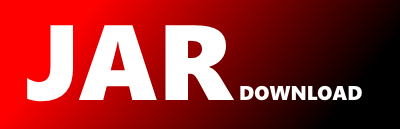
net.finmath.smartcontract.product.xml.EnvironmentalPhysicalLeg Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finmath-smart-derivative-contract Show documentation
Show all versions of finmath-smart-derivative-contract Show documentation
Project to support the implementation a of smart derivative contract.
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.0
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.12.05 at 10:53:57 AM CET
//
package net.finmath.smartcontract.product.xml;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
/**
* Java class for EnvironmentalPhysicalLeg complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="EnvironmentalPhysicalLeg">
* <complexContent>
* <extension base="{http://www.fpml.org/FpML-5/confirmation}PhysicalSwapLeg">
* <sequence>
* <element name="numberOfAllowances" type="{http://www.fpml.org/FpML-5/confirmation}UnitQuantity"/>
* <element name="environmental" type="{http://www.fpml.org/FpML-5/confirmation}EnvironmentalProduct"/>
* <element name="abandonmentOfScheme" type="{http://www.fpml.org/FpML-5/confirmation}EnvironmentalAbandonmentOfSchemeEnum" minOccurs="0"/>
* <element name="deliveryDate" type="{http://www.fpml.org/FpML-5/confirmation}AdjustableOrRelativeDate"/>
* <sequence>
* <element name="paymentDate" type="{http://www.fpml.org/FpML-5/confirmation}DateOffset"/>
* <group ref="{http://www.fpml.org/FpML-5/confirmation}BusinessCentersOrReference.model" minOccurs="0"/>
* </sequence>
* <element name="failureToDeliverApplicable" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="eEPParameters" type="{http://www.fpml.org/FpML-5/confirmation}EEPParameters" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "EnvironmentalPhysicalLeg", propOrder = {
"numberOfAllowances",
"environmental",
"abandonmentOfScheme",
"deliveryDate",
"paymentDate",
"businessCentersReference",
"businessCenters",
"failureToDeliverApplicable",
"eepParameters"
})
public class EnvironmentalPhysicalLeg
extends PhysicalSwapLeg
{
@XmlElement(required = true)
protected UnitQuantity numberOfAllowances;
@XmlElement(required = true)
protected EnvironmentalProduct environmental;
@XmlSchemaType(name = "token")
protected EnvironmentalAbandonmentOfSchemeEnum abandonmentOfScheme;
@XmlElement(required = true)
protected AdjustableOrRelativeDate deliveryDate;
@XmlElement(required = true)
protected DateOffset paymentDate;
protected BusinessCentersReference businessCentersReference;
protected BusinessCenters businessCenters;
protected Boolean failureToDeliverApplicable;
@XmlElement(name = "eEPParameters")
protected EEPParameters eepParameters;
/**
* Gets the value of the numberOfAllowances property.
*
* @return
* possible object is
* {@link UnitQuantity }
*
*/
public UnitQuantity getNumberOfAllowances() {
return numberOfAllowances;
}
/**
* Sets the value of the numberOfAllowances property.
*
* @param value
* allowed object is
* {@link UnitQuantity }
*
*/
public void setNumberOfAllowances(UnitQuantity value) {
this.numberOfAllowances = value;
}
/**
* Gets the value of the environmental property.
*
* @return
* possible object is
* {@link EnvironmentalProduct }
*
*/
public EnvironmentalProduct getEnvironmental() {
return environmental;
}
/**
* Sets the value of the environmental property.
*
* @param value
* allowed object is
* {@link EnvironmentalProduct }
*
*/
public void setEnvironmental(EnvironmentalProduct value) {
this.environmental = value;
}
/**
* Gets the value of the abandonmentOfScheme property.
*
* @return
* possible object is
* {@link EnvironmentalAbandonmentOfSchemeEnum }
*
*/
public EnvironmentalAbandonmentOfSchemeEnum getAbandonmentOfScheme() {
return abandonmentOfScheme;
}
/**
* Sets the value of the abandonmentOfScheme property.
*
* @param value
* allowed object is
* {@link EnvironmentalAbandonmentOfSchemeEnum }
*
*/
public void setAbandonmentOfScheme(EnvironmentalAbandonmentOfSchemeEnum value) {
this.abandonmentOfScheme = value;
}
/**
* Gets the value of the deliveryDate property.
*
* @return
* possible object is
* {@link AdjustableOrRelativeDate }
*
*/
public AdjustableOrRelativeDate getDeliveryDate() {
return deliveryDate;
}
/**
* Sets the value of the deliveryDate property.
*
* @param value
* allowed object is
* {@link AdjustableOrRelativeDate }
*
*/
public void setDeliveryDate(AdjustableOrRelativeDate value) {
this.deliveryDate = value;
}
/**
* Gets the value of the paymentDate property.
*
* @return
* possible object is
* {@link DateOffset }
*
*/
public DateOffset getPaymentDate() {
return paymentDate;
}
/**
* Sets the value of the paymentDate property.
*
* @param value
* allowed object is
* {@link DateOffset }
*
*/
public void setPaymentDate(DateOffset value) {
this.paymentDate = value;
}
/**
* Gets the value of the businessCentersReference property.
*
* @return
* possible object is
* {@link BusinessCentersReference }
*
*/
public BusinessCentersReference getBusinessCentersReference() {
return businessCentersReference;
}
/**
* Sets the value of the businessCentersReference property.
*
* @param value
* allowed object is
* {@link BusinessCentersReference }
*
*/
public void setBusinessCentersReference(BusinessCentersReference value) {
this.businessCentersReference = value;
}
/**
* Gets the value of the businessCenters property.
*
* @return
* possible object is
* {@link BusinessCenters }
*
*/
public BusinessCenters getBusinessCenters() {
return businessCenters;
}
/**
* Sets the value of the businessCenters property.
*
* @param value
* allowed object is
* {@link BusinessCenters }
*
*/
public void setBusinessCenters(BusinessCenters value) {
this.businessCenters = value;
}
/**
* Gets the value of the failureToDeliverApplicable property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isFailureToDeliverApplicable() {
return failureToDeliverApplicable;
}
/**
* Sets the value of the failureToDeliverApplicable property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setFailureToDeliverApplicable(Boolean value) {
this.failureToDeliverApplicable = value;
}
/**
* Gets the value of the eepParameters property.
*
* @return
* possible object is
* {@link EEPParameters }
*
*/
public EEPParameters getEEPParameters() {
return eepParameters;
}
/**
* Sets the value of the eepParameters property.
*
* @param value
* allowed object is
* {@link EEPParameters }
*
*/
public void setEEPParameters(EEPParameters value) {
this.eepParameters = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy