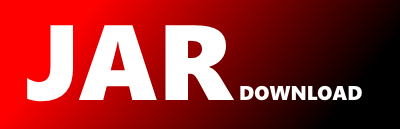
net.finmath.smartcontract.product.xml.EquityDerivativeBase Maven / Gradle / Ivy
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.0
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.12.05 at 10:53:57 AM CET
//
package net.finmath.smartcontract.product.xml;
import javax.xml.datatype.XMLGregorianCalendar;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlSeeAlso;
import jakarta.xml.bind.annotation.XmlType;
/**
* A type for defining the common features of equity derivatives.
*
*
* Java class for EquityDerivativeBase complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="EquityDerivativeBase">
* <complexContent>
* <extension base="{http://www.fpml.org/FpML-5/confirmation}Product">
* <sequence>
* <group ref="{http://www.fpml.org/FpML-5/confirmation}BuyerSeller.model"/>
* <element name="optionType" type="{http://www.fpml.org/FpML-5/confirmation}EquityOptionTypeEnum"/>
* <element name="equityEffectiveDate" type="{http://www.w3.org/2001/XMLSchema}date" minOccurs="0"/>
* <element name="underlyer" type="{http://www.fpml.org/FpML-5/confirmation}Underlyer"/>
* <element name="notional" type="{http://www.fpml.org/FpML-5/confirmation}NonNegativeMoney" minOccurs="0"/>
* <element name="equityExercise" type="{http://www.fpml.org/FpML-5/confirmation}EquityExerciseValuationSettlement"/>
* <group ref="{http://www.fpml.org/FpML-5/confirmation}Feature.model" minOccurs="0"/>
* <element name="strategyFeature" type="{http://www.fpml.org/FpML-5/confirmation}StrategyFeature" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "EquityDerivativeBase", propOrder = {
"buyerPartyReference",
"buyerAccountReference",
"sellerPartyReference",
"sellerAccountReference",
"optionType",
"equityEffectiveDate",
"underlyer",
"notional",
"equityExercise",
"feature",
"fxFeature",
"strategyFeature"
})
@XmlSeeAlso({
EquityDerivativeLongFormBase.class,
EquityDerivativeShortFormBase.class
})
public abstract class EquityDerivativeBase
extends Product
{
@XmlElement(required = true)
protected PartyReference buyerPartyReference;
protected AccountReference buyerAccountReference;
@XmlElement(required = true)
protected PartyReference sellerPartyReference;
protected AccountReference sellerAccountReference;
@XmlElement(required = true)
protected String optionType;
@XmlSchemaType(name = "date")
protected XMLGregorianCalendar equityEffectiveDate;
@XmlElement(required = true)
protected Underlyer underlyer;
protected NonNegativeMoney notional;
@XmlElement(required = true)
protected EquityExerciseValuationSettlement equityExercise;
protected OptionFeatures feature;
protected FxFeature fxFeature;
protected StrategyFeature strategyFeature;
/**
* Gets the value of the buyerPartyReference property.
*
* @return
* possible object is
* {@link PartyReference }
*
*/
public PartyReference getBuyerPartyReference() {
return buyerPartyReference;
}
/**
* Sets the value of the buyerPartyReference property.
*
* @param value
* allowed object is
* {@link PartyReference }
*
*/
public void setBuyerPartyReference(PartyReference value) {
this.buyerPartyReference = value;
}
/**
* Gets the value of the buyerAccountReference property.
*
* @return
* possible object is
* {@link AccountReference }
*
*/
public AccountReference getBuyerAccountReference() {
return buyerAccountReference;
}
/**
* Sets the value of the buyerAccountReference property.
*
* @param value
* allowed object is
* {@link AccountReference }
*
*/
public void setBuyerAccountReference(AccountReference value) {
this.buyerAccountReference = value;
}
/**
* Gets the value of the sellerPartyReference property.
*
* @return
* possible object is
* {@link PartyReference }
*
*/
public PartyReference getSellerPartyReference() {
return sellerPartyReference;
}
/**
* Sets the value of the sellerPartyReference property.
*
* @param value
* allowed object is
* {@link PartyReference }
*
*/
public void setSellerPartyReference(PartyReference value) {
this.sellerPartyReference = value;
}
/**
* Gets the value of the sellerAccountReference property.
*
* @return
* possible object is
* {@link AccountReference }
*
*/
public AccountReference getSellerAccountReference() {
return sellerAccountReference;
}
/**
* Sets the value of the sellerAccountReference property.
*
* @param value
* allowed object is
* {@link AccountReference }
*
*/
public void setSellerAccountReference(AccountReference value) {
this.sellerAccountReference = value;
}
/**
* Gets the value of the optionType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOptionType() {
return optionType;
}
/**
* Sets the value of the optionType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOptionType(String value) {
this.optionType = value;
}
/**
* Gets the value of the equityEffectiveDate property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getEquityEffectiveDate() {
return equityEffectiveDate;
}
/**
* Sets the value of the equityEffectiveDate property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setEquityEffectiveDate(XMLGregorianCalendar value) {
this.equityEffectiveDate = value;
}
/**
* Gets the value of the underlyer property.
*
* @return
* possible object is
* {@link Underlyer }
*
*/
public Underlyer getUnderlyer() {
return underlyer;
}
/**
* Sets the value of the underlyer property.
*
* @param value
* allowed object is
* {@link Underlyer }
*
*/
public void setUnderlyer(Underlyer value) {
this.underlyer = value;
}
/**
* Gets the value of the notional property.
*
* @return
* possible object is
* {@link NonNegativeMoney }
*
*/
public NonNegativeMoney getNotional() {
return notional;
}
/**
* Sets the value of the notional property.
*
* @param value
* allowed object is
* {@link NonNegativeMoney }
*
*/
public void setNotional(NonNegativeMoney value) {
this.notional = value;
}
/**
* Gets the value of the equityExercise property.
*
* @return
* possible object is
* {@link EquityExerciseValuationSettlement }
*
*/
public EquityExerciseValuationSettlement getEquityExercise() {
return equityExercise;
}
/**
* Sets the value of the equityExercise property.
*
* @param value
* allowed object is
* {@link EquityExerciseValuationSettlement }
*
*/
public void setEquityExercise(EquityExerciseValuationSettlement value) {
this.equityExercise = value;
}
/**
* Gets the value of the feature property.
*
* @return
* possible object is
* {@link OptionFeatures }
*
*/
public OptionFeatures getFeature() {
return feature;
}
/**
* Sets the value of the feature property.
*
* @param value
* allowed object is
* {@link OptionFeatures }
*
*/
public void setFeature(OptionFeatures value) {
this.feature = value;
}
/**
* Gets the value of the fxFeature property.
*
* @return
* possible object is
* {@link FxFeature }
*
*/
public FxFeature getFxFeature() {
return fxFeature;
}
/**
* Sets the value of the fxFeature property.
*
* @param value
* allowed object is
* {@link FxFeature }
*
*/
public void setFxFeature(FxFeature value) {
this.fxFeature = value;
}
/**
* Gets the value of the strategyFeature property.
*
* @return
* possible object is
* {@link StrategyFeature }
*
*/
public StrategyFeature getStrategyFeature() {
return strategyFeature;
}
/**
* Sets the value of the strategyFeature property.
*
* @param value
* allowed object is
* {@link StrategyFeature }
*
*/
public void setStrategyFeature(StrategyFeature value) {
this.strategyFeature = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy