFields refinitivMarketDataFields = (RefinitivMarketDataFields) o;
return Objects.equals(this.BID, refinitivMarketDataFields.BID) &&
Objects.equals(this.ASK, refinitivMarketDataFields.ASK) &&
Objects.equals(this.VALUE_DT1, refinitivMarketDataFields.VALUE_DT1) &&
Objects.equals(this.VALUE_TS1, refinitivMarketDataFields.VALUE_TS1);
}
@Override
public int hashCode() {
return Objects.hash(BID, ASK, VALUE_DT1, VALUE_TS1);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class RefinitivMarketDataFields {\n");
sb.append(" BID: ").append(toIndentedString(BID)).append("\n");
sb.append(" ASK: ").append(toIndentedString(ASK)).append("\n");
sb.append(" VALUE_DT1: ").append(toIndentedString(VALUE_DT1)).append("\n");
sb.append(" VALUE_TS1: ").append(toIndentedString(VALUE_TS1)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy