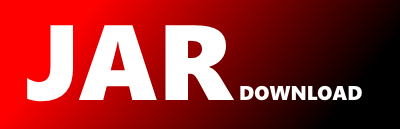
net.finmath.smartcontract.product.xml.ExtendibleProvision Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finmath-smart-derivative-contract Show documentation
Show all versions of finmath-smart-derivative-contract Show documentation
Project to support the implementation a of smart derivative contract.
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.0
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.12.05 at 10:53:57 AM CET
//
package net.finmath.smartcontract.product.xml;
import jakarta.xml.bind.JAXBElement;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlElementRef;
import jakarta.xml.bind.annotation.XmlType;
/**
* A type defining an option to extend an existing swap transaction on the
* specified exercise dates for a term ending on the specified new termination date.
*
*
* Java class for ExtendibleProvision complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="ExtendibleProvision">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <group ref="{http://www.fpml.org/FpML-5/confirmation}BuyerSeller.model"/>
* <element ref="{http://www.fpml.org/FpML-5/confirmation}exercise"/>
* <element name="exerciseNotice" type="{http://www.fpml.org/FpML-5/confirmation}ExerciseNotice" minOccurs="0"/>
* <element name="followUpConfirmation" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* <element name="extendibleProvisionAdjustedDates" type="{http://www.fpml.org/FpML-5/confirmation}ExtendibleProvisionAdjustedDates" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "ExtendibleProvision", propOrder = {
"buyerPartyReference",
"buyerAccountReference",
"sellerPartyReference",
"sellerAccountReference",
"exercise",
"exerciseNotice",
"followUpConfirmation",
"extendibleProvisionAdjustedDates"
})
public class ExtendibleProvision {
@XmlElement(required = true)
protected PartyReference buyerPartyReference;
protected AccountReference buyerAccountReference;
@XmlElement(required = true)
protected PartyReference sellerPartyReference;
protected AccountReference sellerAccountReference;
@XmlElementRef(name = "exercise", namespace = "http://www.fpml.org/FpML-5/confirmation", type = JAXBElement.class)
protected JAXBElement extends Exercise> exercise;
protected ExerciseNotice exerciseNotice;
protected boolean followUpConfirmation;
protected ExtendibleProvisionAdjustedDates extendibleProvisionAdjustedDates;
/**
* Gets the value of the buyerPartyReference property.
*
* @return
* possible object is
* {@link PartyReference }
*
*/
public PartyReference getBuyerPartyReference() {
return buyerPartyReference;
}
/**
* Sets the value of the buyerPartyReference property.
*
* @param value
* allowed object is
* {@link PartyReference }
*
*/
public void setBuyerPartyReference(PartyReference value) {
this.buyerPartyReference = value;
}
/**
* Gets the value of the buyerAccountReference property.
*
* @return
* possible object is
* {@link AccountReference }
*
*/
public AccountReference getBuyerAccountReference() {
return buyerAccountReference;
}
/**
* Sets the value of the buyerAccountReference property.
*
* @param value
* allowed object is
* {@link AccountReference }
*
*/
public void setBuyerAccountReference(AccountReference value) {
this.buyerAccountReference = value;
}
/**
* Gets the value of the sellerPartyReference property.
*
* @return
* possible object is
* {@link PartyReference }
*
*/
public PartyReference getSellerPartyReference() {
return sellerPartyReference;
}
/**
* Sets the value of the sellerPartyReference property.
*
* @param value
* allowed object is
* {@link PartyReference }
*
*/
public void setSellerPartyReference(PartyReference value) {
this.sellerPartyReference = value;
}
/**
* Gets the value of the sellerAccountReference property.
*
* @return
* possible object is
* {@link AccountReference }
*
*/
public AccountReference getSellerAccountReference() {
return sellerAccountReference;
}
/**
* Sets the value of the sellerAccountReference property.
*
* @param value
* allowed object is
* {@link AccountReference }
*
*/
public void setSellerAccountReference(AccountReference value) {
this.sellerAccountReference = value;
}
/**
* Gets the value of the exercise property.
*
* @return
* possible object is
* {@link JAXBElement }{@code <}{@link BermudaExercise }{@code >}
* {@link JAXBElement }{@code <}{@link EuropeanExercise }{@code >}
* {@link JAXBElement }{@code <}{@link AmericanExercise }{@code >}
* {@link JAXBElement }{@code <}{@link Exercise }{@code >}
*
*/
public JAXBElement extends Exercise> getExercise() {
return exercise;
}
/**
* Sets the value of the exercise property.
*
* @param value
* allowed object is
* {@link JAXBElement }{@code <}{@link BermudaExercise }{@code >}
* {@link JAXBElement }{@code <}{@link EuropeanExercise }{@code >}
* {@link JAXBElement }{@code <}{@link AmericanExercise }{@code >}
* {@link JAXBElement }{@code <}{@link Exercise }{@code >}
*
*/
public void setExercise(JAXBElement extends Exercise> value) {
this.exercise = value;
}
/**
* Gets the value of the exerciseNotice property.
*
* @return
* possible object is
* {@link ExerciseNotice }
*
*/
public ExerciseNotice getExerciseNotice() {
return exerciseNotice;
}
/**
* Sets the value of the exerciseNotice property.
*
* @param value
* allowed object is
* {@link ExerciseNotice }
*
*/
public void setExerciseNotice(ExerciseNotice value) {
this.exerciseNotice = value;
}
/**
* Gets the value of the followUpConfirmation property.
*
*/
public boolean isFollowUpConfirmation() {
return followUpConfirmation;
}
/**
* Sets the value of the followUpConfirmation property.
*
*/
public void setFollowUpConfirmation(boolean value) {
this.followUpConfirmation = value;
}
/**
* Gets the value of the extendibleProvisionAdjustedDates property.
*
* @return
* possible object is
* {@link ExtendibleProvisionAdjustedDates }
*
*/
public ExtendibleProvisionAdjustedDates getExtendibleProvisionAdjustedDates() {
return extendibleProvisionAdjustedDates;
}
/**
* Sets the value of the extendibleProvisionAdjustedDates property.
*
* @param value
* allowed object is
* {@link ExtendibleProvisionAdjustedDates }
*
*/
public void setExtendibleProvisionAdjustedDates(ExtendibleProvisionAdjustedDates value) {
this.extendibleProvisionAdjustedDates = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy