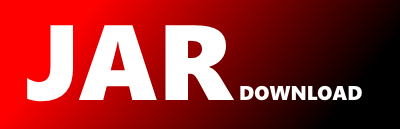
net.finmath.smartcontract.product.xml.CommoditySwap Maven / Gradle / Ivy
Show all versions of finmath-smart-derivative-contract Show documentation
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.0
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.12.05 at 10:53:57 AM CET
//
package net.finmath.smartcontract.product.xml;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.JAXBElement;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlElementRef;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
/**
* The commodity swap product model is designed to support fixed-float swaps,
* float-float swaps, fixed vs. physical swaps, float vs. physical swaps as well as, weather specific
* swaps. Its design is fully compatible with other FpML products and the product reuses standard common
* types.
*
*
* Java class for CommoditySwap complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="CommoditySwap">
* <complexContent>
* <extension base="{http://www.fpml.org/FpML-5/confirmation}Product">
* <sequence>
* <group ref="{http://www.fpml.org/FpML-5/confirmation}CommoditySwapDetails.model"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "CommoditySwap", propOrder = {
"effectiveDate",
"terminationDate",
"settlementCurrency",
"commoditySwapLeg",
"weatherLeg",
"commonPricing",
"marketDisruption",
"settlementDisruption",
"rounding"
})
public class CommoditySwap
extends Product
{
@XmlElement(required = true)
protected AdjustableOrRelativeDate effectiveDate;
@XmlElement(required = true)
protected AdjustableOrRelativeDate terminationDate;
@XmlElement(required = true)
protected IdentifiedCurrency settlementCurrency;
@XmlElementRef(name = "commoditySwapLeg", namespace = "http://www.fpml.org/FpML-5/confirmation", type = JAXBElement.class, required = false)
protected List> commoditySwapLeg;
protected List weatherLeg;
protected Boolean commonPricing;
protected CommodityMarketDisruption marketDisruption;
@XmlSchemaType(name = "token")
protected CommodityBullionSettlementDisruptionEnum settlementDisruption;
protected Rounding rounding;
/**
* Gets the value of the effectiveDate property.
*
* @return
* possible object is
* {@link AdjustableOrRelativeDate }
*
*/
public AdjustableOrRelativeDate getEffectiveDate() {
return effectiveDate;
}
/**
* Sets the value of the effectiveDate property.
*
* @param value
* allowed object is
* {@link AdjustableOrRelativeDate }
*
*/
public void setEffectiveDate(AdjustableOrRelativeDate value) {
this.effectiveDate = value;
}
/**
* Gets the value of the terminationDate property.
*
* @return
* possible object is
* {@link AdjustableOrRelativeDate }
*
*/
public AdjustableOrRelativeDate getTerminationDate() {
return terminationDate;
}
/**
* Sets the value of the terminationDate property.
*
* @param value
* allowed object is
* {@link AdjustableOrRelativeDate }
*
*/
public void setTerminationDate(AdjustableOrRelativeDate value) {
this.terminationDate = value;
}
/**
* Gets the value of the settlementCurrency property.
*
* @return
* possible object is
* {@link IdentifiedCurrency }
*
*/
public IdentifiedCurrency getSettlementCurrency() {
return settlementCurrency;
}
/**
* Sets the value of the settlementCurrency property.
*
* @param value
* allowed object is
* {@link IdentifiedCurrency }
*
*/
public void setSettlementCurrency(IdentifiedCurrency value) {
this.settlementCurrency = value;
}
/**
* Gets the value of the commoditySwapLeg property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the commoditySwapLeg property.
*
*
* For example, to add a new item, do as follows:
*
* getCommoditySwapLeg().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link JAXBElement }{@code <}{@link OilPhysicalLeg }{@code >}
* {@link JAXBElement }{@code <}{@link EnvironmentalPhysicalLeg }{@code >}
* {@link JAXBElement }{@code <}{@link ElectricityPhysicalLeg }{@code >}
* {@link JAXBElement }{@code <}{@link CoalPhysicalLeg }{@code >}
* {@link JAXBElement }{@code <}{@link FloatingPriceLeg }{@code >}
* {@link JAXBElement }{@code <}{@link GasPhysicalLeg }{@code >}
* {@link JAXBElement }{@code <}{@link FixedPriceLeg }{@code >}
* {@link JAXBElement }{@code <}{@link CommoditySwapLeg }{@code >}
*
*
*/
public List> getCommoditySwapLeg() {
if (commoditySwapLeg == null) {
commoditySwapLeg = new ArrayList>();
}
return this.commoditySwapLeg;
}
/**
* Gets the value of the weatherLeg property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the weatherLeg property.
*
*
* For example, to add a new item, do as follows:
*
* getWeatherLeg().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link WeatherLeg }
*
*
*/
public List getWeatherLeg() {
if (weatherLeg == null) {
weatherLeg = new ArrayList();
}
return this.weatherLeg;
}
/**
* Gets the value of the commonPricing property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isCommonPricing() {
return commonPricing;
}
/**
* Sets the value of the commonPricing property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setCommonPricing(Boolean value) {
this.commonPricing = value;
}
/**
* Gets the value of the marketDisruption property.
*
* @return
* possible object is
* {@link CommodityMarketDisruption }
*
*/
public CommodityMarketDisruption getMarketDisruption() {
return marketDisruption;
}
/**
* Sets the value of the marketDisruption property.
*
* @param value
* allowed object is
* {@link CommodityMarketDisruption }
*
*/
public void setMarketDisruption(CommodityMarketDisruption value) {
this.marketDisruption = value;
}
/**
* Gets the value of the settlementDisruption property.
*
* @return
* possible object is
* {@link CommodityBullionSettlementDisruptionEnum }
*
*/
public CommodityBullionSettlementDisruptionEnum getSettlementDisruption() {
return settlementDisruption;
}
/**
* Sets the value of the settlementDisruption property.
*
* @param value
* allowed object is
* {@link CommodityBullionSettlementDisruptionEnum }
*
*/
public void setSettlementDisruption(CommodityBullionSettlementDisruptionEnum value) {
this.settlementDisruption = value;
}
/**
* Gets the value of the rounding property.
*
* @return
* possible object is
* {@link Rounding }
*
*/
public Rounding getRounding() {
return rounding;
}
/**
* Sets the value of the rounding property.
*
* @param value
* allowed object is
* {@link Rounding }
*
*/
public void setRounding(Rounding value) {
this.rounding = value;
}
}