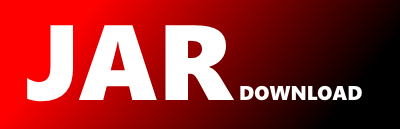
net.finmath.smartcontract.product.xml.CancelableProvision Maven / Gradle / Ivy
Show all versions of finmath-smart-derivative-contract Show documentation
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.0
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.12.05 at 10:53:57 AM CET
//
package net.finmath.smartcontract.product.xml;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.JAXBElement;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlElementRef;
import jakarta.xml.bind.annotation.XmlType;
/**
* A type defining the right of a party to cancel a swap transaction on the
* specified exercise dates. The provision is for 'walkaway' cancellation (i.e. the fair value of the swap
* is not paid). A fee payable on exercise can be specified.
*
*
* Java class for CancelableProvision complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="CancelableProvision">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <group ref="{http://www.fpml.org/FpML-5/confirmation}BuyerSeller.model"/>
* <element ref="{http://www.fpml.org/FpML-5/confirmation}exercise"/>
* <element name="exerciseNotice" type="{http://www.fpml.org/FpML-5/confirmation}ExerciseNotice" minOccurs="0"/>
* <element name="followUpConfirmation" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* <element name="cancelableProvisionAdjustedDates" type="{http://www.fpml.org/FpML-5/confirmation}CancelableProvisionAdjustedDates" minOccurs="0"/>
* <element name="finalCalculationPeriodDateAdjustment" type="{http://www.fpml.org/FpML-5/confirmation}FinalCalculationPeriodDateAdjustment" maxOccurs="unbounded" minOccurs="0"/>
* <element name="initialFee" type="{http://www.fpml.org/FpML-5/confirmation}SimplePayment" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "CancelableProvision", propOrder = {
"buyerPartyReference",
"buyerAccountReference",
"sellerPartyReference",
"sellerAccountReference",
"exercise",
"exerciseNotice",
"followUpConfirmation",
"cancelableProvisionAdjustedDates",
"finalCalculationPeriodDateAdjustment",
"initialFee"
})
public class CancelableProvision {
@XmlElement(required = true)
protected PartyReference buyerPartyReference;
protected AccountReference buyerAccountReference;
@XmlElement(required = true)
protected PartyReference sellerPartyReference;
protected AccountReference sellerAccountReference;
@XmlElementRef(name = "exercise", namespace = "http://www.fpml.org/FpML-5/confirmation", type = JAXBElement.class)
protected JAXBElement extends Exercise> exercise;
protected ExerciseNotice exerciseNotice;
protected boolean followUpConfirmation;
protected CancelableProvisionAdjustedDates cancelableProvisionAdjustedDates;
protected List finalCalculationPeriodDateAdjustment;
protected SimplePayment initialFee;
/**
* Gets the value of the buyerPartyReference property.
*
* @return
* possible object is
* {@link PartyReference }
*
*/
public PartyReference getBuyerPartyReference() {
return buyerPartyReference;
}
/**
* Sets the value of the buyerPartyReference property.
*
* @param value
* allowed object is
* {@link PartyReference }
*
*/
public void setBuyerPartyReference(PartyReference value) {
this.buyerPartyReference = value;
}
/**
* Gets the value of the buyerAccountReference property.
*
* @return
* possible object is
* {@link AccountReference }
*
*/
public AccountReference getBuyerAccountReference() {
return buyerAccountReference;
}
/**
* Sets the value of the buyerAccountReference property.
*
* @param value
* allowed object is
* {@link AccountReference }
*
*/
public void setBuyerAccountReference(AccountReference value) {
this.buyerAccountReference = value;
}
/**
* Gets the value of the sellerPartyReference property.
*
* @return
* possible object is
* {@link PartyReference }
*
*/
public PartyReference getSellerPartyReference() {
return sellerPartyReference;
}
/**
* Sets the value of the sellerPartyReference property.
*
* @param value
* allowed object is
* {@link PartyReference }
*
*/
public void setSellerPartyReference(PartyReference value) {
this.sellerPartyReference = value;
}
/**
* Gets the value of the sellerAccountReference property.
*
* @return
* possible object is
* {@link AccountReference }
*
*/
public AccountReference getSellerAccountReference() {
return sellerAccountReference;
}
/**
* Sets the value of the sellerAccountReference property.
*
* @param value
* allowed object is
* {@link AccountReference }
*
*/
public void setSellerAccountReference(AccountReference value) {
this.sellerAccountReference = value;
}
/**
* Gets the value of the exercise property.
*
* @return
* possible object is
* {@link JAXBElement }{@code <}{@link BermudaExercise }{@code >}
* {@link JAXBElement }{@code <}{@link EuropeanExercise }{@code >}
* {@link JAXBElement }{@code <}{@link AmericanExercise }{@code >}
* {@link JAXBElement }{@code <}{@link Exercise }{@code >}
*
*/
public JAXBElement extends Exercise> getExercise() {
return exercise;
}
/**
* Sets the value of the exercise property.
*
* @param value
* allowed object is
* {@link JAXBElement }{@code <}{@link BermudaExercise }{@code >}
* {@link JAXBElement }{@code <}{@link EuropeanExercise }{@code >}
* {@link JAXBElement }{@code <}{@link AmericanExercise }{@code >}
* {@link JAXBElement }{@code <}{@link Exercise }{@code >}
*
*/
public void setExercise(JAXBElement extends Exercise> value) {
this.exercise = value;
}
/**
* Gets the value of the exerciseNotice property.
*
* @return
* possible object is
* {@link ExerciseNotice }
*
*/
public ExerciseNotice getExerciseNotice() {
return exerciseNotice;
}
/**
* Sets the value of the exerciseNotice property.
*
* @param value
* allowed object is
* {@link ExerciseNotice }
*
*/
public void setExerciseNotice(ExerciseNotice value) {
this.exerciseNotice = value;
}
/**
* Gets the value of the followUpConfirmation property.
*
*/
public boolean isFollowUpConfirmation() {
return followUpConfirmation;
}
/**
* Sets the value of the followUpConfirmation property.
*
*/
public void setFollowUpConfirmation(boolean value) {
this.followUpConfirmation = value;
}
/**
* Gets the value of the cancelableProvisionAdjustedDates property.
*
* @return
* possible object is
* {@link CancelableProvisionAdjustedDates }
*
*/
public CancelableProvisionAdjustedDates getCancelableProvisionAdjustedDates() {
return cancelableProvisionAdjustedDates;
}
/**
* Sets the value of the cancelableProvisionAdjustedDates property.
*
* @param value
* allowed object is
* {@link CancelableProvisionAdjustedDates }
*
*/
public void setCancelableProvisionAdjustedDates(CancelableProvisionAdjustedDates value) {
this.cancelableProvisionAdjustedDates = value;
}
/**
* Gets the value of the finalCalculationPeriodDateAdjustment property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the finalCalculationPeriodDateAdjustment property.
*
*
* For example, to add a new item, do as follows:
*
* getFinalCalculationPeriodDateAdjustment().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FinalCalculationPeriodDateAdjustment }
*
*
*/
public List getFinalCalculationPeriodDateAdjustment() {
if (finalCalculationPeriodDateAdjustment == null) {
finalCalculationPeriodDateAdjustment = new ArrayList();
}
return this.finalCalculationPeriodDateAdjustment;
}
/**
* Gets the value of the initialFee property.
*
* @return
* possible object is
* {@link SimplePayment }
*
*/
public SimplePayment getInitialFee() {
return initialFee;
}
/**
* Sets the value of the initialFee property.
*
* @param value
* allowed object is
* {@link SimplePayment }
*
*/
public void setInitialFee(SimplePayment value) {
this.initialFee = value;
}
}