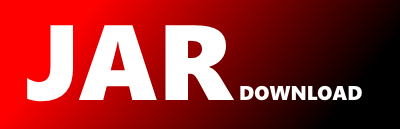
net.finmath.smartcontract.product.IRSwapGenerator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finmath-smart-derivative-contract
Show all versions of finmath-smart-derivative-contract
Project to support the implementation a of smart derivative contract.
Please wait ...